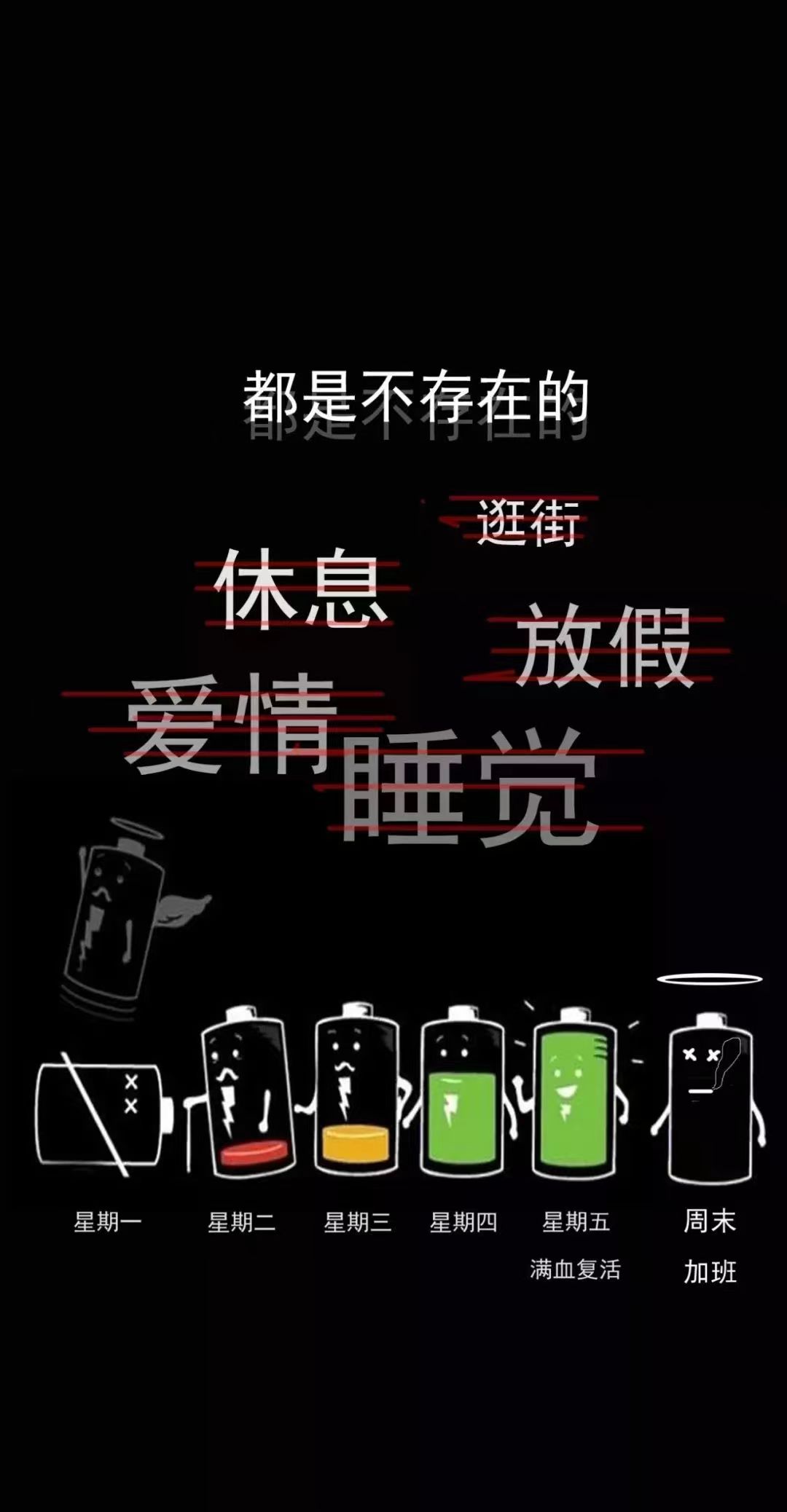
vuex在小程序中的方法
在前面的文章()中,我们提到了,对于vuex在vue2跟vue3的不同,但是呢,并没有提到vuex在小程序中是怎么使用的,这篇文章告诉你,在vuex在小程序中使用的方法。
前言
在前面的文章(vuex的理解)中,我们提到了,对于vuex在vue2跟vue3的不同vuex的理解,但是呢,并没有提到vuex在小程序中是怎么使用的,这篇文章告诉你,在vuex在小程序中使用的方法
1.配置vuex
-
在项目根目录中创建
store
文件夹,专门用来存放 vuex 相关的模块 -
在
store
目录上鼠标右键,选择新建 -> js文件
,新建store.js
文件:
3.在 store.js
中按照如下 4 个步骤初始化 Store 的实例对象:
// 1. 导入 Vue 和 Vuex
import Vue from 'vue'
import Vuex from 'vuex'
// 2. 将 Vuex 安装为 Vue 的插件
Vue.use(Vuex)
// 3. 创建 Store 的实例对象
const store = new Vuex.Store({
// TODO:挂载 store 模块
modules: {},
})
// 4. 向外共享 Store 的实例对象
export default store
4.在 main.js
中导入 store
实例对象并挂载到 Vue 的实例上:
// 1. 导入 store 的实例对象
import store from './store/store.js'
// 省略其它代码...
