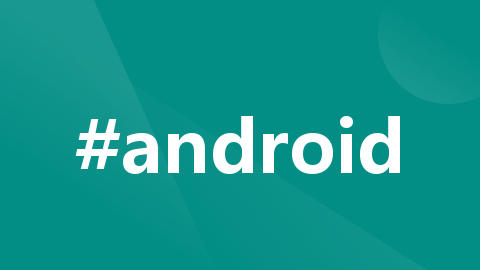
android 获得wifi列表并连接wifi
"设备名(SSID) ->${it.SSID}\t""信号强度 ->${it.level}\t""wifi打开失败,请联系管理员"4.wifiDialog布局。"附件没有可用的wifi""wifi连接中...""wifi连接成功"
·
1.引包
-
implementation
'com.thanosfisherman.wifiutils:wifiutils:1.6.4'
-
implementation
'com.thanosfisherman.elvis:elvis:3.0'
2.使用
WifiDialog(act).Builder().create().show()
3.wifiDialog
-
-
import android.annotation.SuppressLint
-
import android.app.AlertDialog
-
import android.app.Dialog
-
import android.
content.Context
-
import android.net.wifi.ScanResult
-
import android.util.TypedValue
-
import android.view.LayoutInflater
-
import android.view.View
-
import android.view.ViewGroup
-
import android.widget.EditText
-
import android.widget.LinearLayout
-
import android.widget.Toast
-
import androidx.appcompat.app.AppCompatActivity
-
import androidx.recyclerview.widget.LinearLayoutManager
-
import androidx.recyclerview.widget.RecyclerView
-
import com.chad.library.adapter.base.BaseQuickAdapter
-
import com.chad.library.adapter.base.viewholder.BaseViewHolder
-
import com.kongzue.dialog.v
3.WaitDialog
-
import com.snjk.recyclebox.R
-
import com.snjk.recyclebox.offline.utils.dp
2pxI
-
import com.snjk.recyclebox.offline.utils.toast
-
import com.thanosfisherman.wifiutils.WifiUtils
-
import com.thanosfisherman.wifiutils.wifiConnect.ConnectionErrorCode
-
import com.thanosfisherman.wifiutils.wifiConnect.ConnectionSuccessListener
-
import io.reactivex.Observable
-
import io.reactivex.disposables.Disposable
-
import io.reactivex.schedulers.Schedulers
-
import java.util.concurrent.TimeUnit
-
-
class WifiDialog(val activity: AppCompatActivity) : Dialog(activity) {
-
inner
class Builder {
-
private lateinit var dialog: WifiDialog
-
private lateinit var view: View
-
private var wifiAdapter
= WifiAdapter()
-
private val wifiUtils
= WifiUtils.withContext(context)
-
private var disposable: Disposable?
=
null
-
-
@SuppressLint(
"InflateParams")
-
private fun initView() {
-
val inflater
=
-
context.getSystemService(Context.LAYOUT_INFLATER_SERVICE)
as LayoutInflater
-
-
view
= inflater.inflate(R.layout.dialog_wifi,
null)
-
dialog
= WifiDialog(activity)
-
dialog.addContentView(
-
view,
-
ViewGroup.LayoutParams(
-
LinearLayout.LayoutParams.WRAP_
CONTENT,
-
300.dp
2pxI(activity)
-
)
-
)
-
dialog.setCancelable(
true)
-
dialog.setCanceledOnTouchOutside(
true)
-
}
-
-
-
fun create(): Builder {
-
initView()
-
wifiAdapter.setOnItemClickListener { _, _, position -
>
-
shouPwdView(wifiAdapter.getItem(position))
-
}
-
view.findViewById
<RecyclerView
>(R.id.wifiRcv).apply {
-
adapter
= wifiAdapter
-
layoutManager
= LinearLayoutManager(context, LinearLayoutManager.VERTICAL,
false)
-
}
-
wifiUtils.enableWifi {
if (!it)
"wifi打开失败,请联系管理员".toast() }
-
Observable.interval(
30, TimeUnit.SECONDS)
-
.observeOn(Schedulers.io())
-
.doOnSubscribe { d -
> disposable
= d }
-
.doOnNext { scanWifi() }
-
.subscribe()
-
dialog.setOnDismissListener { disposable?.dispose() }
-
scanWifi()
-
return this
-
}
-
-
fun show()
= dialog.show()
-
-
private fun shouPwdView(sr: ScanResult) {
-
val et
= EditText(context)
-
AlertDialog.Builder(context)
-
.setTitle(
"请输入密码")
-
.setView(et)
-
.setNegativeButton(
"取消") { d, _ -
> d.dismiss() }
-
.setPositiveButton(
"连接") { d, _ -
>
-
d.dismiss()
-
showLoading()
-
WifiUtils.withContext(context)
-
.connectWith(sr.SSID, et.text.toString())
-
.setTimeout(
10000)
-
.onConnectionResult(
object : ConnectionSuccessListener {
-
override fun success() {
-
"wifi连接成功".toast()
-
hideLoading()
-
dialog.dismiss()
-
}
-
-
override fun failed(errorCode: ConnectionErrorCode) {
-
Toast.makeText(context,
"EPIC FAIL!$errorCode", Toast.
