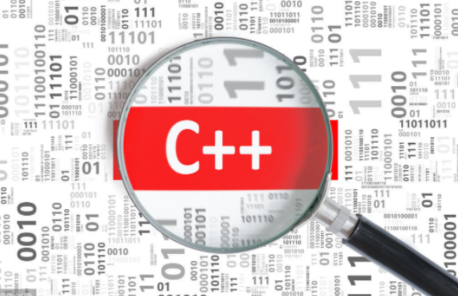
C++11 std::for_each 用法小结
std::for_each相较于for而言,它可以帮助在STL容器中的每个元素都执行一次fn()函数。
·
std::for_each 相较于for而言,它可以帮助在STL容器中的每个元素都执行一次fn()函数。如果你用过 find_first_of 或者 find_last_of,那么现在你要替换所有符合判断表达式的,可以考虑使用for_each了!
来自 https://en.cppreference.com/w/cpp/algorithm/for_each 的demo
#include <vector>
#include <algorithm>
#include <iostream>
struct Sum
{
void operator()(int n) { sum += n; }
int sum{0};
};
int main()
{
std::vector<int> nums{3, 4, 2, 8, 15, 267};
auto print = [](const int& n) { std::cout << " " << n; };
std::cout << "before:";
std::for_each(nums.cbegin(), nums.cend(), print);
std::cout << '\n';
std::for_each(nums.begin(), nums.end(), [](int &n){ n++; });
// calls Sum::operator() for each number
Sum s = std::for_each(nums.begin(), nums.end(), Sum());
std::cout << "after: ";
std::for_each(nums.cbegin(), nums.cend(), print);
std::cout << '\n';
std::cout << "sum: " << s.sum << '\n';
}
示例代码<1> 计算从1加到100的和(1+2+3+…+100=?)
#include <iostream>
#include <vector>
#include <algorithm>
// reference: http://en.cppreference.com/w/cpp/algorithm/for_each
struct Sum
{
Sum() : sum{ 0 } { }
void operator()(int n) { sum += n; }
int sum;
};
int main()
{
//计算从1至100的和
std::vector<int> intArray = {};
for (size_t i = 1; i <= 100; i++)
{
intArray.push_back(i);
}
//calls Sum::operator() for each number
Sum total = std::for_each(intArray.begin(), intArray.end(), Sum());
std::cout << "s=" << total.sum << '\n';
return 0;
}
示例代码<2> 打印每科的分数,计算总分数和平均分
#include <iostream>
#include <vector>
#include <algorithm>
using kv = std::pair<std::string, int>;
void printScore(kv score)
{
std::cout << score.first << "=" << score.second << std::endl;
}
// The function object to determine the average
class Average
{
private:
long num; // The number of elements
long sum; // The sum of the elements
public:
// Constructor initializes the value to multiply by
Average() : num(0), sum(0)
{
}
// The function call to process the next element
void operator ( ) (int elem)
{
num++; // Increment the element count
sum += elem; // Add the value to the partial sum
}
// return Average
operator double()
{
return static_cast<double>(sum) / static_cast<double>(num);
}
};
int main()
{
std::unordered_map<std::string, int> scoreMap =
{
{"语文", 95},
{"数学", 97},
{"英语", 99},
};
//打印每科分数
std::for_each(scoreMap.begin(), scoreMap.end(), printScore);
//计算总分数
int sum = 0;
std::for_each(scoreMap.begin(), scoreMap.end(), [&](kv score)
{
sum += score.second;
});
std::cout << "总分数=" << sum << std::endl;
//
std::vector<int> scores = { 95, 97, 99 };
//
double avg = std::for_each(scores.begin(), scores.end(), Average());
std::cout << "平均分=" << avg << std::endl;
return 0;
}
示例代码<3> 替换字符操作
考虑到 for_each() 可以针对容器里面每个元素做操作。那么假设抖音直播时,要屏蔽“围观群众”发送的不文明用语,那么就可以尝试做关键字屏蔽
#include <iostream>
#include <vector>
#include <unordered_map>
#include <algorithm>
void replaceChar(char& c)
{
if (c == 'f' || c == 'u' || c == 'c' || c == 'k')
c = '*';
}
int main()
{
std::string str = "Fuck, Guangzhou is so hot lately!";
std::cout << "raw string is:" << str << std::endl;
//
std::for_each(str.begin(), str.end(), replaceChar);
std::cout << "after update is:" << str << std::endl;
return 0;
}
更多推荐
所有评论(0)