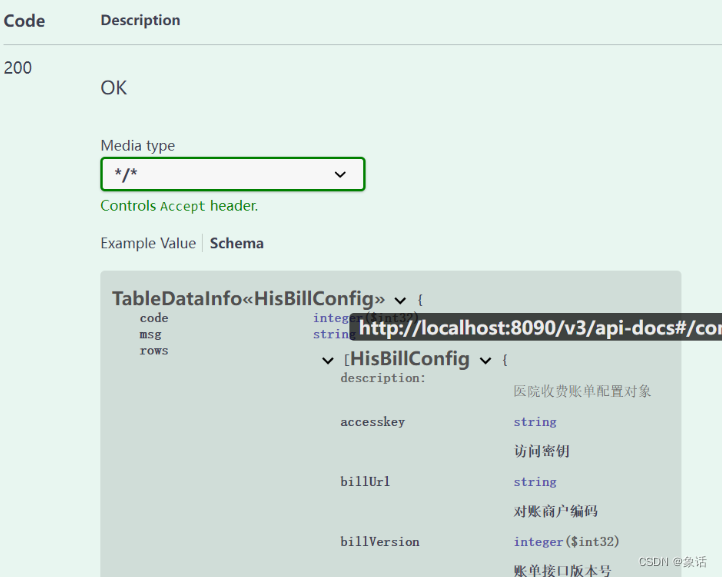
若依框架AjaxResult改造适应Swagger接口文档
若依框架后端使用的响应对象AjaxResult,和Swagger存在不兼容问题,导致返回体即使使用了Swagger注解,但是Swagger接口文档中,不显示返回体的对象Swagger文档:
·
一、 概述
若依框架后端使用的响应对象AjaxResult,和Swagger存在不兼容问题,导致返回体即使使用了Swagger注解,但是Swagger接口文档中,不显示返回体的对象Swagger文档:
若依Gitee上,也存在此问题:
https://gitee.com/y_project/RuoYi-Vue/commit/6805a96e533f56b86aaeecccc2693c6ff4064d31
二、原因
1、若依的AjaxResult没有指定泛型;
2、若依的AjaxResult对象使用了Map类型;
三、改造AjaxResult
改动最小化,保留AjaxResult类名以及原有的方法;
@Data
public class AjaxResult<T> implements Serializable {
private static final long serialVersionUID = 1L;
/**
* 是否成功 true or false
*/
private boolean success;
/**
* 状态码
*/
private int code;
/**
* 返回内容
*/
private String msg;
/**
* 数据对象
*/
private T data;
/**
* 状态类型
*/
public enum Type
{
/** 成功 */
SUCCESS(0),
/** 警告 */
WARN(301),
/** 错误 */
ERROR(500);
private final int value;
Type(int value)
{
this.value = value;
}
public int value()
{
return this.value;
}
}
/**
* 初始化一个新创建的 AjaxResult 对象,使其表示一个空消息。
*/
public AjaxResult()
{
}
/**
* 初始化一个新创建的 AjaxResult 对象
*
* @param type 状态类型
* @param msg 返回内容
* @param data 数据对象
*/
public AjaxResult(Type type, String msg, T data) {
this.code = type.value();
this.msg = msg;
if (StringUtils.isNotNull(data)) {
this.data = data;
}
if (type.value == Type.SUCCESS.value) {
this.success = Boolean.TRUE;
} else {
this.success = Boolean.FALSE;
}
}
/**
* 返回成功消息
*
* @return 成功消息
*/
public static AjaxResult success()
{
return AjaxResult.success("操作成功");
}
/**
* 返回成功数据
*
* @return 成功消息
*/
public static <U> AjaxResult<U> success(U data)
{
return AjaxResult.success("操作成功", data);
}
/**
* 返回成功消息
*
* @param msg 返回内容
* @return 成功消息
*/
public static AjaxResult success(String msg)
{
return AjaxResult.success(msg, null);
}
/**
* 返回成功消息
*
* @param msg 返回内容
* @param data 数据对象
* @return 成功消息
*/
public static <U> AjaxResult<U> success(String msg, U data)
{
return new AjaxResult(Type.SUCCESS, msg, data);
}
/**
* 返回警告消息
*
* @param msg 返回内容
* @return 警告消息
*/
public static AjaxResult warn(String msg)
{
return AjaxResult.warn(msg, null);
}
/**
* 返回警告消息
*
* @param msg 返回内容
* @param data 数据对象
* @return 警告消息
*/
public static <U> AjaxResult<U> warn(String msg, U data)
{
return new AjaxResult(Type.WARN, msg, data);
}
/**
* 返回错误消息
*
* @return
*/
public static AjaxResult error()
{
return AjaxResult.error("操作失败");
}
/**
* 返回错误消息
*
* @param msg 返回内容
