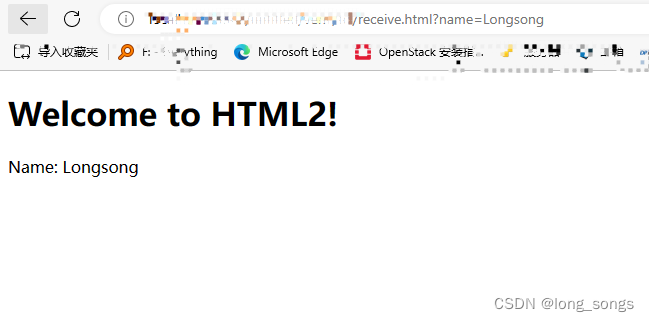
前端HTML网页之间传递数据多种办法,附代码案例
目前常用的有三种办法session传递,cookie传递,url传递url会暴露参数,其余的两个是保存在服务端和浏览器中,不会暴露在地址栏里面下面依次介绍。

一键AI生成摘要,助你高效阅读
问答
·
先看效果
目前常用的有三种办法
session传递,cookie传递,url传递
url会暴露参数,其余的两个是保存在服务端和浏览器中,不会暴露在地址栏里面
使用url:
下面依次介绍
一.session传递
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HTML1</title>
</head>
<body>
<h1>Welcome to HTML1!</h1>
<form method="post" action="receive.html">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
<script>
// 获取表单元素和输入框元素
const form = document.querySelector('form');
const input = document.querySelector('#name');
// 在表单提交时将数据保存到sessionStorage中
form.addEventListener('submit', (event) => {
event.preventDefault();
sessionStorage.setItem('name', input.value);
form.submit();
});
</script>
</body>
</html>
receive.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HTML2</title>
</head>
<body>
<h1>Welcome to HTML2!</h1>
<p>Name: <span id="name"></span></p>
<script>
// 获取session中的数据
const name = sessionStorage.getItem('name');
// 将数据渲染到页面上
document.getElementById('name').textContent = name;
</script>
</body>
</html>
案例说明:
- 在HTML1中,我们使用
form
标签将数据提交到HTML2页面,并设置method
为post
,action
为HTML2的文件路径。- 在HTML2中,我们使用JavaScript获取session中的数据,然后将数据渲染到页面上。我们可以使用
sessionStorage
对象来存储数据,它可以在不同的页面之间共享数据。在这里,我们存储了用户输入的姓名,并在HTML2中获取并渲染了这个数据。
如果传递失败,检查一下以下问题:
- HTML1文件中的表单
action
属性设置错误,导致数据无法传递到HTML2页面。在这里,需要确保HTML1中表单的action
属性设置为HTML2的文件路径,如action="html2.html"
。 - HTML2文件中的JavaScript代码没有正确地从sessionStorage中获取数据。需要确保使用正确的key来获取sessionStorage中的数据。在这里,我们使用的key是
name
,所以需要确保获取数据的代码中使用的也是这个key,如const name = sessionStorage.getItem('name');
。 - 在本地演示时,需要确保HTML1和HTML2两个文件都在同一个服务器上运行,否则sessionStorage无法正常工作。
- 如果使用了浏览器的隐身模式,也可能导致sessionStorage无法正常工作。 如果以上几个原因都不是问题的话,建议检查浏览器的开发者工具中是否有相关的错误信息或警告信息。
二.cookie传递
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HTML1</title>
</head>
<body>
<h1>Welcome to HTML1!</h1>
<form method="post" action="receive.html">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
<script>
// 获取表单元素和输入框元素
const form = document.querySelector('form');
const input = document.querySelector('#name');
// 在表单提交时将数据保存到cookie中
form.addEventListener('submit', (event) => {
event.preventDefault();
document.cookie = `name=${input.value}`;
form.submit();
});
</script>
</body>
</html>
receive.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HTML2</title>
</head>
<body>
<h1>Welcome to HTML2!</h1>
<p>Name: <span id="name"></span></p>
<script>
// 获取cookie中的数据
const cookies = document.cookie.split(';');
let name = '';
for (let cookie of cookies) {
cookie = cookie.trim();
if (cookie.startsWith('name=')) {
name = cookie.substring(5);
break;
}
}
// 将数据渲染到页面上
document.getElementById('name').textContent = name;
</script>
</body>
</html>
案例解释:
- 在HTML1中,我们使用
form
标签将数据提交到HTML2页面,并设置method
为post
,action
为HTML2的文件路径。- 在HTML1中,我们使用JavaScript监听表单的提交事件,在事件处理程序中将数据保存到cookie中,并且在页面跳转之前提交表单。这样,在HTML2中就可以通过cookie获取到数据并渲染了。
- 在HTML2中,我们使用JavaScript获取cookie中的数据,并渲染到页面上。我们可以使用
document.cookie
来访问cookie,它返回一个字符串,包含所有cookie的键值对。在这里,我们遍历这个字符串,找到名为name
的cookie,并获取它的值。然后将这个值渲染到页面上。
三.url传输
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HTML1</title>
</head>
<body>
<h1>Welcome to HTML1!</h1>
<form method="get" action="receive.html">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
<script>
// 获取表单元素和输入框元素
const form = document.querySelector('form');
const input = document.querySelector('#name');
// 在表单提交时将数据拼接到URL中
form.addEventListener('submit', (event) => {
event.preventDefault();
const url = new URL(form.action);
url.searchParams.set('name', input.value);
window.location.href = url.toString();
});
</script>
</body>
</html>
receive.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HTML2</title>
</head>
<body>
<h1>Welcome to HTML2!</h1>
<p>Name: <span id="name"></span></p>
<script>
// 获取URL中的参数
const params = new URLSearchParams(window.location.search);
const name = params.get('name');
// 将数据渲染到页面上
document.getElementById('name').textContent = name;
</script>
</body>
</html>
案例解释:
- 在HTML1中,我们使用
form
标签将数据提交到HTML2页面,并设置method
为get
,action
为HTML2的文件路径。- 在HTML1中,我们使用JavaScript监听表单的提交事件,在事件处理程序中将数据拼接到URL中,并跳转到这个URL。这样,在HTML2中就可以通过URL获取到数据并渲染了。
- 在HTML2中,我们使用JavaScript获取URL中的参数,并渲染到页面上。我们可以使用
URLSearchParams
来访问URL中的参数,它提供了一些实用的方法,如get()
、set()
等。在这里,我们获取名为name
的参数,并将它渲染到页面上。
更多推荐
所有评论(0)