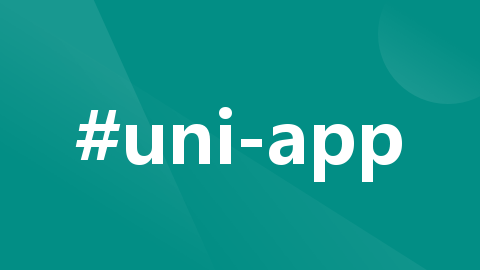
uni-app中使用图表eacharts
uni-app中使用图表eacharts
·
npm install echarts
使用
vue3
配置参考echarts官网
<template>
<view class="ech">
<l-echart ref="chartdom"></l-echart>
</view>
</template>
<style lang="scss">
//设置大小
.ech {
width: 116rpx;
height: 86rpx;
}
</style>
js
import * as echarts from 'echarts'
import {
ref,
reactive,
toRefs,
onMounted
} from 'vue'
import {
onReady,
onLoad,
onShow,
onHide
} from '@dcloudio/uni-app'
let optionEch = reactive({
data: {
animation: true,//折线图动画
toolbox: {
show: true,
feature: {
// dataZoom: {
// yAxisIndex: 'none'
// },
// dataView: { readOnly: false },
// magicType: { type: ['line', 'bar'] },
restore: {},
// saveAsImage: {}//下载我没整好
}
},
title: {
show: true, //false
text: "累计收益走势图", //主标题文本
textStyle: {
fontSize: 12,
}
},
tooltip: {
show: true,
trigger: 'axis',
backgroundColor: "rgba(0,0,0,0.4)",
textStyle: {
color: "#fff",
fontSize: "12",
},
},
legend: {
data: ['单位净值', '累计净值']
},
grid: {
left: 10,
right: 10,
bottom: 55,
top: 40,
containLabel: true
},
xAxis: [{
show: true,
type: 'category',
boundaryGap: false,
data: [1, 2, 3],
axisLabel: {
rotate: 50
}
}],
yAxis: [{
show: true,
axisLine: {
show: true
},
axisTick: {
show: true
},
type: 'value',
axisLabel: {
formatter: function(value, index) {
return value.toFixed(3);
}
},
min: function(value) {
return value.min * 0.999;
},
max: function(value) {
return value.max * 1.002;
},
scale: true, //自适应
}],
dataZoom: [{
type: "slider",
xAxisIndex: 0,
filterMode: "none"
},
{
type: "inside",
xAxisIndex: 0,
filterMode: "none"
}
],
series: [{
name: '单位净值',
type: 'line',
smooth: false,
colorBy: "series",
data: [4, 5, 6],
color: '#00aaff', //改变折线点的颜色
lineStyle: {
color: '#00aaff' //改变折线颜色
},
},
{
name: '累计净值',
type: 'line',
smooth: false,
colorBy: "series",
data: [7, 8, 9],
color: '#c20000', //改变折线点的颜色
lineStyle: {
color: '#c20000' //改变折线颜色
}
},
]
}
})
let chartdom = ref(null)
onMounted(() => {
// 把 echarts 传入
chartdom.value.init(echarts, chartdom => {//初始化调用函数,第一个参数是传入echarts,第二个参数是回调函数,回调函数的参数是 chart 实例
chartdom.setOption(optionEch.data);
})
})
在接口数据请求到之后初始化折线图
onMounted(() => {
// 把 echarts 传入
// chartdom.value.init(echarts, chartdom => {
// chartdom.setOption(optionEch.data);
// })
setTimeout(() => {
fundValuePost();
}, 200)
})
const fundValuePost = () => {
//将图表配置对象复制一份
let chartres = JSON.parse(JSON.stringify(optionEch.data))
chartres.xAxis[0].data = []
chartres.series.forEach((item) => {
item.data = []
})
//调用接口
fundValueApi(fundValuePage).then((res) => {
res.data.data.forEach((item) => {
chartres.xAxis[0].data.push(item.fundValueDate)
chartres.series[0].data.push(item.unitFundValue)
chartres.series[1].data.push(item.accrueFundValue)
})
optionEch.data = JSON.parse(JSON.stringify(chartres));
// 使用请求到的数据,初始化图表
chartdom.value.init(echarts, chartdom => {
chartdom.setOption(optionEch.data);
})
}).catch((err) => {
console.log(err, '收益曲线err')
});
}
一个页面使用多个折线图
<template>
<view class="ech">
<l-echart ref="chartdom"></l-echart>
</view>
<view class="ech">
<l-echart ref="chartdom"></l-echart>
</view>
</template>
js
let chartdom = ref(null)//页面有多个chartdom时,chartdom为数组
onMounted(async () => {
let productGe = await productGetListFun()//获取产品列表productGetListDatas.data
setTimeout(() => {
fundValuePost();
}, 100)
})
let fundValuePage = reactive({
month: "1",
orderBy: "asc",
productId: null
})
const fundValuePost = () => {
let chartArr = []
productGetListDatas.data.forEach((items, indexs) => {//productGetListDatas.data为提前请求拿到的产品列表,然后拿id去请求折线图的数据
let chartres = JSON.parse(JSON.stringify(optionEch.data))
let indexx = indexs
// console.log('chartres',chartres )
chartres.xAxis[0].data = []
chartres.series.forEach((item) => {
item.data = []
})//数据全部清空
fundValuePage.productId = items.productId//拿id去请求折线图的数据
fundValueApi(fundValuePage).then((res) => {
// console.log(indexx, '收益曲线res', res)
res.data.data.forEach((item) => {
chartres.xAxis[0].data.push(item.fundValueDate)
chartres.series[0].data.push(item.accrueFundValue)
})
let objArr = {
ind: indexx,//对应产品数组的index
chartresData: chartres
}
chartArr.push(objArr)//把拿到的所有的折线图数据,全部放入chartArr数组
if (chartArr.length == productGetListDatas.data.length) {
chartArr.forEach((item, index) => {//所有的产品数据全部拿到后,用图表数据的indexx对应产品数组的index,进行遍历,初始化对应图表
chartdom.value[item.ind].init(echarts, chartdom => {
chartdom.setOption(item.chartresData);
})
})
}
}).catch((err) => {
console.log(err, '收益曲线err')
});
})
}
更多推荐
所有评论(0)