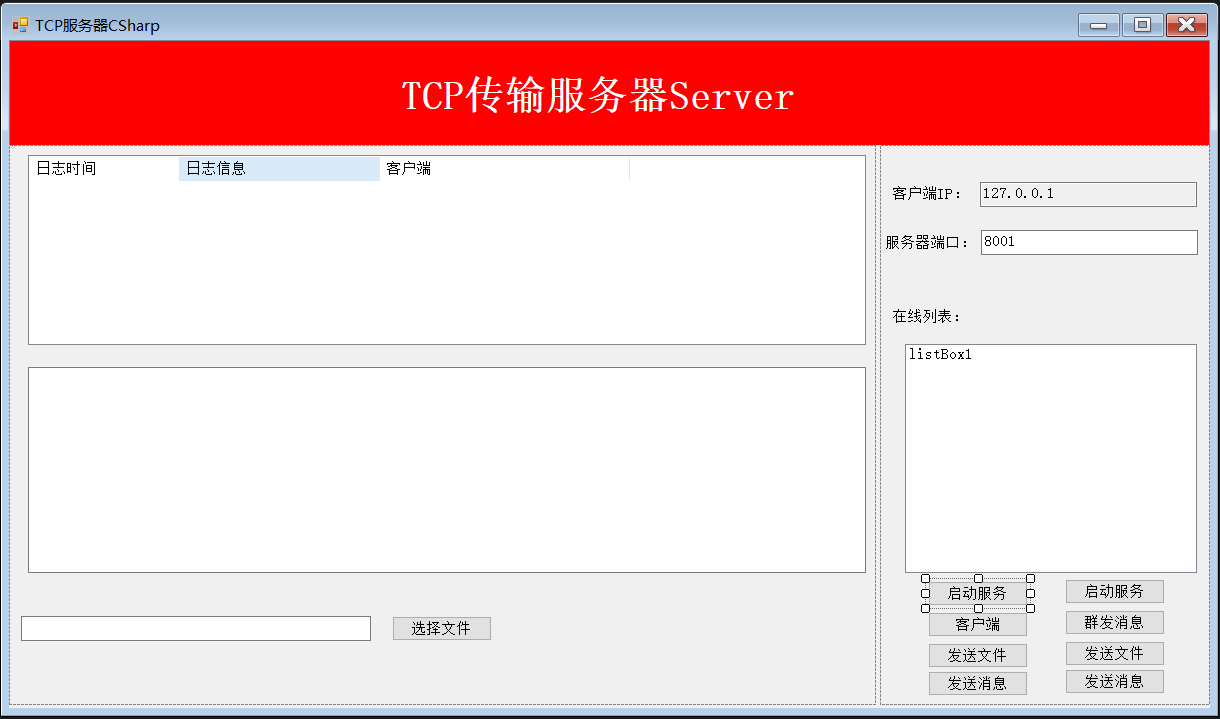
C#的Socket实现网络通信
服务器端程序的编写步骤:1:调用Socket()函数编写创建一个用于创建通信的套接字2:给已经创建的套接字绑定一个端口,这一般通过设置网络套接字地址和调用API3:调用listen()函数使套接字成为一个监听的套接字4:调用accept()函数来接收客户端的连接,这样就可以与客户端进行连接5:处理客户端的连接请求6:终止连接
·
Socket服务器端程序的编写步骤
/// 1:调用Socket()函数编写创建一个用于创建通信的套接字
private Socket socketServer=new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
/// 2:给已经创建的套接字绑定一个端口,这一般通过设置网络套接字地址和调用API
IPEndPoint ipe = new IPEndPoint(IPAddress.Parse(this.txt_IP.Text), int.Parse(this.txt_Port.Text));
socketServer.Bind(ipe);
/// 3:调用listen()函数使套接字成为一个监听的套接字
socketServer.Listen(10);
/// 4:调用accept()函数来接收客户端的连接,这样就可以与客户端进行连接
Socket socketClient = socketServer.Accept(); //调用accepy()函数来接受客户端的连接,这就可以与客户端进行连接
string client = socketClient.RemoteEndPoint.ToString();
CurrentClientList.Add(client, socketClient);
pos.ppp = client;
AddLog(0,client+"服务器开启成功!");
UpdateOnline(client, true);
Task.Run(() =>
{
ReceiveMessage(socketClient);
});
/// 5:处理客户端的连接请求
private void ReceiveMessage(Socket socketClient)//接收客户端数据的方法体
{
while (true)
{
byte[] buffer = new byte[1024 * 1024 * 10];//创建一个缓冲区
int length = -1;
string client = socketClient.RemoteEndPoint.ToString();
try
{
length = socketClient.Receive(buffer);
}
catch (Exception)
{
UpdateOnline(client,false);
AddLog(0,client+"下线了");
CurrentClientList.Remove(client);
throw;
}
if (length > 0)
{
string msg=Encoding.Default.GetString(buffer, 0, length);
AddLog(0,"来自"+socketClient.RemoteEndPoint.ToString()+":"+msg);
}
else
{
UpdateOnline(client, false);
CurrentClientList.Remove(client);
AddLog(0, socketClient.RemoteEndPoint.ToString()+"断开连接");
break;
}
}
}
/// 6:终止连接
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace CSharp网络调试助手开发
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
this.Load += Form1_Load;
}
/// <summary>
/// 服务器端程序的编写步骤
/// 1:调用Socket()函数编写创建一个用于创建通信的套接字
/// 2:给已经创建的套接字绑定一个端口,这一般通过设置网络套接字地址和调用API
/// 3:调用listen()函数使套接字成为一个监听的套接字
/// 4:调用accept()函数来接收客户端的连接,这样就可以与客户端进行连接
/// 5:处理客户端的连接请求
/// 6:终止连接
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private Socket socketServer;
//创建一个字典集合 键是ClientIP 值是SocketClient
private Dictionary<string, Socket> CurrentClientList = new Dictionary<string, Socket>();
Post pos = new Post();
private void Form1_Load(object sender, EventArgs e)
{
this.listView1.Columns[1].Width = this.listView1.ClientSize.Width - this.listView1.Columns[0].Width;
}
private void splitContainer1_Panel1_Paint(object sender, PaintEventArgs e)
{
}
/// <summary>
/// 连接开启服务
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void btn_StartServer_Click(object sender, EventArgs e)
{
socketServer = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);//第一步调用Socket()函数创建一个套接字
IPEndPoint ipe = new IPEndPoint(IPAddress.Parse(this.txt_IP.Text), int.Parse(this.txt_Port.Text));//根据IPAdress以及端口号创建IPE对象
try
{
socketServer.Bind(ipe); //第二步:bind() 关联一个本地地址到套接字socketServer
}
catch (Exception ex)
{
AddLog(2, "服务器开始失败!" + ex.Message);//如果失败写入日志
return;
}
socketServer.Listen(10);//第三步:调用Listen()函数使套接字成为一个套接字
Task.Run(() =>
{
CheckListening();
});
AddLog(0, "服务器开始成功!!");
this.btn_StartServer.Enabled = false;
}
/// <summary>
/// 检查监听线程的方法体
/// </summary>
private void CheckListening()
{
while (true)
{
Socket socketClient = socketServer.Accept(); //调用accepy()函数来接受客户端的连接,这就可以与客户端进行连接
