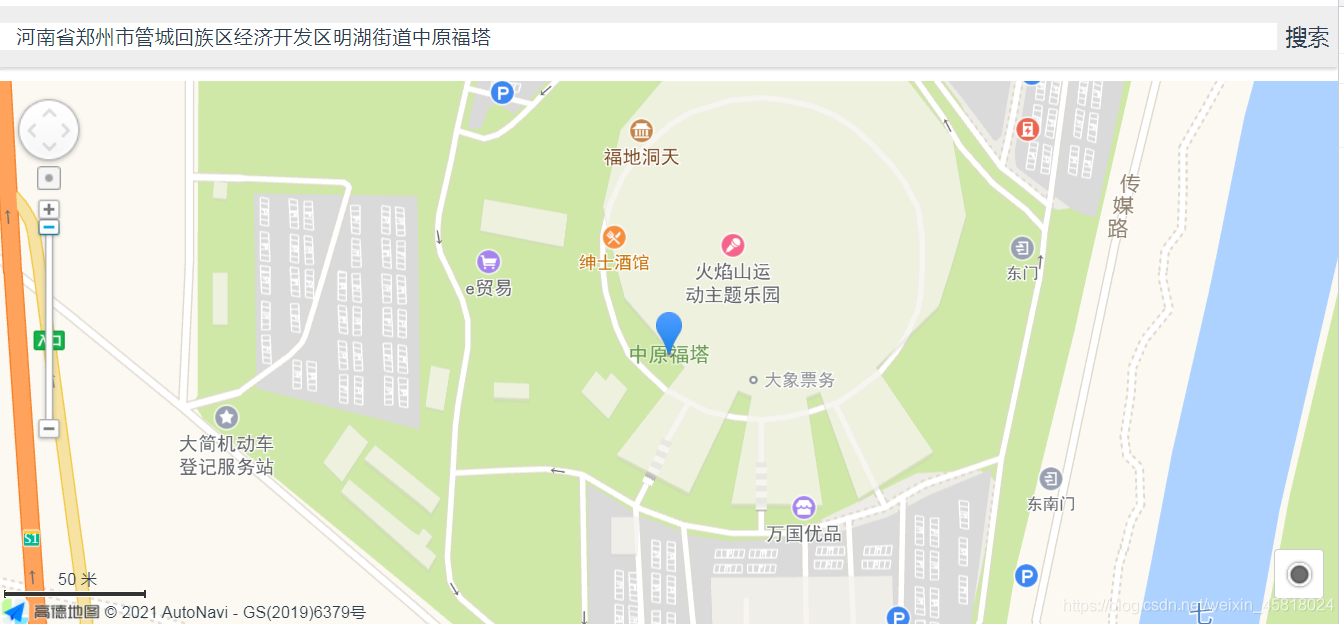
vue中使用高德地图 vue-amap 常见配置
效果:
·
效果:
使用:
先下载依赖包 npm install vue-amap --save
安装、引入依赖
import VueAMap from 'vue-amap'
VueAMap.initAMapApiLoader({
key: '自己高德地图的key',
plugin: [
// 定位空间,用来获取和展示用户主机所在的经纬度位置
'AMap.Geolocation',
// 输入提示插件
'AMap.Autocomplete',
// POI搜索插件
'AMap.PlaceSearch',
// 右下角缩略图插件,比例尺
'AMap.Scale',
// 地图鹰眼插件
'AMap.OverView',
// 地图工具条
'AMap.ToolBar',
// 类别切换空间,实现默认图层与卫星图,实施交通层之间切换的控制
'AMap.MapType',
// 编辑 折线多边形
'AMap.PolyEditor',
'AMap.CircleEditor',
// 地图编码
'AMap.Geocoder'
],
// 默认高德 sdk 版本为 1.4.4
v: '1.4.4'
})
Vue.use(VueAMap)
代码:
HTML:
<div class="amap-page-container">
<el-amap-search-box
class="search-box"
:search-option="searchOption"
:on-search-result="onSearchResult"
></el-amap-search-box>
<!-- 搜索框 -->
<!-- <el-amap-search-box
class="search-boxMap"
:search-option="searchOption"
:on-search-result="onSearchResult"
>
</el-amap-search-box> -->
<el-amap
ref="map"
vid="amapDemo"
:amap-manager="amapManager"
:center="center"
:zoom="zoom"
:plugin="plugin"
:events="events"
:rightclick="rightclick"
class="amap-demo"
>
<!-- 在地图上标记点 -->
<el-amap-marker
v-for="(marker,index) in markers"
:key="index"
:position="marker"
>
</el-amap-marker>
<!-- 坐标点 -->
<el-amap-marker
vid="amapDemo"
:position="center"
></el-amap-marker>
<!-- <el-amap-info-window
:position="currentWindow.position"
:content="currentWindow.content"
:visible="currentWindow.visible"
:events="currentWindow.events"
>
</el-amap-info-window> -->
<!-- 放置图片 -->
<!-- <el-amap-ground-image
v-for="(groundimage,index) in groundimages"
:url="groundimage.url"
:key="index+'1'"
:bounds="groundimage.bounds"
:events="groundimage.events"
>
</el-amap-ground-image> -->
<!-- 文字 -->
<el-amap-text
v-for="(text,index) in texts"
:text="text.text"
:key="index+'index'"
:offset="text.offset"
:position="text.position"
:events="text.events"
>
</el-amap-text>
</el-amap>
<!-- 点击地图的按钮 -->
<div class="toolbar">
<button @click="switchWindow(0)">展示第一个位置</button>
<button @click="switchWindow(1)">展示第二个位置</button>
</div>
</div>
js片段:
import { AMapManager } from 'vue-amap'
const amapManager = new AMapManager()
let Geocoder
export default {
data () {
const self = this
return {
loaded: false,
amapManager,
zoom: 20,
input: '',
searchOption: {
city: '',
citylimit: false
},
center: [113.728679, 34.723295],
events: {
// init: (o) => {
// o.getCity((result) => {
// console.log(result)
// })
// },
moveend: () => { },
zoomchange: () => { },
click: (e) => {
self.center = [e.lnglat.lng, e.lnglat.lat]
Geocoder.getAddress(self.center, function (status, result) {
if (status === 'complete' && result.info === 'OK') {
self.input = result.regeocode.formattedAddress
document.querySelector('.search-box-wrapper input').value =
self.input
}
})
},
// 右键点击事件
rightclick (e) {
self.rightclick(e)
}
},
// ToolBar工具条插件、MapType插件、Scale比例尺插件、OverView鹰眼插件
plugin: [
// 定位插件 右下
{
pName: 'Geolocation',
// 是否使用高精度定位,默认true
enableHighAccuracy: true,
// 超过10秒后停止定位,默认:无穷大
timeout: 100,
// 自动偏移后的坐标为高德坐标,默认:true
convert: true,
// 显示定位按钮,默认:true
showButton: true,
// 定位按钮停靠位置,默认'LB',左下角
buttonPosition: 'RB',
// 定位成功后在定位到的位置显示标记,默认:true
showMarker: true,
// 定位成功后用圆圈表示定位精度范围,默认:true
showCircle: true,
