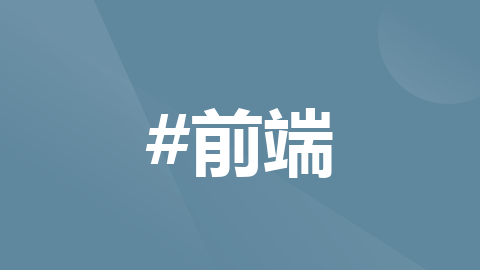
vue3(上拉/触底)加载更多
获取可视窗口高度,滚动高度,滚动条高度。
·
vue3(上拉/触底)加载更多
核心原理
-
监听页面的滚动事件
window.addEventListener('scroll', function)
-
获取可视窗口高度,滚动高度,滚动条高度
document.documentElement.clientHeight; // 获取可视高度 document.documentElement.scrollTop // 获取滚动高度 document.documentElement.scrollHeight // 获取滚动条高度
页面代码
<template>
<div class="container">
<ul>
<li class="item" v-for="(item, index) in dataList" :key="index">{{ item }}</li>
</ul>
</div>
</template>
<script setup>
import { reactive } from 'vue';
const dataList = reactive([1, 2, 3, 4])
// 节流方法
const throttle = (func, delay) => {
let timer = null;
return function () {
if (!timer) {
timer = setTimeout(() => {
func.apply(this, arguments);
timer = null
}, delay)
}
}
}
// 触底函数
const onReachBottom = () => {
window.addEventListener('scroll', throttle(onBottom, 200))
}
// 触底相应函数
const onBottom = () => {
// 获取可视高度
let clientHeight = document.documentElement.clientHeight;
// 获取滚动高度
let scrollTop = document.documentElement.scrollTop
// 滚动条高度
let scrollHeight = document.documentElement.scrollHeight
if (clientHeight + scrollTop > scrollHeight) {
console.log('触底了')
dataList.push(dataList.length + 1)
}
}
onReachBottom()
</script>
<style lang="scss" scoped>
.item {
margin-top: 20px;
width: 200px;
height: 200px;
font-size: 30px;
text-align: center;
line-height: 200px;
background-color: hotpink;
}
</style>
更多推荐
所有评论(0)