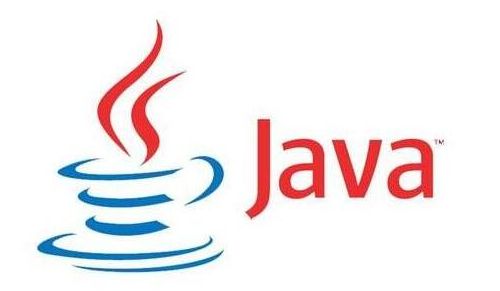
JAVA实现客户信息管理系统以及给大一寒假学生的建议
博客主页小吴_小吴有想法_CSDN博客-笔记,java领域博主欢迎关注点赞收藏和留言天道酬勤,勤能补拙,和小吴一起加油吧大一新生,水平有限,恳请各位大佬指点,不胜感激!1.很多大一学生像我一样都是大一上学期学习C语言,而元旦过后,怀着奋斗的激情,脑海中是否会闪过一丝彷徨?在这里给小伙伴们一点我的建议和看法:1.首先,基础非常重要,如果上学期学的c语言的语法还不够扎实,可以先不急着入门另一门语言,找
博客主页 小吴_小吴有想法_CSDN博客-笔记,java领域博主
欢迎关注
点赞
收藏和留言
天道酬勤,勤能补拙,和小吴一起加油吧
大一新生,水平有限,恳请各位大佬指点,不胜感激!
1.很多大一学生像我一样都是大一上学期学习C语言,而元旦过后,怀着奋斗的激情,脑海中是否会闪过一丝彷徨?
在这里给小伙伴们一点我的建议和看法:
1.首先,基础非常重要,如果上学期学的c语言的语法还不够扎实,可以先不急着入门另一门语言,找一些c语言大佬的总结和笔记消化消化刷刷题巩固一下!(可以直接在csdn上搜c语言大总结)
2.如果报了蓝桥杯(java组)等知识竞赛,可以先了解下java(javase),然后进行系统的算法学习。下面是给小伙伴们的一些建议和我自己走的一些弯路的总结
目录
1.JavaSE基础知识
JavaSE是大部分小伙伴入门必须经历的一个阶段,我也正在努力学习它,下面是我的一些想法:
相信一些小伙伴们在搜索学习路线时大多会看到以下几本书
1.这几本书我刚开始都看了一段时间,我觉得Java编程思想不太适合Java零基础的小白看,等有一定语法基础后再看还是很有帮助的(当然,这些都需要根据个人情况哈)
2.Java核心卷写的也非常全面和详细,我觉得零基础的小伙伴可以先用Java从入门到精通这本书入门并结合Java经典编程300例上面的例题去练习巩固,学一单元就可以刷上面对应每一单元的题,再以核心卷为主去归纳总结(当然,前期主要以看视频学习为主)
在这里推荐几个哔哩哔哩上的几个视频1.尚硅谷尚硅谷Java入门视频教程(在线答疑+Java面试真题)_哔哩哔哩_bilibili
2.黑马程序员黑马程序员全套Java教程_Java基础入门视频教程,零基础小白自学Java必备教程_哔哩哔哩_bilibili 3.动力节点https://search.bilibili.com/all?keyword=%E5%8A%A8%E5%8A%9B%E8%8A%82%E7%82%B9&
小伙伴们可以根据他们的讲课风格挑选自己习惯的看,对于入门,多写多练,成千上万行代码很有帮助!
3.推荐小伙伴们下载typora这个软件,用来记笔记,包括代码块等功能,并分类记还是挺方便的。而且每天也可以把记的笔记同步到博客里去,养成习惯
好啦,下面的客户信息管理系统算是我对前面所学的一些语法知识的巩固和总结
2.客户信息管理软件的功能介绍
一些小知识点:
1.charAt(int index)方法是一个能够用来检索特定索引下的字符的String实例的方法。charAt()方法返回指定索引位置的char值,索引范围是0~length()-1.
