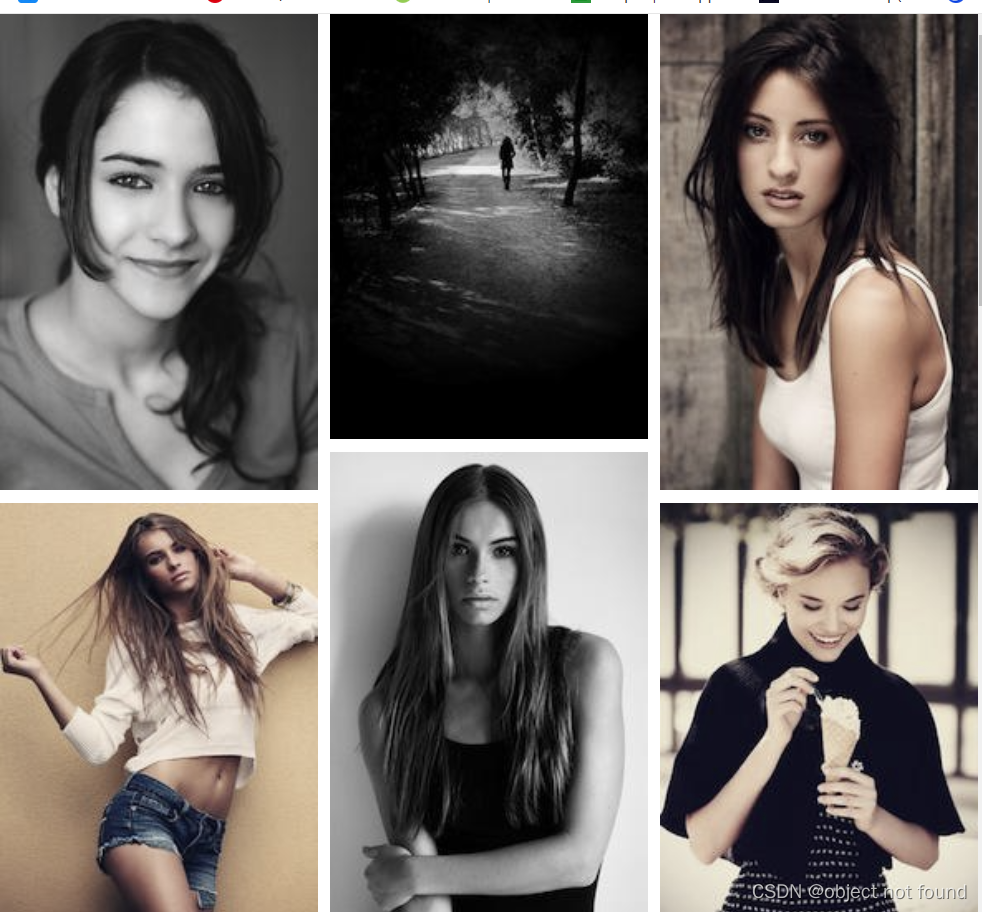
使用js实现响应式瀑布流布局(附带动画效果)
4.进行判断如果i小于列数则将第一列的图片高度添加进数组然后设置style的top,left值控制其位置。5.如果i大于列数就说明是第二行,则找到上一行数组中高度最小的元素,根据其设置top,left值;1.获取图片容器的宽度,根据宽度的大小去控制列数的生成;2.定义间距变量,图片数组和计算出每列宽度;3.遍历图片元素数组,为每一项加上宽度;6.最后根据根据索引将每一项添加到数组。......
效果图
思路
1.获取图片容器的宽度,根据宽度的大小去控制列数的生成;
2.定义间距变量,图片数组和计算出每列宽度;
3.遍历图片元素数组,为每一项加上宽度;
4.进行判断如果i小于列数则将第一列的图片高度添加进数组然后设置style的top,left值控制其位置
5.如果i大于列数就说明是第二行,则找到上一行数组中高度最小的元素,根据其设置top,left值;
6.最后根据根据索引将每一项添加到数组
代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
body {
margin: 0;
}
.wrap {
position: relative;
margin: 10px;
max-width: 1200px;
margin: 0 auto;
}
.box {
position: absolute;
/* 图像灰度 */
filter: grayscale(0.5);
transition: left 0.5s, top 0.5s;
}
.box img {
display: block;
width: 100%;
}
</style>
</head>
<body>
<div class="wrap">
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/001.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/002.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/003.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/004.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/005.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/006.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/007.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/008.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/009.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/010.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/011.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/012.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/013.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/014.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/015.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/016.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/017.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/018.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/019.jpg" alt="" />
</div>
<div class="box">
<img src="http://wlog.cn/demo/waterfall/images/020.jpg" alt="" />
</div>
</div>
<script>
// 根据元素宽度生成列数从而实现响应式
function createColumns(ele) {
let width = ele.offsetWidth;
if (width >= 1200) {
_column = 5;
}
if (width < 1200 && width >= 992) {
_column = 4;
}
if (width < 992 && width >= 768) {
_column = 3;
}
if (width < 768) {
_column = 2;
}
if (width < 360) {
_column = 1;
}
return _column;
}
function render() {
let _wrap = document.querySelector(".wrap"); //父容器
let _column = createColumns(_wrap); //列数
let _spacing = 10; //间距
let _colWidth =
(_wrap.offsetWidth - (_column - 1) * _spacing) / _column; //列宽
let _boxList = document.querySelectorAll(".box");
let _arr = []; //高度数组
for (let i = 0; i < _boxList.length; i++) {
_boxList[i].style.width = _colWidth + "px";
if (i < _column) {
_arr.push(_boxList[i].offsetHeight); //将每一列的高度存放到_arr数组中
_boxList[i].style.top = 0;
_boxList[i].style.left = (_colWidth + _spacing) * i + "px";
} else {
let min = Math.min(..._arr); //最小高度
let index = _arr.indexOf(min); //最小高度的索引
_boxList[i].style.top = min + _spacing + "px"; //第二列居上距离
_boxList[i].style.left = (_spacing + _colWidth) * index + "px"; //第二列居左距离
_arr[index] += _boxList[i].offsetHeight + _spacing;
}
}
}
// 在网页上的资源全部加载完成后再运行代码
window.addEventListener("load", render);
// 窗口宽度改变
window.addEventListener("resize", render);
</script>
</body>
</html>
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
csdn文章推荐受影响解决办法10个字10行
更多推荐
所有评论(0)