* @return 警告消息
*/
public static AjaxResult error(String msg)
{
return AjaxResult.error(msg, null);
}
/**
* 返回错误消息
*
* @param msg 返回内容
* @param data 数据对象
* @return 警告消息
*/
public static <U> AjaxResult<U> error(String msg, U data)
{
return new AjaxResult(Type.ERROR, msg, data);
}
/**
* 方便链式调用
*
* @param key 键
* @param value 值
* @return 数据对象
*/
@Deprecated
public AjaxResult put(String key, Object value) {
//super.put(key, value);
return this;
}
/**
* 是否为成功消息
*
* @return 结果
*/
public boolean isSuccess() {
return success;
}
public String getMsg() {
return msg;
}
public Integer getCode() {
return code;
}
}
四、改造TableDataInfo
public class TableDataInfo<T> implements Serializable
{
private static final long serialVersionUID = 1L;
/** 总记录数 */
private long total;
/** 列表数据 */
private List<T> rows;
/** 消息状态码 */
private int code;
/** 消息内容 */
private String msg;
/**
* 表格数据对象
*/
public TableDataInfo()
{
}
/**
* 分页
*
* @param list 列表数据
* @param total 总记录数
*/
public TableDataInfo(List<T> list, int total)
{
this.rows = list;
this.total = total;
}
public long getTotal()
{
return total;
}
public void setTotal(long total)
{
this.total = total;
}
public List<T> getRows()
{
return rows;
}
public void setRows(List<T> rows)
{
this.rows = rows;
}
public int getCode()
{
return code;
}
public void setCode(int code)
{
this.code = code;
}
public String getMsg()
{
return msg;
}
public void setMsg(String msg)
{
this.msg = msg;
}
}
BaseController中的getDataTable()方法增加泛型:
/**
* 响应请求分页数据
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
protected <T> TableDataInfo<T> getDataTable(List<T> list)
{
TableDataInfo rspData = new TableDataInfo();
rspData.setCode(0);
rspData.setRows(list);
rspData.setTotal(new PageInfo(list).getTotal());
return rspData;
}
五、使用示例
1、查询接口返回AjaxResult时,增加泛型,对于没有返回的接口可以使用 AjaxResult<Void>来消除报黄:
@GetMapping("/calender")
@ApiOperation("对账日历")
@ResponseBody
public AjaxResult<RecordCalenderDTO> demo() {
return AjaxResult.success(new RecordCalenderDTO());
}
2、分页查询接口返回TableDataInfo,增加泛型:
@ApiOperation("查询医院收费账单配置列表")
@PostMapping("/list")
@ResponseBody
public TableDataInfo<HisBillConfig> list(HisBillConfig hisBillConfig) {
startPage();
List<HisBillConfig> list = hisBillConfigService.selectHisBillConfigList(hisBillConfig);
return getDataTable(list);
}
六、Swagger效果
1、返回AjaxResult效果:
2、分页查询TableDataInfo效果:
更多推荐
所有评论(0)