2.equals()比较的是同一个类型的两个不同对象里的属性们是否都相等,相等则返回true,否则返回false。
3.Integer.parselent(String)的作用是将String字符类型数据转换为Integer整型数据。
4.try..catch是用于防止程序出现崩溃而不能处理的时。try catch用于异常捕获,try分支语句执行出现异常被catch捕捉后会执行catch分支语句。
在写客户信息管理系统的时候分4个板块
1.最容易上手的Customer类
package ak;
public class Customer {
private String name;
private char gender;
private int age;
private String phone;
private String email;
public Customer()
{
}
public Customer(String name,char gender,int age,String phone,String email)
{
this.name=name;//姓名name
this.gender=gender;//性别gender
this.age=age;//年龄age
this.phone=phone;//电话phone
this.email=email;//邮箱email
}
public String getName()//获得姓名
{
return name;
}
public void setName(String name)//修改姓名,下面的属性皆一样
{
this.name=name;
}
public char getGender()
{
return gender;
}
public void setGender(char gender)
{
this.gender=gender;
}
public int getAge()
{
return age;
}
public void setAge(int age)
{
this.age=age;
}
public String getPhone()
{
return phone;
}
public void setPhone(String phone)
{
this.phone=phone;
}
public String getEmail()
{
return email;
}
public void setEmail(String email)
{
this.email=email;
}
}
2.紧接着处理CustomerList类
package ak;
import ak.Customer;//调用Customer类
public class CustomerList {
private Customer [] customers;//创立对象数组(如果不是很容易接受,可以看下尚硅谷老师讲对象数组那几节课)
private int total=0;//代表客户数量
public CustomerList(int totalCustomer)
{
customers=new Customer[totalCustomer];//设置构造器处理数组长度
}
public int getTotal()
{
return total;
}
public boolean addCustomer(Customer customer)//增删改查第一步,添加对象=客户
{
if(total<customers.length)
{
customers[total++]=customer;
return true;
}else
return false;
}
public boolean replaceCustomer(int index,Customer customer)//改动对象=客户
{
if(index>=0&&index<total)
{
customers[index]=customer;
return true;
}
else
return false;
}
public boolean deleteCustomer(int index)//删除,将指定要删除的索引位置元素前移
{
if(index>=0&&index<total)
{
for(int i=index;i<total-1;i++)
{
customers[i]=customers[i+1];
}
customers[total-1]=null;
total--;
return true;
}else
return false;
}
public Customer[] getALLCustomers()//查,像c语言的指针函数一样,返回的是地址
{
Customer[] a=new Customer[total];
for(int i=0;i<total;i++)
{
a[i]=customers[i];
}
return customers;
}
public Customer getCustomer(int index)//查找指定位置的客户=对象
{
if(index>=0&&index<total)
{
return customers[index];
}else
return null;
}
}
3.CMUtility类——处理客户信息(性别,电话,邮箱)的细节等
package ak;
import java.util.*;
public class CMUtility {
private static Scanner m=new Scanner(System.in);
private static String readKeyBoard(int limit,boolean bank)//处理信息的,下面很多都要用到
{
for(; ;)
{
String str=m.nextLine();
if(str.length()>=0&&str.length()<=limit)//这个获取字符串的长度不要与获取数组的长度搞混
{
return str;
}
else if(bank)
{
return str;
}
else
System.out.print("请输入长度不超过"+limit+"的字符串");
}
}
public static char readMenuSelection()//用于处理我们要操作的选项,增删改查的哪一种
{
char n;
for(;;)
{
String str=readKeyBoard(1,false);
n=str.charAt(0);
if(n=='1'||n=='2'||n=='3'||n=='4'||n=='5')
{
return n;
}
else
{
System.out.println("选择错误,请重新输入");
}
}
}
public static char readChar()//获取性别,下面利用方法重载来解决当我们修改客户的信息,但是不修改性别时该怎样实现
{
String str=readKeyBoard(1,false);
return str.charAt(0);
}
public static char readChar(char defaultValue)
{
String str=readKeyBoard(1,true);//这里用true,这样我们只有用回车跳过才可以达到我们所需,嘿嘿,到时候我们只需要让参数是本来的数即可
return (str.length()==0)?defaultValue:str.charAt(0);
}
public static int readInt()//获取年龄
{
int s;
for(; ;)
{
String str=readKeyBoard(2,false);
try {
s = Integer.parseInt(str);
return s;
}catch (NumberFormatException e) {
System.out.println("数字输入错误,请重新输入。");
}
}
}
public static int readInt(int defaultValue)//修改年龄,当然也可能不需要改,这时候与上面修改性别的函数有着异曲同工之妙
{
int s;
for(; ;)
{
String str=readKeyBoard(2,true);
if (str.equals(""))//注意这里与修改性别又不想修改时的区别
{
return defaultValue;
}
try {
s= Integer.parseInt(str);
return s;
}
catch (NumberFormatException e) {
System.out.println("数字输入错误,请重新输入。");
}
}
}
public static String readString(int limit)//姓名,电话,邮箱一块搞
{
return readKeyBoard(limit,false);
}
public static String readString(int limit,String defaultValue)//修改姓名,电话,邮箱,不输入信息直接回车咯!
{
String str=readKeyBoard(limit,true);
return str.equals("")?defaultValue:str;
}
public static char readConfirmSelection()//菜单的删除对象=客户的操作,会显示是否确认删除
{
for(; ;)
{
String str=readKeyBoard(1,false).toUpperCase();
char m=str.charAt(0);
if(m=='Y'||m=='N')
{
return m;
}
else
{
System.out.println("选择错误,请重新输入");
}
}
}
}
4.CustomerView类,主类!
package ak;
import ak.Customer;
import ak.CustomerList;
import ak.CMUtility;
public class CustomerView {
private CustomerList customerList=new CustomerList(10);//设置对象数组长度
public CustomerView()//先搞一下客户信息做对照
{
Customer customer=new Customer("传龙",'男',19,"219043","433@qq.com");//注意,年龄19那里只需要写数字就行,不要加“ ”或者’ ‘
customerList.addCustomer(customer);
}
public void enterMainmenu()//来啦来啦,主界面安排上!
