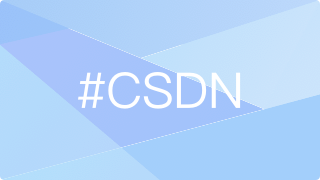
Linux之获取系统时间(time函数、gettimeofday函数)
Linux中获取系统时间函数介绍

一、相关函数介绍
1.1 ctime()函数
#include <time.h>
char *ctime(const time_t *timer)
①函数功能:将日历时间参数time转换为一个表示本地当前时间的字符串;
②函数参数:timer为time()函数获得
③函数返回值:返回字符串格式(星期 月 日 小时:分:秒 年);
1.2 localtime()函数
struct tm *localtime(const time_t *timer);
tm结构:
struct tm{
int tm_sec;
int tm_min;
int tm_hour;
int tm_mday;
int tm_mon;
int tm_year;
int tm_wday;
int tm_yday;
int tm_isdst;
};
①函数功能:使用timer的值填充tm结构体,其值会被分解为tm结构,并用本地时区表示;
②函数参数:timer为time()函数获得;
③函数返回值:以tm结构表达的时间;
1.3 strftime()函数
#include <time.h>
size_t strftime(char *str, size_t maxsize, const char *format, const struct tm *timeptr)
①函数功能:年-月-日 小时:分钟:秒 格式的日期字符串转换成其它形式的字符串;
②函数参数:
第一个参数→str为指向目标数组的指针,用来复制产生的C字符串;
第二个参数→maxsize为被复制到str的最大字符数;
第三个参数→format为C字符串,包含了普通字符和特殊格式说明符的任何组合。这些格式说明符由函数替换为表示 tm 中所指定时间的相对应值;
第四个参数→timeptr指向 tm 结构的指针,tm结构体详情可见ctime()函数;
③函数返回值:如果产生的 C 字符串小于 maxsize 个字符(包括空结束字符),则会返回复制到 str 中的字符总数(不包括空结束字符),否则返回零。
二、获取系统时间方法
2.1.1 time()函数
#include <time.h>
time_t time(time_t *timer);
time_t 是一个unsigned long类型,
①函数功能:得到当前日历时间或设置日历时间
②函数参数:
timer = NULL时,为得到当前日历时间(从1970-01-01 00:00:00到现在的秒数);
timer = 时间数值时,用于设置日历时间,其返回值存储在timer中;
③函数返回值:返回当前日历时间
2.1.2 time()案例
输出时间格式:Mon Mar 20 15 : 51 : 31 2023
#include <stdio.h>
#include <time.h>
#include <stdlib.h>
#include <sys/time.h>
int printf_time(void);
int main(int argc,char **argv)
{
printf_time();//调用获取时间函数
return 0;
}
int printf_time(void)
{
time_t timep;//time_t 是一个适合存储日历时间类型
time(&timep);
char *s = ctime(&timep);//ctime()把日期和时间转换为字符串
printf("date:%s",s);
return 0;
}
输出结果:
date:Mon Mar 20 15:51:31 2023
2.2.1 gettimeofday()函数
#include <unistd.h>
#include <sys/time.h>
int gettimeofday(struct timeval *tv,struct timezone *tz);
timeval结构体:
struct timeval{
long tv_sec; // 秒数
long tv_usec; // 微秒数
};
timezone结构体:
struct timezone{
int tz_minuteswest;//格林威治时间往西方的时差
int tz_dsttime;//DST 时间的修正方式
};
①函数功能:把目前的时间由tv所指向的结构体返回,当地时区的信息则放到tz所指的结构中;
②函数参数:
第一个参数→tv为timeval结构体;
第二个参数→tz为timezone结构体;
③函数返回值:在gettimeofday()函数中的俩个参数都可以为NULL,为NULL时对应的结构体将不返回值。成功,返回0;失败,返回-1,原因存在errno中;
2.2.2 gettimeofday()案例
输出时间格式:2023-03-20 20:38:37
#include <stdio.h>
#include <time.h>
#include <stdlib.h>
#include <sys/time.h>
int get_time(char *buff,int len);
int main(int argc, const char **argv)
{
char buff[40];
get_time(buff, sizeof(buff));
printf("time:%s\n", buff);
return 0;
}
int get_time(char *buff,int len)
{
struct timeval tv;
struct tm* ptm;
char time_string[40];
gettimeofday(&tv, NULL);
ptm = localtime (&(tv.tv_sec));
strftime (time_string, sizeof(time_string), "%Y-%m-%d %H:%M:%S", ptm); //输出格式为: 2022-03-30 20:38:37
snprintf (buff, len, "%s", time_string);
return 0;
}
输出结果:
time:2023-03-20 20:38:37
更多推荐
所有评论(0)