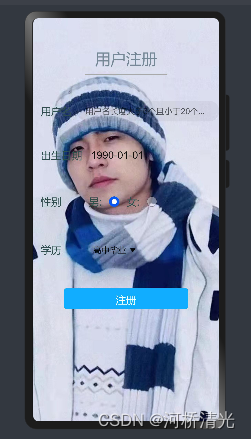
HarmonyOS实现简易的用户注册页面
通过文本输入框、日期选择控件、单选按钮、下拉菜单和页面路由实现简易的用户注册案例。下图是示例的基本结构。
·
-
前言:
通过文本输入框、日期选择控件、单选按钮、下拉菜单和页面路由实现简易的用户注册案例。
下图是示例的基本结构。
-
代码实现——index页面:
1.用户名——利用input组件进行用户名的输入,长度为0时会提示用户名不能为空,不为0但小于零时会提示长度小于6。
<!-- username -->
<div class="item-container">
<text class="item-title">{{ $t('Strings.userName') }}</text>
<div class="item-content">
<input class="input-text" placeholder="{{ $t('Strings.userNamePrompt') }}" onchange="getName"></input>
</div>
</div>
//js部分
getName(e) {
this.name = e.value;
console.info("name=" + this.name)
},
2.日期选择——利用picker组件设置type属性为date,起始日期selected为1990-01-01,最晚日期为2022-11-17。
<!-- date -->
<div class="item-container">
<text class="item-title">{{ $t('Strings.date') }}</text>
<div class="item-content">
<picker type="date" end="2022-11-17" selected="1990-01-01" value="{{ date }}" onchange="getDate"></picker>
</div>
</div>
// js部分
getDate(e) {
this.date = e.year + '-' + (e.month + 1) + '-' + e.day;
console.info("date=" + this.date)
},
3.性别——利用input的type属性设置为radio单选按钮,并且在每一个input设置一个id,酱紫就可以利用label组件的target属性指向该id,实现点击文本也能选中按钮。
<!-- gender -->
<div class="item-container">
<text class="item-title">{{ $t('Strings.gender') }}</text>
<div class="item-content">
<label target="radio1">{{ $t('Strings.male') }}:</label>
<input id="radio1" type="radio" name="radio" value="{{ $t('Strings.male') }}" onchange="getMaleGender"
checked="true"></input>
<label target="radio2">{{ $t('Strings.female') }}:</label>
<input id="radio2" type="radio" name="radio" value="{{ $t('Strings.female') }}" onchange="getFemaleGender">
</input>
</div>
</div>
// js部分
getFemaleGender(e) {
if (e.checked) {
this.gender = 'Strings.female'
console.info("gender =" + this.gender)
}
},
getMaleGender(e) {
if (e.checked) {
this.gender = 'Strings.male'
console.info("gender =" + this.gender)
}
},
4.教育学历——利用select下拉菜单组件以及option实现学历的选择,此处需要默认选中时可以利用selected="true"即可实现。
<!-- education -->
<div class="item-container">
<text class="item-title">{{ $t('Strings.education') }}</text>
<select class="select" onchange="getEducation">
<option value="{{ $t('Strings.graduated') }}" selected="true">{{ $t('Strings.graduated') }}</option>
<option value="{{ $t('Strings.bachelor') }}">{{ $t('Strings.bachelor') }}</option>
<option value="{{ $t('Strings.master') }}">{{ $t('Strings.master') }}</option>
<option value="{{ $t('Strings.doctor') }}">{{ $t('Strings.doctor') }}</option>
</select>
</div>
// js部分
getEducation(e) {
this.education = e.newValue;
console.info("education=" + this.education)
},
5.注册按钮——利用input的type属性设置为button
<div class="button-container">
<input type="button" class="btn" onclick="onRegister" value="{{ $t('Strings.register') }}"/>
</div>
// js部分
onRegister() {
if (this.name.length == 0) {
prompt.showToast({
message: this.$t('Strings.name_null')
})
return;
}
if (this.name.length < 6) {
prompt.showToast({
message: this.$t('Strings.name_short')
})
return;
}
if (this.name.length > 20) {
prompt.showToast({
message: this.$t('Strings.name_long')
})
return;
}
if (this.date.length == 0) {
prompt.showToast({
message: this.$t('Strings.date_null')
})
return;
}
if (this.gender.length == 0) {
prompt.showToast({
message: this.$t('Strings.gender_null')
})
return;
}
if (this.education.length == 0) {
prompt.showToast({
message: this.$t('Strings.education_null')
})
return;
}
router.push({
uri: 'pages/success/success'
})
}
6. index页面完整代码:
<!--index.hml-->
<div class="container">
<div class="image-p">
<image class="page-image cover" src="/common/images/jay.png"></image>
</div>
<div class="page-title-wrap">
<text class="page-title">{{ $t('Strings.componentName') }}
</text>
</div>
<!-- username -->
<div class="item-container">
<text class="item-title">{{ $t('Strings.userName') }}</text>
<div class="item-content">
<input class="input-text" placeholder="{{ $t('Strings.userNamePrompt') }}" onchange="getName"></input>
</div>
</div>
<!-- date -->
<div class="item-container">
<text class="item-title">{{ $t('Strings.date') }}</text>
<div class="item-content">
<picker type="date" end="2022-11-17" selected="1990-01-01" value="{{ date }}" onchange="getDate"></picker>
</div>
</div>
<!-- gender -->
<div class="item-container">
<text class="item-title">{{ $t('Strings.gender') }}</text>
<div class="item-content">
<label target="radio1">{{ $t('Strings.male') }}:</label>
<input id="radio1" type="radio" name="radio" value="{{ $t('Strings.male') }}" onchange="getMaleGender"
checked="true"></input>
<label target="radio2">{{ $t('Strings.female') }}:</label>
