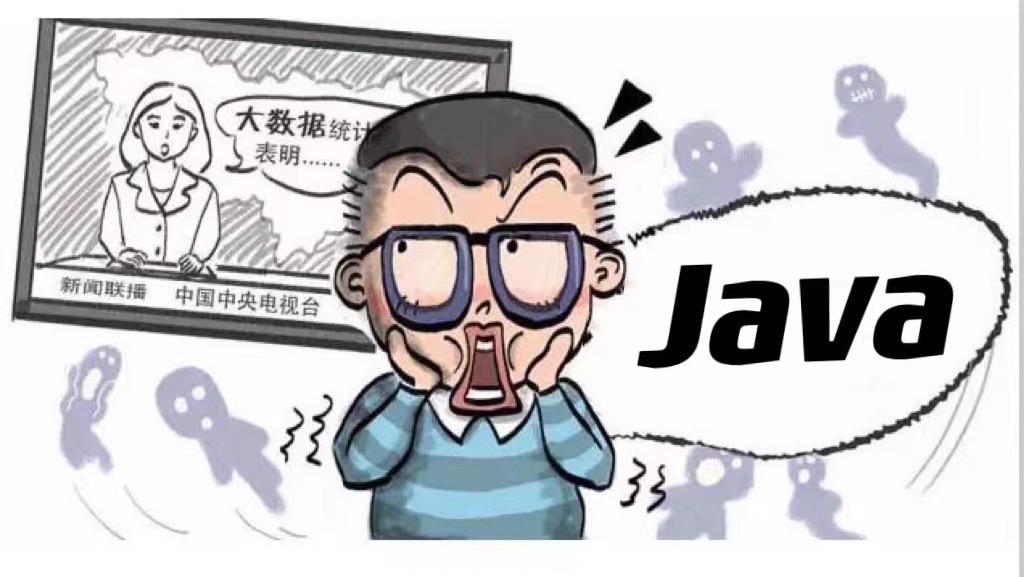
Java基础知识九:Map集合基本介绍、Map集合put()方法、获取Map集合键值元素3种方式,HashMap集合讲解与案例分析,Collections常用3个方法与对ArrayList集合进行排序
Map集合1、Map集合:添加方法:put( )//Map的基本使用public classmapDemo1{public static voidmain(String[]args) {//Map集合中键、值对应//put()方法添加元素// 键有重复时,put()方法会把后面的值替换前面的值Map<String,String>map=newHashMap<>();...

Map集合
1、Map集合:添加方法:put( )
//Map的基本使用
public class mapDemo1 {
public static void main(String[] args) {
//Map集合中键、值对应
//put()方法添加元素
// 键有重复时,put()方法会把后面的值替换前面的值
Map<String,String> map = new HashMap<>();
map.put("itheima001","java");
map.put("itheima002","python");
map.put("itheima003","mysql");
map.put("itheima004","oracle");
map.put("itheima004","hadoop");
System.out.println(map);
}
}
2、Map集合基本功能介绍
//Map基本方法的使用
public class mapDemo2 {
public static void main(String[] args) {
Map<String,String> map = new HashMap<String,String>();
map.put("浙江省","杭州市");
map.put("江苏省","南京市");
map.put("湖南省","长沙市");
map.put("广东省","深圳市");
map.remove("江苏省"); //根据key删除元素
map.clear(); //清除Map集合中所有的键值元素
boolean blg1 = map.containsKey("湖南省"); //判断集合中是否包含有对应的key
System.out.println(blg1);
boolean blg2 = map.containsValue("杭州市");//判断集合中是否包含有对应的Value
System.out.println(blg2);
boolean blg3 = map.isEmpty(); //判断集合是否为空
System.out.println(blg3);
int num = map.size(); //判断集合的长度
System.out.println(num);
System.out.println(map);
}
}
3、获取Map集合键、值元素的3个方法
//Map集合获取的三个方法
public class mapDemo3 {
public static void main(String[] args) {
Map<String,String> map = new HashMap<>();
map.put("浙江省","杭州市");
map.put("江苏省","南京市");
map.put("湖南省","长沙市");
map.put("广东省","深圳市");
System.out.println(map.get("湖南省"));//获取该Key对应的Value
System.out.println("------------------");
Set<String> set = map.keySet(); //获取所有Key的集合
for(String key: set){
System.out.println(key);
}
System.out.println("------------------");
Collection<String> coll = map.values();//获取所有Value的集合
for(String value: coll){
System.out.println(value);
}
}
4、遍历Map集合方式一
//Map集合遍历方式一
public class mapDemo4 {
public static void main(String[] args) {
Map<String,String> map = new HashMap<>();
map.put("浙江省","杭州市");
map.put("江苏省","南京市");
map.put("湖南省","长沙市");
map.put("广东省","深圳市");
Set<String> key = map.keySet();//获取所有键的集合
//遍历键的集合
for(String keys: key){
String value = map.get(keys); //根据Key来找到对应的Value
System.out.println(value);
}
}
5、遍历Map集合方式二
//遍历Map集合中的Key、Value
//entrySet()下有getKey()与getValue()两个方法可以分别获取到Map集合中的Key、Value
public class mapDemo4 {
public static void main(String[] args) {
Map<String,String> map = new HashMap<>();
map.put("浙江省","杭州市");
map.put("江苏省","南京市");
map.put("湖南省","长沙市");
map.put("广东省","深圳市");
Set<Map.Entry<String, String>> entrySet = map.entrySet();
for(Map.Entry<String, String> me: entrySet){
String key = me.getKey();
String value = me.getValue();
System.out.println(key+","+value);
}
}
