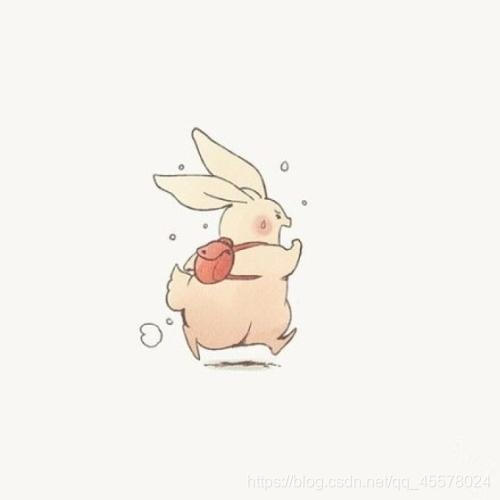
vue实现弹窗子组件打开关闭的几种方法
1. 直接在父组件内操作子组件的变量this.$refs.dialogName.showDialog = true直接(耦合度太高,不推荐直接操作子组件)
·
方法一:直接在父组件内操作子组件的变量
父组件:
父组件:
<template>
<div>
<el-button @click="lookChild">查看</el-button>
<child ref="childDialog" />
</div>
</template>
<script>
import child from './components/dialog/child'
export default {
methods: {
lookChild() {
this.$refs.childDialog.showDialog = true
}
}
}
</script>
子组件:
<template>
<el-dialog
title="弹窗标题"
:visible.sync="showDialog"
width="80%"
center
>
弹窗内容
</el-dialog>
</template>
<script>
export default {
data() {
return {
showDialog: false
}
}
}
</script>
(耦合度太高,不推荐直接操作子组件)
方法二:通过v-if控制弹窗组件的销毁,重建
父组件:
<template>
<div>
<el-button @click="lookChild">查看</el-button>
<child v-if=“showChildDialog” @dialogClose="dialogClose" />
</div>
</template>
<script>
import child from './components/dialog/child'
export default {
data() {
return {
showChildDialog: false
}
},
methods: {
lookChild() {
this.showChildDialog = true
},
/** 关闭弹窗 */
dialogClose() {
this.showChildDialog = false
}
}
}
</script>
子组件:
<template>
<el-dialog
title="弹窗标题"
:visible.sync="showDialog"
width="80%"
center
@close="dialogClose"
>
弹窗内容
</el-dialog>
</template>
<script>
export default {
data() {
return {
showDialog: true
}
},
methods: {
dialogClose() {
this.$emit('dialogClose')
}
}
}
</script>
(v-if会销毁,重建弹窗,若弹窗内容较多,重建会增加大量渲染开销)
方法三:父子组件传值(推荐)
父组件:
<template>
<div>
<el-button @click="lookChild">查看</el-button>
<child :show-child-dialog.sync="showDialog" />
</div>
</template>
<script>
import child from './components/dialog/child'
export default {
data() {
return {
showDialog: false
}
},
methods: {
lookChild() {
this.showDialog = true
}
}
}
</script>
子组件:
<template>
<el-dialog
title="弹窗标题"
:visible.sync="showDialog"
width="80%"
center
>
弹窗内容
</el-dialog>
</template>
<script>
export default {
props: {
showChildDialog: {
type: Boolean,
default: false
}
},
computed: {
showDialog: {
get: function() {
return this.showChildDialog
},
set: function(newValue) {
this.$emit('update:showChildDialog', newValue) // 触发更新事件,父组件的showDialog会自动更新
}
}
}
}
</script>
- .sync修饰符:它会被扩展为一个自动更新父组件属性的 v-on 监听器
更多推荐
所有评论(0)