LENGTH_SHORT)
-
.show()
-
hideLoading()
-
}
-
})
-
.
start()
-
}.show()
-
}
-
-
-
private fun scanWifi() {
-
wifiUtils.scanWifi {
-
if (it.isNullOrEmpty())
"附件没有可用的wifi".toast()
else {
-
wifiAdapter.setNewInstance(it)
-
wifiAdapter.notifyDataSetChanged()
-
/
/
/
/以下为打印数据
-
/
/ val sb
= StringBuffer()
-
/
/ it.map {
-
/
/ sb.append(
"设备名(SSID) ->${it.SSID}\t")
-
/
/ .append(
"信号强度 ->${it.level}\t")
-
/
/ .append(
"BSSID ->${it.BSSID}\t")
-
/
/ .append(
"level ->${it.level}\t")
-
/
/ .append(
"wifi -> ${it}")
-
/
/ }
-
/
/ Log.e(
"TTT", sb.toString())
-
}
-
}.
start()
-
}
-
-
private fun showLoading() {
-
WaitDialog.show(activity,
"wifi连接中...")
-
.setOnBackClickListener {
false }
-
}
-
-
private fun hideLoading() {
-
WaitDialog.dismiss()
-
}
-
-
inner
class WifiAdapter : BaseQuickAdapter
<ScanResult, BaseViewHolder
>(R.layout.item_wifi) {
-
override fun convert(holder: BaseViewHolder, item: ScanResult) {
-
holder.setImageResource(
-
R.id.wifiIcon,
when (item.level) {
-
in
0 downTo -
50 -
> R.drawable.icon_wifi_full
-
in -
50 downTo -
70 -
> R.drawable.icon_wifi_good
-
in -
70 downTo -
90 -
> R.drawable.icon_wifi_little
-
else -
> R.drawable.icon_wifi_weak
-
}
-
)
-
.setText(R.id.wifiName, item.SSID)
-
.setGone(R.id.wifiConn, !wifiUtils.isWifiConnected(item.SSID))
-
.setGone(R.id.wifiToConn, wifiUtils.isWifiConnected(item.SSID))
-
}
-
}
-
}
-
}
4.wifiDialog布局
-
<?xml version
=
"1.0" encoding
=
"utf-8"?
