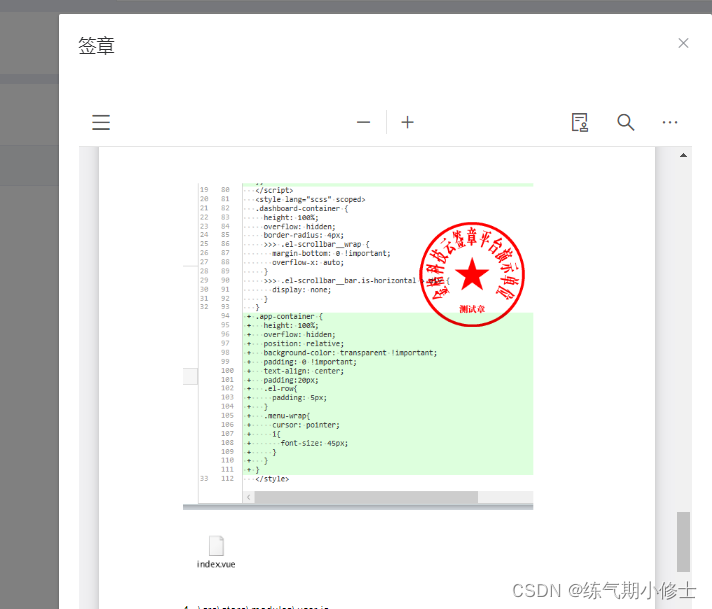
金格集成(springboot+vue)
一.配置相关iSignature_Cloud_API.lic:认证文件,这个应该是有过期时间的。过期后可能会显示中间件授权相关提示。Mypdffileresource.java:关键实现类,金格操作会调用此处的方法进行操作。pdfviewer.properties:这个地方里面有个跨域配置可能需要注意在本地调试时,需配置上本地地址:2. 如果你集成服务,他们会提供一个jsp后端项目。基本上像什么服
一.配置相关
iSignature_Cloud_API.lic:
认证文件,这个应该是有过期时间的。过期后可能会显示中间件授权相关提示。
Mypdffileresource.java:
关键实现类,金格操作会调用此处的方法进行操作。
pdfviewer.properties:
这个地方里面有个跨域配置可能需要注意在本地调试时,需配置上本地地址:
2. 如果你集成服务,他们会提供一个jsp后端项目。基本上像什么服务器之类的基本信息都给你了,需要对应改下位置就好,配置文件移动到和springboot的resource下
contorller
@Autowired
private MyPdfFileResource myPdfFileResource;
@RequestMapping(value = "/jingePdf",method = {RequestMethod.GET,RequestMethod.POST})
@ApiOperation("金格请求入口")
public void jingePdf(HttpServletRequest request, HttpServletResponse response) throws IOException, DataException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String contentType = request.getContentType();
try {
PdfViewer.execute(request, response,myPdfFileResource);
} catch (Throwable e) {
if (ReqUtil.isStartWithApplicationJson(contentType)) {
JSONObject obj = new JSONObject();
obj.put("status", false);
obj.put("message", "程序异常,请联系管理员!");
response.getWriter().write(obj.toJSONString());
}
}
}
对于spring可以自动注入MyPdfFileResource.
