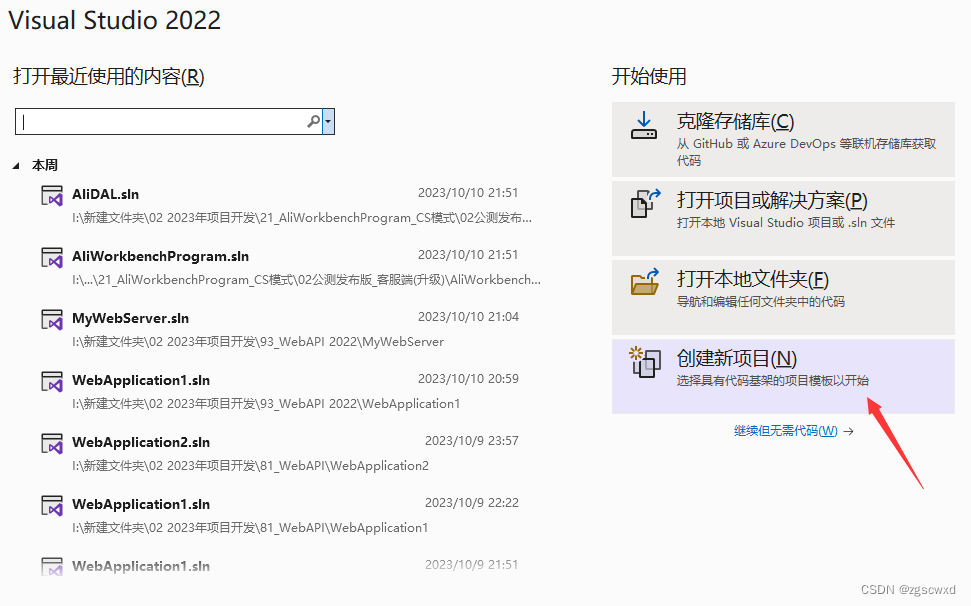
[入门一]C# webApi创建、与发布、部署、api调用
c# Web API实例,与发布、部署、api调用

一.创建web api项目
1.1、项目创建
MVC架构的话,它会有view-model-control三层,在web api中它的前端和后端是分离的,所以只在项目中存在model-control两层
1.2、修改路由
打开App_Start文件夹下,WebApiConfig.cs ,修改路由,加上{action}/ ,这样就可以在api接口中通过接口函数名,来导向我们希望调用的api函数,否则,只能通过controller来导向,就可能会造成有相同参数的不同名函数,冲突。其中,{id}是api接口函数中的参数。
默认路由配置信息为:【默认路由模板无法满足针对一种资源一种请求方式的多种操作。】
WebApi的默认路由是通过http的方法(get/post/put/delete)去匹配对应的action,也就是说webapi的默认路由并不需要指定action的名称
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web.Http;
namespace WebAPI
{
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Web API 配置和服务
// Web API 路由
config.MapHttpAttributeRoutes();
config.Routes.MapHttpRoute(
name: "DefaultApi",
//修改路由,加上{action}/ ,这样就可以在api接口中通过接口函数名,来导向我们希望调用的api函数,
//否则,只能通过controller来导向,就可能会造成有相同参数的不同名函数,冲突。其中,{id}是api接口函数中的参数
routeTemplate: "api/{controller}/{action}/{id}",
defaults: new { id = RouteParameter.Optional }
);
}
}
}
二.测试案例
写一个测试的api函数,并开始执行(不调试)
2.1、我们在model文件夹中添加一个类movie
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace WebAPI.Models
{
public class movie
{
public string name { get; set; }
public string director { get; set; }
public string actor { get; set; }
public string type { get; set; }
public int price { get; set; }
}
}
2.1.2、我们在model文件夹中添加一个类Product
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace WebAPI.Models
{
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public string Category { get; set; }
public decimal Price { get; set; }
}
}
2.2、在controller文件夹下添加web api控制器,命名改为TestController
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
using WebAPI.Models;
namespace WebAPI.Controllers
{
public class TestController : ApiController
{
movie[] mymovie = new movie[]
{
new movie { name="海蒂和爷爷",director="阿兰.葛斯彭纳",actor="阿努克",type="动漫",price=28},
new movie { name="云南虫谷",director="佚名",actor="潘粤明",type="惊悚",price=32},
new movie { name="沙海",director="佚名",actor="吴磊",type="惊悚",price=28},
new movie { name="千与千寻",director="宫崎骏",actor="千寻",type="动漫",price=28}
};
public IEnumerable<movie> GetAllMovies()
{
return mymovie;
}
public IHttpActionResult GetMovie(string name) //异步方式创建有什么作用
{
var mov = mymovie.FirstOrDefault((p) => p.name == name);
if (mymovie == null)
{
return NotFound();
}
return Ok(mymovie);
}
}
}
这样就完成了一个web api实例的编写
2.2.2、在controller文件夹下添加web api控制器,命名改为productsController
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
using WebAPI.Models;
namespace WebAPI.Controllers
{
public class productsController : ApiController
{
Product[] products = new Product[]
{
new Product { Id = 1, Name = "Tomato Soup", Category = "Groceries", Price = 1 },
new Product { Id = 2, Name = "Yo-yo", Category = "Toys", Price = 3.75M },