// 定位成功后将定位到的位置作为地图中心点,默认true
panToLocation: true,
// 定位成功后调整地图视野范围使定位位置及精度范围视野内可见,默认:false
zoomToAccuracy: true,
extensions: 'all',
events: {
init (o) {
o.getCurrentPosition((status, result) => {
if (status === 'error') return alert('定位失败')
if (result && result.position) {
const lng = result.position.lng
const lat = result.position.lat
self.center = [lng, lat]
self.loaded = true
self.zoom = 10
self.$nextTick()
}
})
}
}
},
{
pName: 'Geocoder',
events: {
init (o) {
Geocoder = o
// o.getAddress(self.center, function (status, result) {
// if (status === 'complete' && result.info === 'OK') {
// self.input = result.regeocode.formattedAddress
// document.querySelector('.search-box-wrapper input').value =
// self.input
// }
// })
}
}
},
// 地图工具条插件 左上
{
pName: 'ToolBar',
events: {
// init (o) {
// console.log(o)
// }
}
},
{
pName: 'MapType',
defaultType: 0
// events: {
// init (o) {
// console.log(o)
// }
// }
},
// 比例尺插件 左下
{
pName: 'Scale'
// events: {
// init (instance) {
// console.log(instance)
// }
// }
}, {
pName: 'OverView'
// events: {
// init (instance) {
// console.log(instance)
// }
// }
}],
markers: [
[121.49996, 31.297646],
[121.40018, 31.197622],
[121.49991, 31.207649]
],
windows: [ // 窗口信息框
{
position: [121.69996, 31.237646],
content: '<div>我在这里呢 !</div>',
visible: true,
events: {
close () {
console.log('关闭窗口一!')
}
}
}, {
position: [121.5875285, 31.21515044],
content: '<div>我在国际博览中心!</div>',
visible: true,
events: {
close () {
console.log('关闭窗口二!')
}
}
}
],
// 放置图片
groundimages: [
{
url: 'https://aos-cdn-image.amap.com/pp/avatar/a12/ce/fe/57710054.jpg?ver=1531037095&imgoss=1',
bounds: [[121.5273285, 31.21515044], [122.9273285, 32.31515044]],
events: {
click () {
alert('click groundimage')
}
}
}
],
// 放置文字
texts: [
{
position: [121.49996, 31.297646],
text: '<div>第一个</div>',
offset: [0, -50],
events: {
click: () => {
console.log('1')
}
}
},
{
position: [121.40018, 31.197622],
text: '<div>第二个</div>',
offset: [0, -50],
events: {
click: () => {
console.log('2')
}
}
}
],
// 当前窗口
currentWindow: {
position: [0, 0],
content: '',
events: {},
visible: false
}
}
},
methods: {
// 切换信息窗口
switchWindow (tab) {
this.currentWindow.visible = false
this.$nextTick(() => {
this.currentWindow = this.windows[tab]
this.currentWindow.visible = true
})
},
// 搜索事件
onSearchResult (pois) {
// this.input = document.querySelector('.search-box-wrapper input').value
// this.center = [pois[0].lng, pois[0].lat]
if (pois.length > 0) {
this.zoom = 16
var poi = pois[0]
const lng = poi.lng
const lat = poi.lat
this.center = [lng, lat]
}
// console.log(this.center)
},
rightclick (e) {
console.log(e)
}
}
}
css样式
<style lang='less' scoped>
// .search-boxMap {
// position: absolute;
// top: 25px;
// left: 70px;
// }
// .amap-page-container {
// position: relative;
// }
.amap-page-container {
width: 100%;
height: 400px;
margin-bottom: 20px;
}
.toolbar button {
background: #42b983;
border: 0;
color: white;
padding: 8px;
margin: 0 5px;
border-radius: 3px;
cursor: pointer;
margin-top: 10px;
}
</style>
<style>
.el-vue-search-box-container {
width: 100% !important;
margin-bottom: 10px;
}
.search-box-wrapper {
background-color: #eee;
}
</style>
或者可以使用封装的高德地图 效果不是太好看
地址:AMap-Vue
更多推荐
所有评论(0)