<input id="radio2" type="radio" name="radio" value="{{ $t('Strings.female') }}" onchange="getFemaleGender">
</input>
</div>
</div>
<!-- education -->
<div class="item-container">
<text class="item-title">{{ $t('Strings.education') }}</text>
<select class="select" onchange="getEducation">
<option value="{{ $t('Strings.graduated') }}" selected="true">{{ $t('Strings.graduated') }}</option>
<option value="{{ $t('Strings.bachelor') }}">{{ $t('Strings.bachelor') }}</option>
<option value="{{ $t('Strings.master') }}">{{ $t('Strings.master') }}</option>
<option value="{{ $t('Strings.doctor') }}">{{ $t('Strings.doctor') }}</option>
</select>
</div>
<div class="button-container">
<input type="button" class="btn" onclick="onRegister" value="{{ $t('Strings.register') }}"/>
</div>
</div>
//index.js
import prompt from '@system.prompt'
import router from '@system.router'
export default {
data: {
name: '',
date: '1990-01-01',
gender: 'Strings.male',
education: 'Strings.graduated',
},
onInit() {
},
// js部分
getName(e) {
this.name = e.value;
console.info("name=" + this.name)
},
// js部分
getDate(e) {
this.date = e.year + '-' + (e.month + 1) + '-' + e.day;
console.info("date=" + this.date)
},
// js部分
getFemaleGender(e) {
if (e.checked) {
this.gender = 'Strings.female'
console.info("gender =" + this.gender)
}
},
getMaleGender(e) {
if (e.checked) {
this.gender = 'Strings.male'
console.info("gender =" + this.gender)
}
},
// js部分
getEducation(e) {
this.education = e.newValue;
console.info("education=" + this.education)
},
// js部分
onRegister() {
if (this.name.length == 0) {
prompt.showToast({
message: this.$t('Strings.name_null')
})
return;
}
if (this.name.length < 6) {
prompt.showToast({
message: this.$t('Strings.name_short')
})
return;
}
if (this.name.length > 20) {
prompt.showToast({
message: this.$t('Strings.name_long')
})
return;
}
if (this.date.length == 0) {
prompt.showToast({
message: this.$t('Strings.date_null')
})
return;
}
if (this.gender.length == 0) {
prompt.showToast({
message: this.$t('Strings.gender_null')
})
return;
}
if (this.education.length == 0) {
prompt.showToast({
message: this.$t('Strings.education_null')
})
return;
}
router.push({
uri: 'pages/success/success'
})
}
}
/*index.css*/
.container{
flex: 1;
flex-direction: column;
}
.image-p{
width:100%;
position:absolute;
height:100%;
}
.page-title-wrap {
padding-top: 50px;
padding-bottom: 25px;
justify-content: center;
}
.page-title{
padding-top: 10px;
padding-bottom: 10px;
padding-left: 20px;
padding-right: 20px;
border-color: darkgray;
color: lightslategray;
border-bottom-width: 2px;
}
.btn {
width: 300px;
height: 130px;
text-align: center;
border-radius: 5px;
margin-right: 60px;
margin-left: 60px;
margin-top: 30px;
margin-bottom: 30px;
color: #ffffff;
font-size: 20px;
background-color: #0faeff;
}
.item-container {
margin-top: 25px;
margin-bottom: 25px;
}
.item-title {
width: 30%;
padding-left: 15px;
padding-right: 5px;
font-size:20px;
color: darkslategray;
text-align: left;
}
.item-content {
justify-content: center;
text-align: left;
font-size:20px;
color:darkslategray;
}
.input-text {
width: 350px;
font-size:16px;
color:darkgray;
}
.select {/* justify-content: center;*/
text-align: right;
}
.button-container {
width: 100%;
height: 100px;
}
.btn {
width: 100%;
height: 130px;
padding: 10px;
text-align: center;
}
-
代码实现——success页面
跳转注册成功页面后设置一个按钮返回主页面
<!--success.hml-->
<div class="container">
<div class="image-p">
<image class="page-image cover" src="/common/images/jay.png"></image>
</div>
<div class="item-container">
<text class="txt"> {{ $t('Strings.success') }}</text>
<input type="button" class="btn" onclick="onBack" value="{{ $t('Strings.back') }}"/>
</div>
</div>
//js
import router from '@system.router'
export default {
data: {},
onBack() {
router.push({
uri: 'pages/index/index'
})
}
}
/*success.css*/
.container{
flex: 1;
flex-direction: column;
}
.image-p{
width:100%;
position:absolute;
height:100%;
}
.btn {
width: 100px;
height: 50px;
text-align: center;
border-radius: 5px;
margin-right: 60px;
margin-left: 60px;
margin-top: 30px;
margin-bottom: 30px;
color: white;
font-size: 30px;
background-color: #0faeff;
}
.item-container {
width: 100%;
flex: 1;
flex-direction: column;
}
.txt {
margin-top: 100px;
margin-bottom: 100px;
font-size: 50px;
text-align: center;
color: darkslategray;
}
.item-content {
width: 100%;
justify-content: center;
padding-left: 30px;
padding-right: 30px;
}
.button-container {
width: 100%;
height: 150px;
}
.btn {
width: 100%;
height: 50px;
padding: 10px;
text-align: center;
align-items:center;
}
-
实现效果图:
更多推荐
所有评论(0)