3.前端
前端部分让他们提供一个vue项目。他们有vue的示例
我们要关注的是如何传参数。
<template>
<div class="UploadFile-container">
<template v-if="filePath && filePath.length > 0">
<el-button
type="danger"
icon="el-icon-s-check"
circle
@click="showSignaturePage"
></el-button>
<el-button
v-if="this.formData.signatureDownLoadUrl"
type="success"
icon="el-icon-download"
circle
@click="download"
></el-button>
</template>
<el-dialog title="签章" :visible.sync="dialogFormVisible" append-to-body>
<iframe
id="jingeIframe"
class="App-iframe"
ref="iframe"
:title="title"
src="./pdfjs/viewer.html"
@load="setConfig"
></iframe>
<div slot="footer" class="dialog-footer">
<el-button type="primary" @click="closeDialog">关闭</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
const units = {
KB: 1024,
MB: 1024 * 1024,
GB: 1024 * 1024 * 1024
};
import { getDownloadUrl } from "@/api/common";
import { PreviewUrl, EditUrl } from "@/api/extend/documentPreview";
export default {
name: "UploadYozoFile",
props: {
value: {
type: String,
default: ""
},
},
data() {
return {
name: "file",
filePath: "",
dialogFormVisible: false,
previewUrl: undefined,
fileTitle:undefined,
formData: {
appType: "1",
previewUrl: undefined,
editUrl: undefined,
signatureDownLoadUrl: undefined,
signaturePreviewUrl: undefined
},
title: "金格云阅读",
iframeData: null,
nowShow: "",
};
},
methods: {
closeDialog() {
this.dialogFormVisible = false;
},
open(documentId) {
const KGPdfViewerWebApp = this.iframeData.KGPdfViewerWebApp;
KGPdfViewerWebApp.open(documentId);
},
stamp() {
const KGPdfViewerWebApp = this.iframeData.KGPdfViewerWebApp;
KGPdfViewerWebApp.stamp();
},
stampOfText(text) {
const KGPdfViewerWebApp = this.iframeData.KGPdfViewerWebApp;
KGPdfViewerWebApp.stampOfText(
`1-${KGPdfViewerWebApp.PDFViewerApplication.pagesCount}`,
text
);
},
stampOfXY(left, top) {
const KGPdfViewerWebApp = this.iframeData.KGPdfViewerWebApp;
KGPdfViewerWebApp.stampOfXY(
`1-${KGPdfViewerWebApp.PDFViewerApplication.pagesCount}`,
left,
top
);
},
setConfig() {
const KGPdfViewerWebApp = this.iframeData.KGPdfViewerWebApp;
KGPdfViewerWebApp.setUrl(this.define.jinge);
// 这里很关键配置的就是后端接口的地址,如 localhost:8080/api/file/jingePdf
KGPdfViewerWebApp.userId = "T001";
KGPdfViewerWebApp.sealType = "PT";
KGPdfViewerWebApp.stampType = 0;
KGPdfViewerWebApp.sealOrigin = 0;
KGPdfViewerWebApp.certOrigin = 0;
KGPdfViewerWebApp.fileType = "PDF";
KGPdfViewerWebApp.stampSuccess = res => {
let result = JSON.parse(res);
this.formData.signaturePreviewUrl = result.signaturePreviewUrl;
this.formData.signatureDownLoadUrl = result.signatureDownLoadUrl;
let data = JSON.stringify({
...this.formData,
signaturePreviewUrl: result.signaturePreviewUrl,
signatureDownLoadUrl: result.signatureDownLoadUrl
});
this.$emit("input", data);
this.$emit("change", data);
};
KGPdfViewerWebApp.deleteSealSuccess = () => {};
let pdf = this.formData.signatureDownLoadUrl;
// 拼接文件名
pdf = pdf + "##" + this.fileTitle;
console.log(pdf);
this.open(pdf);
},
download() {
if (!this.formData.signatureDownLoadUrl) return;
window.location.href = this.formData.signatureDownLoadUrl;
},
solveUrl() {
return new Promise((resolve, reject) => {
if (!this.filePath) return;
PreviewUrl({ urlRelativePath: this.filePath,convert:true }).then(res => {
this.previewUrl = res.data.viewUrl;
this.fileTitle = res.data.title;
resolve(res.data.viewUrl);
});
}).catch(err => {
reject(err);
});
},
showSignaturePage() {
// 如果signaturpreviewurl为空,使用filepath获取可盖章地址
this.dialogFormVisible = true;
this.$nextTick(async () => {
if (!this.iframeData) {
