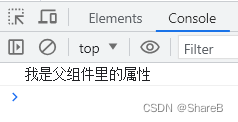
VUE3组件之间传值、方法调用
VUE3组件传值调用

一键AI生成摘要,助你高效阅读
问答
·
一、父组件给子组件、子子组件传值,子/子子组件万能调用父组件属性
使用provide、inject组件
父子组件之间的数据传递需要用到 props 属性,而 provide / inject 就是用来解决 props 多层嵌套的问题
父组件:parent.vue
<script setup>
import { reactive, getCurrentInstance, computed, provide } from 'vue'
import test from './test.vue'
const { proxy } = getCurrentInstance()
const _this = proxy
const theme = computed(() => AppStore.GET_THEME())
const state = reactive({
myData:'我是父组件里的属性',
})
provide(
//若直接写state.myData无效,需加computed
'parentData',computed(() => state.myData)
)
</script>
子组件:test.vue
<script setup>
import {reactive,getCurrentInstance,onMounted,inject,computed} from 'vue'
const {proxy} = getCurrentInstance()
const _this = proxy
const state = reactive({})
const parentData = inject('parentData')
onMounted(() => {
console.log(parentData.value)
})
</script>
二、常规父给子组件传值、子调父方法:props(defineProps)
父组件:parent.vue
<template>
<div class="account-sheet">
<div class="tabs-content">
<test :parentData="state.myData" :parentFunction="myFunction" />
</div>
</div>
</template>
<script setup>
import { reactive, getCurrentInstance, provide, computed } from 'vue'
import test from './test.vue'
const { proxy } = getCurrentInstance()
const _this = proxy
const theme = computed(() => AppStore.GET_THEME())
const state = reactive({
patientInfo: null,
ActiveIndex: 0,
myData:'我是父组件里的属性',
})
const myFunction = ()=>{
console.log('我是父组件里的方法')
}
</script>
子组件:test.vue
<script setup>
import {reactive,getCurrentInstance,onMounted,inject,computed} from 'vue'
const {proxy} = getCurrentInstance()
const _this = proxy
const state = reactive({})
const props = defineProps({
parentData:{
type:String,
default: () => '',
},
parentFunction:{
type:Function,
default: () => null,
},
})
onMounted(() => {
//父组件中的属性
console.log(props.parentData);
//父组件中的方法
props.parentFunction();
})
</script>
三、子调父组件中的方法、属性
父组件:parent.vue
<template>
<div class="account-sheet">
<div class="tabs-content">
<!-- 父组件:定义ref属性 -->
<test ref="sonRef" />
</div>
</div>
</template>
<script setup>
import {reactive,getCurrentInstance,computed,onMounted,ref} from 'vue'
import test from './test.vue'
const {proxy} = getCurrentInstance()
const _this = proxy
const theme = computed(() => AppStore.GET_THEME())
const state = reactive({
})
// 定义实体
const sonRef = ref()
onMounted(() => {
//调用子组件中的方法
sonRef.value.myFunction();
//调用子组件中的属性
console.log(sonRef.value.state.sonData)
})
</script>
子组件:test.vue
<script setup>
import {reactive,getCurrentInstance,defineExpose} from 'vue'
const {proxy} = getCurrentInstance()
const _this = proxy
const state = reactive({
sonData:'我是子组件中的属性',
})
const myFunction = ()=>{
console.log('我是子组件中的方法')
}
defineExpose({
myFunction,state
})
</script>
更多推荐
所有评论(0)