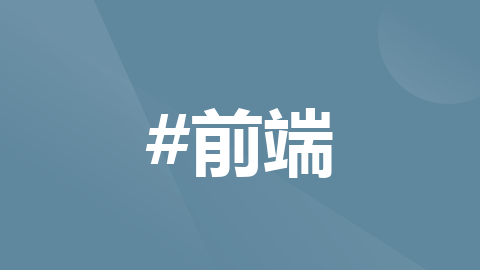
针对Avue、ElementUI框架的表格树,全选多选勾选子节点的问题完善
众所周知,ElementUi的表格树只有单层级的单选多选的功能,树形父子关系不相互关联,那么如何实现表格树选中时父子关系上下关联呢,下面上代码。(注:本代码是基于avue框架的,所以代码结构上是avue风格,不过多选功能ElementUI是完全适用的,毕竟avue就是ElementUI基础上产出的)用到了这两个方法@select=“select”@select-all=“selectAll”<
·
众所周知,ElementUi的表格树只有单层级的单选多选的功能,树形父子关系不相互关联,那么如何实现表格树选中时父子关系上下关联呢,下面上代码。(注:本代码是基于avue框架的,所以代码结构上是avue风格,不过多选功能ElementUI是完全适用的,毕竟avue就是ElementUI基础上产出的)
用到了这两个方法
@select=“select”
@select-all=“selectAll”
<template>
<div>
<basic-container>
<avue-crud
:option="option"
:table-loading="loading"
:data="data"
v-model="form"
ref="crud"
@search-change="searchChange"
@search-reset="searchReset"
@current-change="currentChange"
@selection-change="selectionChange"
@refresh-change="refreshChange"
@on-load="onLoad"
@tree-load="treeLoad"
class="HautoTable"
@select="select"
@select-all="selectAll"
>
</avue-crud>
</basic-container>
</div>
</template>
<script>
export default {
data() {
return {
query: {},
loading: true,
parentId: 0,
selectionList: [],
option: {
labelWidth: 150,
index: false,
lazy: true,
rowKey: "id",
defaultExpandAll: false,
searchShow: false,
searchMenuSpan: 5,
border: true,
viewBtn: false,
dialogClickModal: false,
menu: false,
searchSpan: 6,
searchGutter: 0,
searchLabelWidth: 100,
selection: true,
reserveSelection: true,
addBtn: false,
delBtn: false,
editBtn: false,
indexLabel: "序号",
indexWidth: 80,
columnBtn: false,
refreshBtn: true,
saveBtn: false,
highlightCurrentRow: true,
span: 12,
column: [
{
label: "部门简称",
prop: "deptName",
search: true,
},
{
label: "部门全称",
prop: "fullName",
search: true,
},
{
label: "部门编码",
prop: 'deptCode',
search: true,
width: 80,
}
]
},
data: []
};
},
methods: {
searchReset() {
this.query = {};
this.onLoad(this.page);
},
searchChange(params, done) {
this.query = params;
this.onLoad(params);
done();
},
selectionClear() {
this.selectionList = [];
this.$refs.crud.toggleSelection();
},
currentChange(currentPage) {
this.page.currentPage = currentPage;
},
refreshChange() {
this.onLoad(this.query);
},
onLoad(params = {}) {
this.loading = true;
getTreeList(this.parentId, Object.assign(params, this.query)).then(res => {
if (res.data && res.data.data && res.data.data.length > 0) {
this.data = res.data.data;
this.loading = false;
this.selectionClear();
} else {
this.loading = false;
this.data = []
}
})
},
// 下面是表格树动态点击多选取消方法-----------------------------------
// 设置子级选中/取消
setChildren(children, type) {
// 编辑多个子层级
children.map(j => {
this.toggleSelection(j, type)
if (j.children) {
this.setChildren(j.children, type)
}
})
},
// 设置父级选中/取消
setParent(currentRow, type, parent, selectionLists) {
if (!parent.length) {
parent = this.data
}
let allSelect = []
parent.forEach(item => {
if (item.children) {
// 注:ancestors是当前选中节点的所有父节点的id,是一个字符串形式的数据,这个很关键
if (currentRow.ancestors.indexOf(item.id) != -1) {
// 选中
if (type) {
selectionLists.forEach(k => {
item.children.forEach(j => {
if(k.id == j.id) {
allSelect.push(j)
}
})
})
if (allSelect.length == item.children.length) {
this.toggleSelection(item, type)
selectionLists.push(item)
this.select(selectionLists,item)
} else {
this.setParent(currentRow, type, item.children, selectionLists)
}
} else {
// 取消选中
this.toggleSelection(item, type)
this.setParent(currentRow, type, item.children)
}
}
}
})
},
// 选中父节点时,子节点一起选中取消
select(selection, row) {
const hasSelect = selection.some(el => {
return row.id === el.id
})
if (hasSelect) {
if (row.children) {
// 解决子组件没有被勾选到
this.setChildren(row.children, true)
}
// 子级选中, 如果当前的同级都选中了,则父级就选中了(目前只做到父级选中,爷爷级选不中)
this.setParent(row, true, [], selection)
} else {
if (row.children) {
this.setChildren(row.children, false)
}
// 子级取消选中, 传入当前选中节点, 所有父级取消选中
this.setParent(row, false, [])
}
},
toggleSelection(row, select) {
if (row) {
this.$nextTick(() => {
this.$refs.crud &&
this.$refs.crud.toggleRowSelection(row, select)
}
)
}
},
// 选择全部
selectAll(selection) {
// data第一层只要有在selection里面就是全选
const isSelect = selection.some(el => {
const tableDataIds = this.tableData.map(j => j.id)
return tableDataIds.includes(el.id)
})
// data第一层只要有不在selection里面就是全不选
const isCancel = !this.tableData.every(el => {
const selectIds = selection.map(j => j.id)
return selectIds.includes(el.id)
})
if (isSelect) {
selection.map(el => {
if (el.children) {
// 解决子组件没有被勾选到
this.setChildren(el.children, true)
}
})
}
if (isCancel) {
this.tableData.map(el => {
if (el.children) {
// 解决子组件没有被勾选到
this.setChildren(el.children, false)
}
})
}
},
selectionChange(list) {
this.selectionList = list;
this.$emit('searchList', this.ids)
},
treeLoad(tree, treeNode, resolve) {
const parentId = tree.id;
let childrenModel = []
getTreeList(parentId).then(res => {
childrenModel = res.data.data;
resolve(childrenModel);
// 如果父级选中,那么加载子集之后,子集设置选中
const hasPreSelect = this.selectionList?.some(el => {
return parentId === el.id
})
if (hasPreSelect) {
childrenModel.forEach( i=> {
this.toggleSelection(i, true)
})
}
});
this.$nextTick(() => {
tree.children = childrenModel
})
}
}
};
</script>
以上就是今天的全部内容,希望对你有所帮助,我们下期再见
PS: 是在一个大佬的代码基础上加的功能,参考博客:https://blog.csdn.net/Yzt_199626/article/details/117089321?spm=1001.2014.3001.5502
更多推荐
所有评论(0)