>
-
<LinearLayout xmlns:android
=
"http://schemas.android.com/apk/res/android"
-
xmlns:app
=
"http://schemas.android.com/apk/res-auto"
-
xmlns:tools
=
"http://schemas.android.com/tools"
-
android:layout_width
=
"260dp"
-
android:layout_height
=
"320dp"
-
android:layout_gravity
=
"center"
-
android:background
=
"@drawable/ic_wifi_dialog"
-
android:orientation
=
"vertical"
>
-
-
<TextView
-
android:layout_width
=
"match_parent"
-
android:layout_height
=
"46dp"
-
android:gravity
=
"center"
-
android:text
=
"wifi列表"
-
android:textSize
=
"14sp"
/
>
-
-
<View
-
android:layout_width
=
"match_parent"
-
android:layout_height
=
"1px"
-
android:background
=
"#cfcfcf"
/
>
-
-
<androidx.recyclerview.widget.RecyclerView
-
android:id
=
"@+id/wifiRcv"
-
android:layout_width
=
"match_parent"
-
android:layout_height
=
"match_parent"
-
app:layoutManager
=
"androidx.recyclerview.widget.LinearLayoutManager"
-
tools:listitem
=
"@layout/item_wifi"
/
>
-
<
/LinearLayout
>
5.wifi布局
-
<?xml version
=
"1.0" encoding
=
"utf-8"?
>
-
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android
=
"http://schemas.android.com/apk/res/android"
-
xmlns:app
=
"http://schemas.android.com/apk/res-auto"
-
xmlns:tools
=
"http://schemas.android.com/tools"
-
android:layout_width
=
"match_parent"
-
android:layout_height
=
"46dp"
-
android:orientation
=
"vertical"
>
-
-
<TextView
-
android:id
=
"@+id/wifiName"
-
android:layout_width
=
"wrap_content"
-
android:layout_height
=
"match_parent"
-
android:layout_gravity
=
"center_vertical"
-
android:layout_weight
=
"1"
-
android:gravity
=
"center_vertical"
-
android:paddingStart
=
"10dp"
-
android:paddingEnd
=
"10dp"
-
android:textSize
=
"14sp"
-
app:layout_constraintLeft_toLeftOf
=
"parent"
-
app:layout_constraintTop_toTopOf
=
"parent"
-
tools:text
=
"snkj"
/
>
-
-
<ImageView
-
android:id
=
"@+id/wifiIcon"
-
android:layout_width
=
"16dp"
-
android:layout_height
=
"16dp"
-
android:src
=
"@drawable/icon_wifi_full"
-
app:layout_constraintBottom_toBottomOf
=
"parent"
-
app:layout_constraintLeft_toRightOf
=
"@+id/wifiName"
-
app:layout_constraintTop_toTopOf
=
"parent"
-
tools:ignore
=
"ContentDescription"
/
>
-
-
<View
-
android:layout_width
=
"match_parent"
-
android:layout_height
=
"1px"
-
android:background
=
"#efefef"
-
app:layout_constraintBottom_toBottomOf
=
"parent"
-
app:layout_constraintLeft_toLeftOf
=
"parent"
-
app:layout_constraintRight_toRightOf
=
"parent"
/
>
-
-
<TextView
-
android:id
=
"@+id/wifiConn"
-
android:layout_width
=
"wrap_content"
-
android:layout_height
=
"match_parent"
-
android:gravity
=
"center_vertical"
-
android:paddingStart
=
"10dp"
-
android:paddingEnd
=
"10dp"
-
android:text
=
"已连接"
-
android:textColor
=
"#009900"
-
android:textSize
=
"12sp"
-
android:visibility
=
"gone"
-
app:layout_constraintBottom_toBottomOf
=
"parent"
-
app:layout_constraintRight_toRightOf
=
"parent"
-
app:layout_constraintTop_toTopOf
=
"parent"
/
>
-
-
<TextView
-
android:id
=
"@+id/wifiToConn"
-
android:layout_width
=
"wrap_content"
-
android:layout_height
=
"match_parent"
-
android:gravity
=
"center_vertical"
-
android:paddingStart
=
"10dp"
-
android:paddingEnd
=
"10dp"
-
android:text
=
"连接"
-
android:textColor
=
"#0000cc"
-
android:textSize
=
"12sp"
-
app:layout_constraintBottom_toBottomOf
=
"parent"
-
app:layout_constraintRight_toRightOf
=
"parent"
-
app:layout_constraintTop_toTopOf
=
"parent"
/
>
-
-
-
<
/androidx.constraintlayout.widget.ConstraintLayout
>
更多推荐
所有评论(0)