this.iframeData = this.$refs.iframe.contentWindow;
}
await this.initSignature();
let pdf = this.formData.signatureDownLoadUrl;
// 拼接文件名
this.nowShow = pdf + "##" + this.fileTitle;
// this.open(this.nowShow);
});
},
// 初始化盖章地址
async initSignature() {
return new Promise(async (resolve, reject)=>{
if (!this.formData.signaturePreviewUrl) {
this.formData.signaturePreviewUrl = await this.solveUrl();
}
this.formData.signatureDownLoadUrl = this.formData.signaturePreviewUrl.replaceAll(
"fcscloud/view/preview",
"fcscloud/view/download"
);
resolve()
})
},
}
};
</script>
<style scoped lang="scss">
>>> .el-button {
margin: 5px;
}
.down-wrap {
text-align: center;
}
.App-iframe {
width: 100%;
height: 600px;
border: none;
}
.App-button-group {
display: flex;
width: 100vw;
justify-content: space-around;
margin: 0 auto;
}
.App-button {
width: 120px;
height: 32px;
background: #fff;
outline: none;
border: none;
border-radius: 4px;
padding: 0;
margin-bottom: 24px;
cursor: pointer;
}
</style>
代码只是说明使用,结合具体业务封装组件。主要是通过this.open方法传,试过了这个地方没法使用对象传多个参数,看了下源码发现他就是一个string。所以要this.open只能传一个字符串。要么用拼接传过去,要么用json字符串传过去,这里简单使用拼接方式。
后端对应会填充到documentid.具体处理的话你们需要根据自己的规则写。
这是我的,可以参考。
package jnpf.base;
import cn.hutool.http.HttpRequest;
import cn.hutool.http.HttpResponse;
import cn.hutool.http.HttpUtil;
import com.alibaba.fastjson.JSONObject;
import com.kinggrid.pdfviewer.PdfFileResource;
import jnpf.emnus.YzFileApi;
import jnpf.file.FileApi;
import jnpf.model.YozoFileContext;
import jnpf.model.YozoFileOperator;
import jnpf.model.YozoFileParams;
import jnpf.util.JsonUtil;
import jnpf.util.YozoUtils;
import lombok.extern.slf4j.Slf4j;
import net.sf.ehcache.Cache;
import net.sf.ehcache.Element;
import org.apache.commons.io.IOUtils;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import java.io.*;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.*;
/**
* 该接口实现为多实例,可以设置成员变量
*
* 方法执行顺序
* 1、getPdfFile
* 2、getPdfFileStream
* 3、getSavePdfFileSteam
* 4、getDocumentName
* 5、success
* @author Administrator
*/
@Slf4j
@Component
public class MyPdfFileResource extends PdfFileResource {
// private final static Logger LOGGER = LoggerFactory.getLogger(MyPdfFileResource.class);
//
@Autowired
private FileApi fileApi;
@Autowired
private YozoFileOperator yozoFileOperator;
@Value("${config.YozoOwnPreview}")
private String yozoOwnPreview;
private Cache keyModeCache=null;
// 上传后返回的信息
private Map<String, String> result;
// 临时保存的文件路径
private String tmpFile;
{
keyModeCache=BaseCacheImpl.cacheManager.getCache("keyModeCache");
}
/**
* 返回需要盖章或查看PDF:文件模式
*/
@Override
public String getPdfFile() {
// 如果文件流模式,该接口返回null
// Tomcat 6 及以下版本
// request.getSession().getServletContext().getRealPath("/files/" + documentId);
// return request.getServletContext().getRealPath("/files/" + documentId);
String fileName = fileApi.getPath("temporary")+"1.pdf";
// return fileName;
return null;
}
/**
* 返回需要盖章或查看PDF:文件流模式
* 远程文件下载到本地的temp.pdf
* @return
*/
@Override
public InputStream getPdfFileStream() {
String fileName = fileApi.getPath("temporary")+"temp.pdf";
FileInputStream fileInputStream=null;
// 获取转化地址
String[] split = documentId.split("##");
String fileUrl= split[0];
try {
FileOutputStream fos = new FileOutputStream(fileName);
long size = HttpUtil.download(fileUrl, fos,true);
fileInputStream = new FileInputStream(fileName);
} catch (IOException e) {
e.printStackTrace();
}
return fileInputStream;
}
/**
* 盖章后文件保存
*/
@Override
public OutputStream getSavePdfFileSteam() {
// String dir = request.getServletContext().getRealPath("/files");