new Product { Id = 3, Name = "Hammer", Category = "Hardware", Price = 16.99M }
};
public IEnumerable<Product> GetAllProducts()
{
return products;
}
public IHttpActionResult GetProduct(int id)
{
var product = products.FirstOrDefault((p) => p.Id == id);
if (product == null)
{
return NotFound();
}
return Ok(product);
}
}
}
2.2.3、在controller文件夹下添加web api控制器,命名改为MyController
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
namespace WebAPI.Controllers
{
public class MyController : ApiController
{
[HttpGet]
public string MyExample(string param1, int param2)
{
string res = "";
res = param1 + param2.ToString();
//这边可以进行任意操作,比如数据存入或者取出数据库等
return res;
}
}
}
三.本地调试
3.1 运行调试,以本地 localhost(或127.0.0.1)形式访问
①点击工具栏【IIS Express】
②浏览地址输入接口,看是否可以访问
localhost:44381/api/products/GetAllProducts
注意:
这里的路径是写你的控制器前缀名称(Control文件下的productsController控制器文件的前缀)
https://localhost:44381/api/Test/GetAllMovies
2)直接在浏览器中调试也行
想要调试的值,可以将WebApiConfig.cs的代码修如下
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web.Http;
namespace WebAPI
{
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Web API 配置和服务
// Web API 路由
config.MapHttpAttributeRoutes();
config.Routes.MapHttpRoute(
name: "DefaultApi",
//修改路由,加上{action}/ ,这样就可以在api接口中通过接口函数名,来导向我们希望调用的api函数,
//否则,只能通过controller来导向,就可能会造成有相同参数的不同名函数,冲突。其中,{id}是api接口函数中的参数
routeTemplate: "api/{controller}/{action}/{id}",
defaults: new { id = RouteParameter.Optional }
);
//去掉xml返回格式、设置json字段命名采用
var appXmlType =
config.Formatters.XmlFormatter.SupportedMediaTypes.FirstOrDefault(t => t.MediaType == "application/xml");
config.Formatters.XmlFormatter.SupportedMediaTypes.Remove(appXmlType);
}
}
}
ok,显示成功
localhost:44381/api/My/MyExample?param1=¶m2=2
WebApi项目实例3-1
3-1 (1)新添加到控制器UserInfoController,
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
using WebAPI.Models;
namespace WebAPI.Controllers
{
public class UserInfoController : ApiController
{
//检查用户名是否已注册
private ApiTools tool = new ApiTools();
// [HttpPost]
[HttpGet]
public HttpResponseMessage CheckUserName(string _userName)
{
int num = UserInfoGetCount(_userName);//查询是否存在该用户
if (num > 0)
{
return tool.MsgFormat(ResponseCode.操作失败, "不可注册/用户已注册", "1 " + _userName);
}
else
{
return tool.MsgFormat(ResponseCode.成功, "可注册", "0 " + _userName);
}
}
private int UserInfoGetCount(string username)
{
//return Convert.ToInt32(SearchValue("select count(id) from userinfo where username='" + username + "'"));
return username == "admin" ? 1 : 0;
}
}
}
添加返回(响应)类ApiTools
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net.Http;
using System.Text.RegularExpressions;
using System.Web;
namespace WebAPI.Models
{
//添加返回(响应)类
public class ApiTools
{
