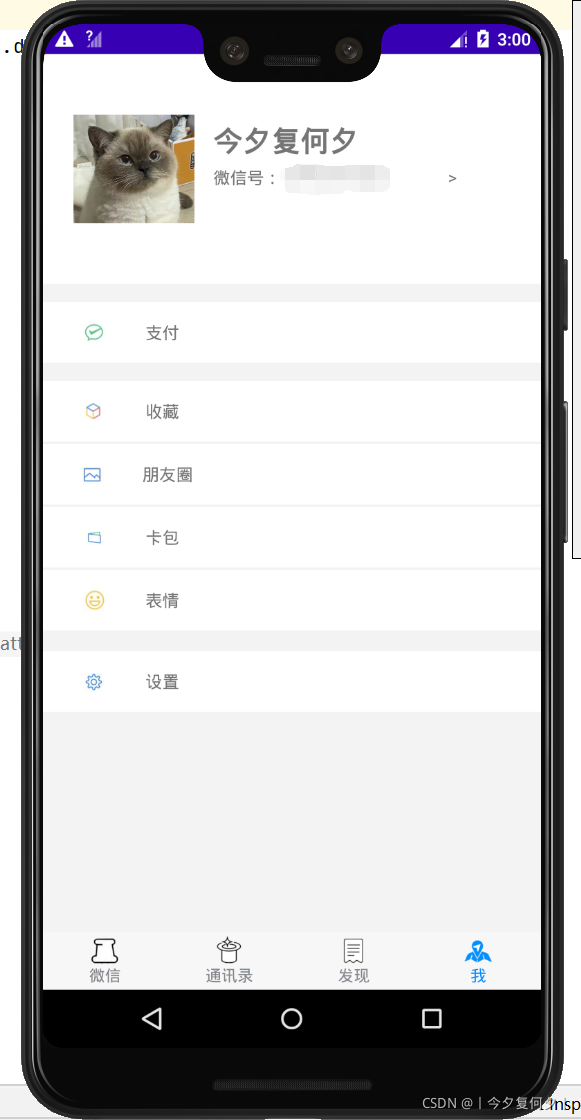
Android studio(安卓应用开发):实现微信中,“微信”和“我的”页面
效果图:(细节图片自换)代码:app-java-com.example.a1031-MainActivitypackage com.example.a1031;import android.annotation.SuppressLint;import android.app.Activity;import android.app.AlertDialog;import android.app.Dia
·
效果图:(细节图片自换)
代码:
app-java-com.example.a1031-MainActivity
package com.example.a1031;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.FragmentManager;
import android.app.FragmentTransaction;
import android.content.DialogInterface;
import android.graphics.Color;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentActivity;
import android.view.KeyEvent;
import android.view.View;
import android.view.Window;
import android.widget.ImageView;
import android.widget.TextView;
public class MainActivity extends Activity implements View.OnClickListener {
private FragmentOne firstFragment = null;// 用于显示第一个界面
private FragmentTwo secondFragment = null;// 用于显示第二个界面
private FragmentThree thirdFragment = null;// 用于显示第三个界面
private FragmentFour fourthFragment = null;// 用于显示第四个界面
private View firstLayout = null;// 第一个显示布局
private View secondLayout = null;// 第二个显示布局
private View thirdLayout = null;// 第三个显示布局
private View fourthLayout = null;// 第四个显示布局
private ImageView message_image = null;
private ImageView news_image = null;
private ImageView contacts_image = null;
private ImageView setting_image = null;
private TextView message_text = null;
private TextView news_text = null;
private TextView contacts_text = null;
private TextView setting_text = null;
private FragmentManager fragmentManager = null;// 用于对Fragment进行管理
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);//要求窗口没有title
super.setContentView(R.layout.activity_main);
// 初始化布局元素
initViews();
fragmentManager = getFragmentManager();//用于对Fragment进行管理
// 设置默认的显示界面
setTabSelection(0);
}
/**
* 在这里面获取到每个需要用到的控件的实例,并给它们设置好必要的点击事件
*/
@SuppressLint("NewApi")
public void initViews() {
fragmentManager = getFragmentManager();
firstLayout = findViewById(R.id.message_layout);
secondLayout = findViewById(R.id.news_layout);
thirdLayout = findViewById(R.id.contacts_layout);
fourthLayout = findViewById(R.id.setting_layout);
message_image = (ImageView) findViewById(R.id.message_image);
news_image = (ImageView) findViewById(R.id.news_image);
contacts_image = (ImageView) findViewById(R.id.contacts_image);
setting_image = (ImageView) findViewById(R.id.setting_image);
message_text = (TextView) findViewById(R.id.message_text);
news_text = (TextView) findViewById(R.id.news_text);
contacts_text = (TextView) findViewById(R.id.contacts_text);
setting_text = (TextView) findViewById(R.id.setting_text);
//处理点击事件
firstLayout.setOnClickListener(this);
secondLayout.setOnClickListener(this);
thirdLayout.setOnClickListener(this);
fourthLayout.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.message_layout:
setTabSelection(0);// 当点击了我的主页tab时,选中第1个tab