{
boolean a=true;
while(a)
{
System.out.println("-----------------客户信息管理软件-----------------");
System.out.println(" 1 添加客户");
System.out.println(" 2 修改客户 ");
System.out.println(" 3 删除客户 ");
System.out.println(" 4 客户列表 ");
System.out.println(" 5 退 出 ");
System.out.println(" 请选择(1-5): ");
char select=CMUtility.readMenuSelection();
switch(select)
{//注意,这里要用'1',而不是1,呜呜我刚开始就忘记了
case '1':
addNewCustomer();
break;
case '2':
modifyCustomer();
break;
case '3':
deleteCustomer();
break;
case '4':
listALLCustomers();
break;
case '5':
System.out.println("是否确认退出(Y/N)?");
char c=CMUtility.readConfirmSelection();
if(c=='Y')
{
a=false;
}
}
}
}
public void addNewCustomer()//添加客户
{
int total=customerList.getTotal();
if(total<customerList.getALLCustomers().length)
{
System.out.println("客户姓名:");
String name=CMUtility.readString(10);
System.out.println("客户性别:");
char gender=CMUtility.readChar();
System.out.println("客户年龄:");
int age=CMUtility.readInt();
System.out.println("客户电话:");
String tel=CMUtility.readString(13);
System.out.println("客户邮箱");
String email=CMUtility.readString(15);
Customer cust=new Customer(name,gender,age,tel,email);
customerList.addCustomer(cust);
System.out.println("-----------------添加成功-----------------\t");
}else{
System.out.println("-----------客户列表已满,添加失败-------------\t");
}
}
public void modifyCustomer()//修改客户
{
System.out.println("-----------------修改客户-----------------");
int index;
Customer cust;
for(; ;)
{
System.out.println("请输入要修改的客户序号(-1)退出");
index=CMUtility.readInt();
if(index==-1)
{
return ;//注意,这里如果用break只是会退出for循环,不会退出修改客户这个方法
}
index--;//虽然我们选中比如说是修改客户4,但是数组是从0开始储存的,所以这时候把序号-1即为元素位置!
cust=customerList.getCustomer(index);
if(cust==null)
{
System.out.println("无法找到客户");
}
else
{
break;
}
}
System.out.println("姓名("+cust.getName()+"):");
String name=CMUtility.readString(10,cust.getName());
System.out.println("性别("+cust.getGender()+"):");
char gender=CMUtility.readChar(cust.getGender());
System.out.println("年龄("+cust.getAge()+"):");
int age=CMUtility.readInt(cust.getAge());
System.out.println("电话("+cust.getPhone()+"):");
String phone=CMUtility.readString(13,cust.getPhone());
System.out.println("邮箱("+cust.getEmail()+"):");
String email=CMUtility.readString(15,cust.getEmail());
Customer newcust=new Customer(name,gender,age,phone,email);
boolean ss=customerList.replaceCustomer(index,newcust);
if(ss)
{
System.out.println("-----------------修 改 成 功-----------------\\t");
}
else
{
System.out.println("-----------------修 改 失 败-----------------\t");
}
}
public void deleteCustomer()//删除客户
{
System.out.println("-----------------删除客户-----------------");
Customer cust;
int index;
for (;;) {
System.out.println("请输入要删除的客户序号(输入-1退出)");
index = CMUtility.readInt();
if (index == -1) {
return;
}
index--;
cust = customerList.getCustomer(index);
if (cust == null) {
System.out.println("无法找到客户!");
} else {
break;
}
}
System.out.println("是否确认删除?(Y/N)");
char r=CMUtility.readConfirmSelection();
if(r=='Y')
{
boolean rr=customerList.deleteCustomer(index);
if(rr)
{
System.out.println("-----------------删 除 成 功-----------------\\t");
}
else
{
System.out.println("删除失败,请输入正确序号:1->\"+customerList.getTotal()");
}
}
}
public void listALLCustomers()//查找客户
{
System.out.println("-------------------客 户 列 表------------------\n");
int total=customerList.getTotal();
if(total==0)
{
System.out.println("没有客户记录!");
}
else
{
System.out.println("编号\t姓名\t性别\t年龄\t电话\t\t邮箱");//这里有2个\t的是因为在实际运行中我们发现电话太长啦,和上面不对照!
Customer[] custList=customerList.getALLCustomers();//把所有客户(对象)都搞过来!
for(int i=0;i<total;i++)
{ //注意这里序号是i+1,因为数组下标是从0开始遍历哈!
System.out.println(i+1 + "\t" + custList[i].getName() + "\t" + custList[i].getGender() + "\t"+
custList[i].getAge() + "\t" + custList[i].getPhone() + "\t\t" + custList[i].getEmail() + "\t");
}
}
System.out.println("-----------------客户列表完成-----------------\n");
}
public static void main(String[] args)
{
CustomerView jie=new CustomerView();
jie.enterMainmenu();
}
}
下面是我随便输入的几个信息演示:
如果有需要《java从入门到精通》,《Java编程思想》,《java核心卷》,《深入理解jvm虚拟机》等电子书可以私聊我哈!加油!
天道酬勤,勤能补拙,和小吴一起加油吧!
更多推荐
所有评论(0)