String dir = fileApi.getPath("temporary");
// tmpFile = dir + UUID.randomUUID().toString() + File.separator + documentId;
String[] split = documentId.split("##");
tmpFile = dir + split[1] ;
FileOutputStream fileOutputStream;
try {
fileOutputStream = new FileOutputStream(tmpFile);
} catch (FileNotFoundException e) {
throw new RuntimeException(e.getMessage(),e);
}
return fileOutputStream;
}
/**
* 文档名称,记录日志时会使用
*/
@Override
public String getDocumentName(){
return "document.pdf";
}
/**
* 加盖、删除印章等操作成功会执行该方法
* 1、可以实现覆盖原文档
* 2、删除原文档等
*/
@Override
public void success() {
// log.info("success action = " + action);
// log.info("success"+action);
// 覆盖盖章后的文档
if(tmpFile != null){
saveFile();
}
}
/**
* 加盖、删除印章等操作失败时,删除临时文件
*/
@Override
public void fail() {
// log.info("fail action = " + action);
if(tmpFile != null){
File file = new File(tmpFile);
if(file.exists())file.delete();
}
}
/**
* 覆盖原文档 ,上传远程,删除下载回来的文件
*/
private void saveFile(){
// 原文档路径
// String fileName = dir + File.separator + documentId;
FileOutputStream fileOutputStream = null;
FileInputStream in = null;
File file = new File(tmpFile);
String url = yozoOwnPreview + YzFileApi.UPLOAD_PREVIEW.getMethod();
String resultString = YozoUtils.submitPost(url, file);
Map<String, Object> maps = JSONObject.parseObject(resultString, Map.class);
result = (Map<String, String>) maps.get("data");
if(file.exists()) {
file.delete();
}
// 删除临时文件
String fileName = fileApi.getPath("temporary")+"temp.pdf";
File file2 = new File(fileName);
if(file2.exists()) {
file2.delete();
}
// 本地复制文件的形式
// try{
// in = new FileInputStream(tmpFile);
// fileOutputStream = new FileOutputStream(fileName);
// IOUtils.copy(in, fileOutputStream);
// } catch (IOException e) {
// throw new RuntimeException(e.getMessage(), e);
// } finally {
// IOUtils.closeQuietly(in);
// IOUtils.closeQuietly(fileOutputStream);
// if(file.exists()) file.delete();
// }
}
/**
* 域签名、电子签章,密钥盘签名时会先执行该方法,
* 1、实现保存盖章未签名的文档
* 2、签名完成后,需要在未签名的文档上(通过getPrePdfFile)加上签名值,覆盖该文档
* 3、再执行success
*/
@Override
public void preSuccess(){
// 集成时建议使用缓存机制(如:ehcache、redis等), 超过30秒删除该缓存
keyModeCache.put(new Element(documentId, tmpFile));
}
@Override
public String getPrePdfFile(){
tmpFile = keyModeCache.get(documentId).getObjectValue().toString();
keyModeCache.remove(documentId);
return tmpFile;
}
@Override
public List<String> getGBEsids() {
List<String> list = new ArrayList<String>();
list.add("36010000000624");
return list;
}
@Override
public String getSuccessPassBackParameter() {
Map data = new HashMap();
// 转化获取预览地址
YozoFileParams yozoFileParams = new YozoFileParams();
yozoFileParams.setAppType("1");
JSONObject jsonObject = null;
YozoFileContext fileOperator = yozoFileOperator.getFileOperator(yozoFileParams);
try {
String viewUrl = result.get("data");
yozoFileParams.setUrlRelativePath(viewUrl);
HashMap<String, String> resultData = fileOperator.previewUrl(yozoFileParams);
String signaturePreviewUrl = resultData.get("viewUrl");
String downloadUrl = signaturePreviewUrl.replace("fcscloud/view/preview","fcscloud/view/download");
data.put("signaturePreviewUrl",signaturePreviewUrl);
data.put("signatureDownLoadUrl",downloadUrl);
jsonObject = new JSONObject(data);
} catch (IOException e) {
e.printStackTrace();
}
return jsonObject.toString();
}
}
效果
注意:
1.要把jsp的jar打包到自己的后端哪里。请使用maven的,现在我想应该没人还是直接用jar包直接丢的吧,怎么把jar打包一个maven库,请参考如下文章。
将多个jar打包成一个jar_练气期小修士的博客-CSDN博客
2.使用前端的seturl其实就是写自己后端的服务地址。
类似这样的,localhost:8080/api/file/jingePdf 具体就是要访问在pdf接口即可
更多推荐
所有评论(0)