private string msgModel = "{{\"code\":{0},\"message\":\"{1}\",\"result\":{2}}}";
public ApiTools()
{
}
public HttpResponseMessage MsgFormat(ResponseCode code, string explanation, string result)
{
string r = @"^(\-|\+)?\d+(\.\d+)?$";
string json = string.Empty;
if (Regex.IsMatch(result, r) || result.ToLower() == "true" || result.ToLower() == "false" || result == "[]" || result.Contains('{'))
{
json = string.Format(msgModel, (int)code, explanation, result);
}
else
{
if (result.Contains('"'))
{
json = string.Format(msgModel, (int)code, explanation, result);
}
else
{
json = string.Format(msgModel, (int)code, explanation, "\"" + result + "\"");
}
}
return new HttpResponseMessage { Content = new StringContent(json, System.Text.Encoding.UTF8, "application/json") };
}
}
public enum ResponseCode
{
操作失败 = 00000,
成功 = 10200,
}
}
3-1 (2)本地调试,调用Web API接口
运行调试,以本地 localhost(或127.0.0.1)形式访问
①点击工具栏【IIS Express】
②浏览地址输入接口,看是否可以访问
https://localhost:44381/api/UserInfo/CheckUserName?_userName=wxd
3.2 运行调试,以本地IP(192.168.6.152)形式访问
127.0.0.1是回路地址,来检验本机TCP/IP协议栈,实际使用过程中服务端不在本机,是外部地址,要用IP地址测试。
外部用户采用IP+端口号访问,如下图浏览器访问不了,400错误。
解决方案:
因为 IIS 7 采用了更安全的 web.config 管理机制,默认情况下会锁住配置项不允许更改。
以管理员身份运行命令行【此处不要操作】
C:\windows\system32\inetsrv\appcmd unlock config -section:system.webServer/handlers
如果modules也被锁定,再运行
C:\windows\system32\inetsrv\appcmd unlock config -section:system.webServer/modules
客户端程序:调用接口分为以下几种情况:
通过Javascript 和 jQuery 调用 Web API
右键资源管理器解决方案下面的项目,添加-新建项
将index.html内容替换成:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>Product App</title>
</head>
<body>
<div>
<h2>All Products</h2>
<ul id="products" />
</div>
<div>
<h2>Search by ID</h2>
<input type="text" id="prodId" size="5" />
<input type="button" value="Search" onclick="find();" />
<p id="product" />
</div>
<script src="https://ajax.aspnetcdn.com/ajax/jQuery/jquery-2.0.3.min.js"></script>
<script>
var uri = 'api/Products';
$(document).ready(function () {
// Send an AJAX request
$.getJSON(uri)
.done(function (data) {
// On success, 'data' contains a list of products.
$.each(data, function (key, item) {
// Add a list item for the product.
$('<li>', { text: formatItem(item) }).appendTo($('#products'));
});
});
});
function formatItem(item) {
return item.Name + ': $' + item.Price;
}
function find() {
var id = $('#prodId').val();
$.getJSON(uri + '/' + id)
.done(function (data) {
$('#product').text(formatItem(data));
})
.fail(function (jqXHR, textStatus, err) {
$('#product').text('Error: ' + err);
});
}
</script>
</body>
</html>
四.发布web api 并部署
4.1、首先,右键项目,选择发布:
到这里,程序已经发布到指定的路径下了(这里的路径,可以是本机的文件夹,也可以是服务器上的ftp路径)
4.2、我们还剩最后一步,就是,在IIS上,把发布的服务端程序挂上去,不说了,直接上图:
打开iis,选中网站,右键 添加网站,
好了,服务端程序发布并部署完成。
这个WebAPI就是刚刚我们部署好的,点击下图右侧的浏览*91(http),会打开网页
更多推荐
所有评论(0)