break;
case R.id.news_layout:
setTabSelection(1);// 当点击了购物车tab时,选中第2个tab
break;
case R.id.contacts_layout:
setTabSelection(2);// 当点击了我的订单tab时,选中第3个tab
break;
case R.id.setting_layout:
setTabSelection(3);// 当点击了个人中心tab时,选中第4个tab
break;
default:
break;
}
}
/**
* 根据传入的index参数来设置选中的tab页 每个tab页对应的下标。0表示主页,1表示支出,2表示收入,3表示设置
*/
@SuppressLint("NewApi")
private void setTabSelection(int index) {
clearSelection();// 每次选中之前先清除掉上次的选中状态
FragmentTransaction transaction = fragmentManager.beginTransaction();// 开启一个Fragment事务
hideFragments(transaction);// 先隐藏掉所有的Fragment,以防止有多个Fragment显示在界面上的情况
switch (index) {
case 0:
// 当点击了我的主页tab时改变控件的图片和文字颜色
message_image.setImageResource(R.drawable.fa_1);//修改布局中的图片
message_text.setTextColor(Color.parseColor("#0090ff"));//修改字体颜色
if (firstFragment == null) {
// 如果FirstFragment为空,则创建一个并添加到界面上
firstFragment = new FragmentOne();
transaction.add(R.id.content, firstFragment);
} else {
// 如果FirstFragment不为空,则直接将它显示出来
transaction.show(firstFragment);//显示的动作
}
break;
// 以下和firstFragment类同
case 1:
news_image.setImageResource(R.drawable.fc_1);
news_text.setTextColor(Color.parseColor("#0090ff"));
if (secondFragment == null) {
secondFragment = new FragmentTwo();
transaction.add(R.id.content, secondFragment);
} else {
transaction.show(secondFragment);
}
break;
case 2:
contacts_image.setImageResource(R.drawable.fb_1);
contacts_text.setTextColor(Color.parseColor("#0090ff"));
if (thirdFragment == null) {
thirdFragment = new FragmentThree();
transaction.add(R.id.content, thirdFragment);
} else {
transaction.show(thirdFragment);
}
break;
case 3:
setting_image.setImageResource(R.drawable.fd_1);
setting_text.setTextColor(Color.parseColor("#0090ff"));
if (fourthFragment == null) {
fourthFragment = new FragmentFour();
transaction.add(R.id.content, fourthFragment);
} else {
transaction.show(fourthFragment);
}
break;
}
transaction.commit();
}
/**
* 清除掉所有的选中状态
*/
private void clearSelection() {
message_image.setImageResource(R.drawable.fa);
message_text.setTextColor(Color.parseColor("#82858b"));
contacts_image.setImageResource(R.drawable.fb);
contacts_text.setTextColor(Color.parseColor("#82858b"));
news_image.setImageResource(R.drawable.fc);
news_text.setTextColor(Color.parseColor("#82858b"));
setting_image.setImageResource(R.drawable.fd);
setting_text.setTextColor(Color.parseColor("#82858b"));
}
/**
* 将所有的Fragment都设置为隐藏状态 用于对Fragment执行操作的事务
*/
@SuppressLint("NewApi")
private void hideFragments(FragmentTransaction transaction) {
if (firstFragment != null) {
transaction.hide(firstFragment);
}
if (secondFragment != null) {
transaction.hide(secondFragment);
}
if (thirdFragment != null) {
transaction.hide(thirdFragment);
}
if (fourthFragment != null) {
transaction.hide(fourthFragment);
}
}
//封装一个AlertDialog
private void exitDialog() {
Dialog dialog = new AlertDialog.Builder(this)
.setTitle("温馨提示")
.setMessage("您确定要退出程序吗?")