string client = socketClient.RemoteEndPoint.ToString();
CurrentClientList.Add(client, socketClient);
pos.ppp = client;
AddLog(0,client+"服务器开启成功!");
UpdateOnline(client, true);
Task.Run(() =>
{
ReceiveMessage(socketClient);
});
}
}
/// <summary>
/// 接收客户端数据的方法体
/// </summary>
/// <param name="socketClient"></param>
private void ReceiveMessage(Socket socketClient)//接收客户端数据的方法体
{
while (true)
{
byte[] buffer = new byte[1024 * 1024 * 10];//创建一个缓冲区
int length = -1;
string client = socketClient.RemoteEndPoint.ToString();
try
{
length = socketClient.Receive(buffer);
}
catch (Exception)
{
UpdateOnline(client,false);
AddLog(0,client+"下线了");
CurrentClientList.Remove(client);
throw;
}
if (length > 0)
{
string msg=Encoding.Default.GetString(buffer, 0, length);
AddLog(0,"来自"+socketClient.RemoteEndPoint.ToString()+":"+msg);
}
else
{
UpdateOnline(client, false);
CurrentClientList.Remove(client);
AddLog(0, socketClient.RemoteEndPoint.ToString()+"断开连接");
break;
}
}
}
#region 接收信息的方法
private string CurrentTime
{
get { return DateTime.Now.ToString("yyyy-HH-dd HH:mm:ss"); }
}
private void AddLog(int index, string info)
{
Socket socketClient = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
if (!this.listView1.InvokeRequired)
{
ListViewItem Ist = new ListViewItem("" + CurrentTime, index);
Ist.SubItems.Add(info);
listView1.Items.Insert(0, Ist);
//listView1.Items.Insert(2, socketClient.RemoteEndPoint.ToString());
}
else
{
Invoke(new Action(() =>
{
ListViewItem ist = new ListViewItem("" + CurrentTime, index);
ist.SubItems.Add(info);
listView1.Items.Insert(0, ist);
//listView1.Items.Insert(2, socketClient.RemoteEndPoint.ToString());
}));
}
}
#endregion
#region 在线列表更新
/// <summary>
/// 在线列表更新
/// </summary>
/// <param name="client"></param>
/// <param name="operate"></param>
private void UpdateOnline(string client, bool operate)
{
if (!this.listBox1.InvokeRequired)
{
if (operate)
{
this.listBox1.Items.Add(client);
}
else
{
foreach (string item in this.listBox1.Items)
{
if (item == client)
{
this.listBox1.Items.Remove(item);
break;
}
}
}
}
else
{
Invoke((Action)(() =>
{
if (operate)
{
this.listBox1.Items.Add(client);
}
else
{
foreach (string item in this.listBox1.Items)
{
if (item == client)
{
this.listBox1.Items.Remove(item);
break;
}
}
}
}));
}
}
#endregion
private void splitContainer1_Panel2_Paint(object sender, PaintEventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog dialog=new OpenFileDialog();
dialog.Multiselect= true;//允许打开多个文件
dialog.Title = "请选择文件";
dialog.Filter = "txt格式(*.txt)|*.txt|所有文件|*.*";//选择的文件格式
if (dialog.ShowDialog()==System.Windows.Forms.DialogResult.OK)
{
string file = dialog.FileName;
}
}
private void button4_Click(object sender, EventArgs e)
{
OpencvSharp sharp = new OpencvSharp();
sharp.Show();
}
private void button3_Click(object sender, EventArgs e)
{
if (pos.ppp != null)
{
try
{
string post = pos.ppp;
string client = post;
CurrentClientList[client].Send(Encoding.Default.GetBytes(this.textBox2.Text.Trim()));
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
throw;
}
}
else
{
MessageBox.Show("请选择你要发送的客户端!");
}
}
private void button6_Click(object sender, EventArgs e)
{
}
private void Form1_Resize(object sender, EventArgs e)
{
}
}
}
Post.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp网络调试助手开发
{
public class Post
{
public string ppp;
}
}
更多推荐
所有评论(0)