6、案例一:创建HashMap集合,遍历学生类对象
//案例:创建集合,遍历学生类对象
public class HashMapDemo1 {
public static void main(String[] args) {
HashMap<String, Student> hs = new HashMap<>();
Student stu1 = new Student("深圳",21);
Student stu2 = new Student("北京",32);
Student stu3 = new Student("北京",43);
Student stu4 = new Student("西安",54);
hs.put("itheima001",stu1);
hs.put("itheima002",stu2);
hs.put("itheima003",stu3);
hs.put("itheima004",stu4);
//第一种方式:通过Key找到值
Set<String> keyset = hs.keySet();//获取所有Key的集合
for(String key: keyset){
Student value = hs.get(key);//根据Key来找到对应的Value
System.out.println(value.getName()+","+value.getAge());
}
//第二种方式:键值对对象找到Key和Value
Set<Map.Entry<String, Student>> entrySet = hs.entrySet();
for(Map.Entry<String, Student> me: entrySet){
String key = me.getKey();
Student value = me.getValue();
System.out.println(value.getName()+","+value.getAge());
}
}
}
7、案例二:遍历HashMap集合,键为Student对象、值为String类型
/*案例:HashMap集合存储学生对象,并遍历
* 创建一个HashMap集合,键是学生对象(Student),值是居住地(String),存储多个键值对元素,并遍历。
* 要求保证键的唯一性:如果学生对象的成员变量值相同,就默认是同一个学生对象。
* */
public class HashMapDemp2 {
public static void main(String[] args) {
HashMap<Student,String> hm = new HashMap<>();
Student stu1 = new Student("深圳",22 );
Student stu2 = new Student("杭州",33 );
Student stu3 = new Student("郑州",44 );
Student stu4 = new Student("昆明",55 );
//有两个相同的对象时,需要在Student中重写equals与hashCode方法,保证键的唯一性
Student stu5 = new Student("昆明",55 );
hm.put(stu1,"深圳市福田区");
hm.put(stu2,"杭州市西湖区");
hm.put(stu3,"郑州市新郑区");
hm.put(stu4,"昆明市高新区");
hm.put(stu5,"丽江市新江区");
Set<Student> studentSet = hm.keySet();//获取所有Key的集合
for(Student key: studentSet){
String value = hm.get(key);//根据Key来找到对应的Value
System.out.println(key.getName()+","+key.getAge()+value);
}
}
}
8、案例三:遍历HashMap集合,键、值都是String类型
/*ArrayList集合中存储三个HashMap集合元素
每个HashMap的Key和Value都是String类型
最后遍历ArrayList集合
*/
public class ArrayListAndHashMap {
public static void main(String[] args) {
ArrayList<HashMap<String,String>> al = new ArrayList<HashMap<String, String>>();
HashMap<String,String> hm1 = new HashMap<String,String>();
hm1.put("北京","海淀区");
hm1.put("深圳","罗湖区");
hm1.put("上海","青浦区");
al.add(hm1);
HashMap<String,String> hm2 = new HashMap<String,String>();
hm2.put("设计","小王");
hm2.put("编辑","小赵");
hm2.put("运营","小李");
al.add(hm2);
HashMap<String,String> hm3 = new HashMap<String,String>();
hm3.put("数学","刘老师");
hm3.put("英语","孙老师");
hm3.put("历史","陈老师");
al.add(hm3);
for(HashMap<String,String> hm: al){
Set<String> keySet = hm.keySet();
for(String key: keySet){
String value = hm.get(key);
System.out.println(key+","+value);
}
}
}
}
9、案例四:遍历HashMap集合,键String、值ArrayList集合
/*案例:创建一个HashMap集合,存储三个键值对元素
每一个键值对元素的键是String类型,值是ArrayList集合
每一个ArrayList是String类型,最后遍历HashMap集合
* */
public class HashMapAndArrarList {
public static void main(String[] args) {