.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface arg0, int arg1) {
finish();
}
})
.setNegativeButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface arg0, int arg1) {
}
}).create();
dialog.show();//显示对话框
}
/**
* 返回菜单键监听事件
*/
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
if (keyCode == KeyEvent.KEYCODE_BACK) {//如果是返回按钮
exitDialog();
}
return super.onKeyDown(keyCode, event);
}
}
app-java-com.example.a1031-FragmentOne
package com.example.a1031;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class FragmentOne extends Fragment {
private int[] pic ={R.drawable.fa,R.drawable.fb,R.drawable.fc,R.drawable.fd,R.drawable.fa,};
private String value[][] = {
{"禅城第一靓仔","去老广喝早茶","上午 10:10"},
{"顺德第一靓仔","去老广喝早茶","上午 10:15"},
{"三水第一靓仔","去老广喝早茶","上午 10:20"},
{"高明第一靓仔","去老广喝早茶","上午 10:25"},
{"南海第一靓仔","去老广喝早茶","上午 10:30"},
};
//定义一个map集合存放数据
private List<Map<String,String>> list = new ArrayList<>();
private ListView datalist;
private SimpleAdapter simpleAdapter;//适配器
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_fragment_one, container, false);
datalist =view.findViewById(R.id.datalist);
for(int i=0;i<pic.length;i++){
Map<String,String> map = new HashMap<>();
map.put("pic",String.valueOf(pic[i]));
map.put("qmc",value[i][0]);
map.put("content",value[i][1]);
map.put("time",value[i][2]);
list.add(map);//将map放到list集合中
}
simpleAdapter = new SimpleAdapter(getActivity(),
this.list,
R.layout.listveiw,
new String[]{"pic","qmc","content","time"},
new int[]{R.id.img1,R.id.qmc,R.id.content,R.id.time}
);
datalist.setAdapter(simpleAdapter);
// Inflate the layout for this fragment
return view;
}
}
app-java-FragmentFour
package com.example.a1031;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.app.Fragment;
public class FragmentFour extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState)
{
View fourthLayout = inflater.inflate(R.layout.fragment_fragment_four, container, false);
return fourthLayout;
}
}
app-java-com.example.a1031-FragmentThree
package com.example.a1031;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class FragmentThree extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_fragment_three, container, false);
}
}
app-java-com.example.a1031-FragmentTwo
package com.example.a1031;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class FragmentTwo extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_fragment_two, container, false);
}
}
app-res-layout-activity_main
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
<FrameLayout
android:id="@+id/content"
android:layout_width="match_parent"
android:layout_height="match_parent">
</FrameLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#f7f7f7"
android:gravity="center"
android:orientation="horizontal"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true">
<LinearLayout
android:id="@+id/message_layout"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_centerVertical="true"
android:layout_weight="1"
android:orientation="vertical"
android:padding="3dp">
<ImageView
android:id="@+id/message_image"
android:layout_width="wrap_content"
android:layout_height="24dp"
android:layout_gravity="center_horizontal"
android:src="@drawable/fa" />
<TextView
android:id="@+id/message_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:gravity="top"
android:text="微信"
android:textColor="#82858b"
android:textSize="13dp" />
</LinearLayout>
<LinearLayout
android:id="@+id/news_layout"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_centerVertical="true"
android:layout_weight="1"
android:orientation="vertical"
android:padding="3dp">
<ImageView
android:id="@+id/news_image"
android:layout_width="wrap_content"
android:layout_height="24dp"
android:layout_gravity="center_horizontal"
android:src="@drawable/fb" />
<TextView
android:id="@+id/news_text"
android:layout_width="wrap_content"
android:layout_height="20dp"
android:layout_gravity="center_horizontal"
android:gravity="top"
android:text="通讯录"
android:textColor="#82858b"
android:textSize="13dp" />
</LinearLayout>
<LinearLayout
android:id="@+id/contacts_layout"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_centerVertical="true"
android:layout_weight="1"
android:orientation="vertical"
android:padding="3dp">
<ImageView
android:id="@+id/contacts_image"
android:layout_width="wrap_content"
android:layout_height="24dp"
android:layout_gravity="center_horizontal"
android:src="@drawable/fc" />
<TextView
android:id="@+id/contacts_text"
android:layout_width="wrap_content"
android:layout_height="20dp"
android:layout_gravity="center_horizontal"
android:gravity="top"
android:text="发现"
android:textColor="#82858b"
android:textSize="13dp" />
</LinearLayout>
<LinearLayout
android:id="@+id/setting_layout"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_centerVertical="true"
android:layout_weight="1"
android:orientation="vertical"
android:padding="3dp">
<ImageView
android:id="@+id/setting_image"
android:layout_width="wrap_content"
android:layout_height="24dp"
android:layout_gravity="center_horizontal"
android:src="@drawable/fd" />
<TextView
android:id="@+id/setting_text"
android:layout_width="wrap_content"
android:layout_height="20dp"
android:layout_gravity="center_horizontal"
android:gravity="top"
android:text="我"
android:textColor="#82858b"
android:textSize="13dp" />
</LinearLayout>
</LinearLayout>
