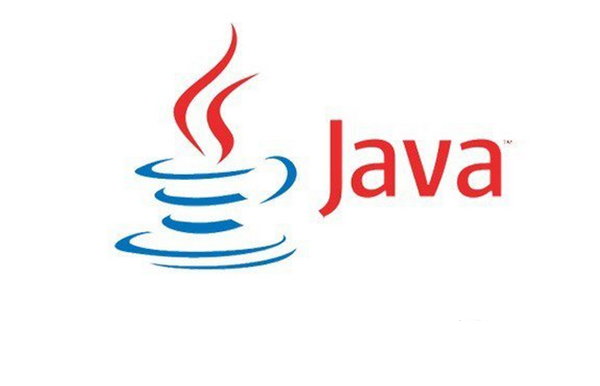
复合yaml文件转成json列表操作
背景k8s中 如果一个yaml文件包含多种资源类型 使用kubectl apply -f xx.yaml 可以批量创建现在需要通过web json消息方式支持此种类型的yaml文件注入到k8s中查了相关资料 都是 yaml文件中只包含一种资源类型处理方法需要自己封装 根据此类yaml的特点 先进行分割依次进行yaml转jsonstring返回jsonString 列表yaml样例---json:-
·
背景
k8s中 如果一个yaml文件包含多种资源类型 使用kubectl apply -f xx.yaml 可以批量创建
现在需要通过web json消息方式支持此种类型的yaml文件注入到k8s中
查了相关资料 都是 yaml文件中只包含一种资源类型
处理方法
需要自己封装 根据此类yaml的特点 先进行分割
依次进行yaml转jsonstring
返回jsonString 列表
yaml样例
---
json:
- rigid
- better for data interchange
yaml:
- slim and flexible
- better for configuration
object:
key: value
array:
- null_value:
- boolean: true
- integer: 1
- alias: &example aliases are like variables
- alias: *example
paragraph: >
Blank lines denote
paragraph breaks
content: |-
Or we
can auto
convert line breaks
to save space
alias: &foo
bar: baz
alias_reuse: *foo
---
json:
- rigid
- better for data interchange
yaml:
- slim and flexible
- better for configuration
object:
key: value
array:
- null_value:
- boolean: true
- integer: 1
- alias: &example aliases are like variables
- alias: *example
paragraph: >
Blank lines denote
paragraph breaks
content: |-
Or we
can auto
convert line breaks
to save space
alias: &foo
bar: baz
alias_reuse: *foo
代码样例
相关库
<!-- https://mvnrepository.com/artifact/com.fasterxml.jackson.dataformat/jackson-dataformat-yaml -->
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-yaml</artifactId>
<version>2.13.2</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.13.2</version>
</dependency>
package utils;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.yaml.YAMLFactory;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Pattern;
public class YamlUtils {
static String regex = "^---";
static Pattern p = Pattern.compile(regex);
public static List<String> YamlToJson(String filePath) throws IOException {
File file = new File(filePath);
return YamlToJson(file);
}
public static List<String> YamlToJson(File file) throws IOException {
FileInputStream fs = new FileInputStream(file);
BufferedInputStream bs = new BufferedInputStream(fs);
return YamlToJson(bs);
}
public static List<String> YamlToJson(InputStream inputStream) throws IOException {
List<String> result = new ArrayList<>();
BufferedReader bs = new BufferedReader(new InputStreamReader(inputStream));
String str = null;
StringBuffer yamlStr = new StringBuffer();
while ((str = bs.readLine())!= null){
if (p.matcher(str).find()){
if (yamlStr.toString().length() != 0){
result.add(convertYamlToJson(yamlStr.toString()));
}
yamlStr = new StringBuffer();
}else {
yamlStr.append(str).append("\n");
}
}
if (yamlStr.toString().length() != 0){
result.add(convertYamlToJson(yamlStr.toString()));
}
return result;
}
public static String convertYamlToJson(String yaml) throws IOException {
ObjectMapper yamlReader = new ObjectMapper(new YAMLFactory());
Object obj = yamlReader.readValue(yaml, Object.class);
ObjectMapper jsonWriter = new ObjectMapper();
return jsonWriter.writeValueAsString(obj);
}
}
如果问题 请多指教
更多推荐
所有评论(0)