HashMap<String, ArrayList<String>> hm = new HashMap<>();
ArrayList<String> arr1 = new ArrayList<>();
arr1.add("语文");
arr1.add("英语");
arr1.add("体育");
hm.put("小学",arr1);
ArrayList<String> arr2 = new ArrayList<>();
arr2.add("语文");
arr2.add("英语");
arr2.add("体育");
hm.put("初中",arr2);
ArrayList<String> arr3 = new ArrayList<>();
arr3.add("语文");
arr3.add("英语");
arr3.add("体育");
hm.put("高中",arr3);
Set<String> keySet = hm.keySet();
for(String key: keySet){
System.out.println(key);
ArrayList<String> al = hm.get(key);
for(String value: al){
System.out.println("\t"+value);
}
}
}
}
10、案例:统计每个字母出现的次数
//案例:键盘随机录入一串字母,统计每个字母出现的次数
//结果:a(5)b(6)c(8)...
public class HashMapAndTreeMap {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入一串字母:");
String line = sc.nextLine();
//HashMap<Character,Integer> hm = new HashMap<>(); //无排序
TreeMap<Character,Integer> hm = new TreeMap<>(); //有排序 ,对键进行排序
//遍历字符串得到每个一字符
for(int i=0;i<line.length();i++){
char key = line.charAt(i);//根据索引就找到了每个值,这个值是做为key存在的
//拿到每一个字符做为键,去HashMap中找对应的值,看其返回值
Integer value = hm.get(key);//key是基本类型,这里有一个自动装箱的动作
if(value==null){
//如果返回值是null,说明该字符在HashMap中不存在,就把该字符做为键,1做为值存储
hm.put(key,1);//这里也有一个自动装箱的动作
}else{
value++; //这里有一个自动拆箱的动作
hm.put(key,value);//这里要重新存储一下这个字符和value++之后的值
}
}
//遍历HashMap集合,得到键和值,按要求进行拼接
StringBuilder sb = new StringBuilder();
Set<Character> keySet = hm.keySet();
for(Character key: keySet){
Integer value = hm.get(key);
sb.append(key).append("(").append(value).append(")");//拼接后是StringBuilder类型
}
String result = sb.toString();//StringBuilder类型进行一下转换成需要的结果
System.out.println(result); //输入结果
}
}
11、Collections: 基本方法使用
/*Collections常用的三个方法:
sort():将指定的元素进行升序排序
reverse():反转指定列表中元素的顺序
shuffle():随机排列指定元素列表
*/
public class collections {
public static void main(String[] args) {
List<Integer> list = new LinkedList<>();
list.add(20);
list.add(30);
list.add(50);
list.add(10);
list.add(60);
list.add(40);
Collections.sort(list);//将指定的元素进行升序排序
Collections.reverse(list);//反转指定列表中元素的顺序
Collections.shuffle(list);//随机排列指定元素列表
System.out.println(list);
}
}
12、Collections对ArrayList集合进行排序
//Collections案例一:
public class CollectionDemo2 {
public static void main(String[] args) {
ArrayList<Student> al = new ArrayList<>();
Student stu1 = new Student("北京",21 );
Student stu2 = new Student("深圳",32 );
Student stu3 = new Student("上海",96 );
Student stu4 = new Student("西安", 58);
Student stu5 = new Student("杭州",72 );
al.add(stu1);
al.add(stu2);
al.add(stu3);
al.add(stu4);
al.add(stu5);
//sort():将指定的元素进行升序排序
//采用比较器的方式
Collections.sort(al, new Comparator<Student>() {
@Override
public int compare(Student s1, Student s2) {
int num = s1.getAge()-s2.getAge();
int num2 = num==0?s1.getName().compareTo(s2.getName()):num;
return num2;
}
});
for(Student s: al){
System.out.println(s.getName()+","+s.getAge());
}
}
}
更多推荐
所有评论(0)