</RelativeLayout>
app-res-layout-listveiw
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<ImageView
android:id="@+id/img1"
android:src="@drawable/fa_1"
android:layout_width="0px"
android:layout_weight="2"
android:layout_height="100px"/>
<LinearLayout
android:layout_width="0px"
android:layout_weight="6"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/qmc"
android:textColor="#FF0000"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="222222"/>
<TextView
android:id="@+id/content"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="33333"/>
</LinearLayout>
<LinearLayout
android:layout_width="0px"
android:layout_weight="2"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/time"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="上午 10:15" />
</LinearLayout>
</LinearLayout>
app-res-layout-fragment_fragment_one
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ListView
android:id="@+id/datalist"
android:layout_width="match_parent"
android:layout_marginTop="40dp"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
app-res-layout-fragment_fragment_two
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/ck2">
</LinearLayout>
app-res-layout-fragment_fragment_three
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/ck2">
</LinearLayout>
app-res-layout-fragment_fragment_four
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#F3F3F3"
android:orientation="vertical"
tools:context="com.example.a1031.MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#fff"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#fff"
android:layout_marginTop="50dp"
android:layout_marginBottom="50dp"
android:layout_marginLeft="25dp"
android:orientation="horizontal">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="5"
android:layout_marginRight="10dp"
android:adjustViewBounds="true"
android:src="@drawable/tx4" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="2"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:layout_marginTop="5dp"
android:text="今夕复何夕"
android:textSize="24dp"
android:textStyle="bold" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_marginLeft="5dp"
android:layout_marginTop="5dp"
android:layout_height="wrap_content"
android:text="微信号:15994975264" />
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:layout_marginLeft="50dp"
android:layout_weight="1"
android:text=">" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginTop="15dp"
android:layout_marginBottom="15dp"
android:background="#fff"
android:orientation="horizontal">
<ImageView
android:id="@+id/zf4"
android:layout_width="25dp"
android:layout_height="25dp"
android:layout_gravity="center"
android:layout_weight="1"
android:adjustViewBounds="true"
android:src="@drawable/zf4" />
<TextView
android:id="@+id/textView5"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:gravity="center_vertical"
android:layout_weight="5"
android:text="支付" />
</LinearLayout>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#fff"
android:orientation="horizontal">
<ImageView
android:id="@+id/imageView3"
android:layout_weight="1"
android:layout_width="25dp"
android:layout_height="25dp"
android:layout_gravity="center"
android:src="@drawable/sc4" />
<TextView
android:id="@+id/textView6"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:gravity="center_vertical"
android:layout_weight="5"
android:text="收藏" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#fff"
android:layout_marginTop="2dp"
android:layout_marginBottom="2dp"
android:orientation="horizontal">
<ImageView
android:id="@+id/imageView4"
android:layout_width="25dp"
android:layout_height="25dp"
android:layout_gravity="center"
android:layout_weight="1"
android:src="@drawable/pyq4" />
<TextView
android:id="@+id/textView7"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:gravity="center_vertical"
android:layout_weight="5"
android:text="朋友圈" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#fff"
android:orientation="horizontal">
<ImageView
android:id="@+id/imageView5"
android:layout_width="25dp"
android:layout_height="20dp"
android:layout_gravity="center"
android:layout_weight="1"
android:src="@drawable/kb4" />
<TextView
android:id="@+id/textView8"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:gravity="center_vertical"
android:layout_weight="5"
android:text="卡包" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#fff"
android:layout_marginTop="2dp"
android:layout_marginBottom="2dp"
android:orientation="horizontal">
<ImageView
android:id="@+id/imageView6"
android:layout_width="25dp"
android:layout_height="25dp"
android:layout_gravity="center"
android:layout_weight="1"
android:src="@drawable/bq4" />
<TextView
android:id="@+id/textView9"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:gravity="center_vertical"
android:layout_weight="5"
android:text="表情" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginTop="15dp"
android:background="#fff"
android:orientation="horizontal">
<ImageView
android:id="@+id/imageView7"
android:layout_width="25dp"
android:layout_height="25dp"
android:layout_gravity="center"
android:layout_weight="1"
android:src="@drawable/sz4" />
<TextView
android:id="@+id/textView10"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:gravity="center_vertical"
android:layout_weight="5"
android:text="设置" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
更多推荐
所有评论(0)