const app = new Vue({
...App,
// 2. 将 store 挂载到 Vue 实例上
store,
})
app.$mount()
2.创建store模块
这里就 以 创建 购物车的store 为例
-
在
store
目录上鼠标右键,选择新建 -> js文件
,创建购物车的 store 模块,命名为cart.js
: -
在
cart.js
中,初始化如下的 vuex 模块:export default { // 为当前模块开启命名空间 namespaced: true, // 模块的 state 数据 state: () => ({ // 购物车的数组,用来存储购物车中每个商品的信息对象 // 每个商品的信息对象,都包含如下 6 个属性: // { goods_id, goods_name, goods_price, goods_count, goods_small_logo, goods_state } cart: [], }), // 模块的 mutations 方法 mutations: {}, // 模块的 getters 属性 getters: {}, }
-
在
store/store.js
模块中,导入并挂载购物车的 vuex 模块,示例代码如下:import Vue from 'vue' import Vuex from 'vuex' // 1. 导入购物车的 vuex 模块 import moduleCart from './cart.js' Vue.use(Vuex) const store = new Vuex.Store({ // TODO:挂载 store 模块 modules: { // 2. 挂载购物车的 vuex 模块,模块内成员的访问路径被调整为 m_cart,例如: // 购物车模块中 cart 数组的访问路径是 m_cart/cart m_cart: moduleCart, }, }) export default store
3.使用
在 对应的页面里使用已经创建好的store中的数据
这里就以 goods_detail.vue为例
-
在
goods_detail.vue
页面中,修改<script></script>
标签中的代码如下:// 从 vuex 中按需导出 mapState 辅助方法 import { mapState } from 'vuex' export default { computed: { // 调用 mapState 方法,把 m_cart 模块中的 cart 数组映射到当前页面中,作为计算属性来使用 // ...mapState('模块的名称', ['要映射的数据名称1', '要映射的数据名称2']) ...mapState('m_cart', ['cart']), }, // 省略其它代码... }
注意:今后无论映射 mutations 方法,还是 getters 属性,还是 state 中的数据,都需要指定模块的名称,才能进行映射。
-
在页面渲染时,可以直接使用映射过来的数据,例如:
<!-- 运费 --> <view class="yf">快递:免运费 -- {{cart.length}}</view>
注意点:
修改购物车中商品的勾选状态时要注意
第二点: 导入 mapMutations
这个辅助函数要写在methods里
-
在
store/cart.js
模块中,声明如下的mutations
方法,用来修改对应商品的勾选状态:// 更新购物车中商品的勾选状态 updateGoodsState(state, goods) { // 根据 goods_id 查询购物车中对应商品的信息对象 const findResult = state.cart.find(x => x.goods_id === goods.goods_id) // 有对应的商品信息对象 if (findResult) { // 更新对应商品的勾选状态 findResult.goods_state = goods.goods_state // 持久化存储到本地 this.commit('m_cart/saveToStorage') } }
-
在
cart.vue
页面中,导入mapMutations
这个辅助函数,从而将需要的 mutations 方法映射到当前页面中使用:import badgeMix from '@/mixins/tabbar-badge.js' import { mapState, mapMutations } from 'vuex' export default { mixins: [badgeMix], computed: { ...mapState('m_cart', ['cart']), }, data() { return {} }, methods: { ...mapMutations('m_cart', ['updateGoodsState']), // 商品的勾选状态发生了变化 radioChangeHandler(e) { this.updateGoodsState(e) }, }, }
后续 补充
1. js文件 store ----- userInfo.js
export default {
namespaced: true,
state: () => ({
userCity: '' // 地址信息
// userCity: uni.getStorageSync('userCity') || '',
}),
mutations: {
// 保存用户地址信息
updateUserCity(state, obj) {
state.userCity = obj
// 修改用户地址信息
this.commit('m_userInfo/saveCityStorage')
},
saveCityStorage(state) {
uni.setStorageSync('userCity', state.userCity)
}
},
}
2. store.js文件
import Vue from 'vue'
import Vuex from 'vuex'
import userInfo from './userInfo.js'
Vue.use(Vuex)
export default new Vuex.Store({
modules: {
m_userInfo: userInfo
}
})
3. 项目中 需保存vuex中的值 以及修改 保存后的值 也是使用下面的方法
import {
mapMutations
} from 'vuex'
methods: {
...mapMutations('m_userInfo', ['updateUserCity']),
// 获取值后 进行保存 this.updateUserCity(值)
this.updateUserCity(this.city)
}
4. 在页面上显示 值
<template>
// 页面上渲染值
{{userCity}}
</template>
import {
mapState,
mapMutations
} from 'vuex'
computed: {
...mapState('m_userInfo', ['userCity'])
},
methods: {
...mapMutations('m_userInfo', ['updateUserCity']),
this.updateUserCity(item.name)
}
更多推荐
所有评论(0)