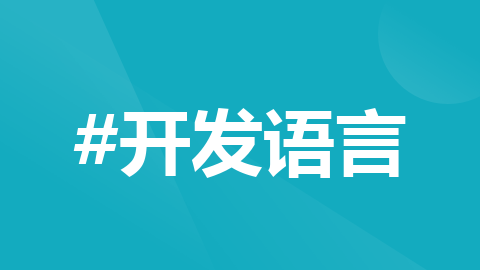
c++获取当前目录
c++获取当前目录,Windows和Linux

一键AI生成摘要,助你高效阅读
问答
·
1.说明
- 如果编译器支持C++17,则建议使用std::filesystem::current_path
- 如果只在windows平台使用,可使用_getcwd
- 如果只在linux平台使用,可使用getcwd
- 如果代码要跨平台使用,可以使用通过编译统一的版本
2. C++17 std::filesystem::current_path
#include <iostream>
#include <filesystem>
namespace fs = std::filesystem;
int main()
{
std::cout << "Current working directory: " << fs::current_path() << '\n';
}
Current working directory: "D:/local/ConsoleApplication1"
std::filesystem::current_path 还可以改变当前目录
https://en.cppreference.com/w/cpp/filesystem/current_path
3.windows平台 _getcwd
#include <direct.h>
std::string current_working_directory()
{
char buff[250];
_getcwd(buff, 250);
std::string current_working_directory(buff);
return current_working_directory;
}
int main()
{
std::cout << "Current working directory: " << current_working_directory() << endl;
}
直接使用getcwd会有告警
https://docs.microsoft.com/en-us/cpp/c-runtime-library/reference/getcwd-wgetcwd?view=msvc-160
4.linux平台 getcwd
#include <unistd.h>
int main()
{
char buff[250];
getcwd(buff, 250);
string current_working_directory(buff);
cout << "Current working directory: " << current_working_directory<< endl;
return 0;
}
或者使用get_current_dir_name
#include <unistd.h>
int main()
{
char *cwd = get_current_dir_name();
cout << "Current working directory: " << cwd << endl;
free(cwd);
return 0;
}
https://man7.org/linux/man-pages/man3/getcwd.3.html
https://linux.die.net/man/3/get_current_dir_name
5. 通过编译宏统一两者版本
#if defined(_MSC_VER)
#include <direct.h>
#define GetCurrentDir _getcwd
#elif defined(__unix__)
#include <unistd.h>
#define GetCurrentDir getcwd
#else
#endif
std::string get_current_directory()
{
char buff[250];
GetCurrentDir(buff, 250);
string current_working_directory(buff);
return current_working_directory;
}
int main(int argc, char* argv[])
{
std::cout << "Current working directory: " << get_current_directory() << endl;
return 0;
}
参考: https://www.tutorialspoint.com/find-out-the-current-working-directory-in-c-cplusplus
更多推荐
所有评论(0)