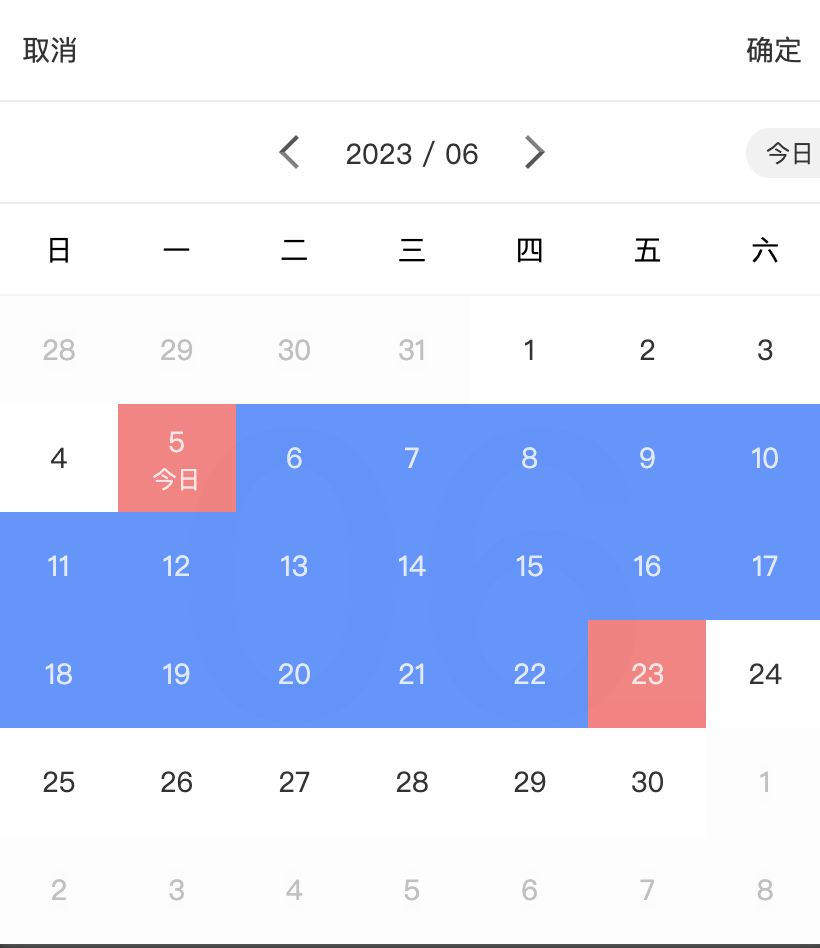
uniapp uni-calender日历查近期还款历史 闰年平年 大小月
uniapp uni-calender日历组件 时间范围变化
·
近期用uniapp开发小程序,有个查询还款历史的需求,度娘了下,始终没有很理想的方法,自己总结归纳写了一个:
思路:
1,要查询几个月的,就在当前日期上,减掉几个月。大方向是这样。
2,细节:相减之后的那年要判断是闰年,平年,Math.min() 方法取值,以防是当月的最后一天,每个月天数不一样,定义上闰年和平年的对象数组。
3,细节:目前需求习惯用 yyyy-mm-dd的形式表达,最好return出的是new Date() 格式,方便转换成任何需要的格式。
不多说,上代码,开干!!!
1,现在工具类untils.js中定义 minusMonths
// 往前推几个月 查询还款, 默认查询 3个月还款
// let uu = new Date(2023,4,31);
// console.log(uu.toLocaleDateString(), 'uu')// 2023/5/31
// let dd = minusMonths(uu, 8) ; //2023/6/2 往前推8个月
// console.log(dd.toLocaleDateString(), 'dddd') // 2022/10/2
// dd.toLocaleDateString().replaceAll("/", "-") // 2022-10-2
export function minusMonths(startDate, months) {
var counts = {
normal: [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31],
leap: [31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
};
console.log(startDate.getMonth() + 1 - months)
var year;
if (startDate.getMonth() + 1 - months > 0) {
year = startDate.getFullYear()
} else {
if (Math.floor(months / 12) == 0) {
year = startDate.getFullYear() - 1
} else {
year = startDate.getFullYear() - Math.floor(months / 12)
}
}
// var year = startDate.getMonth()+1 - months > 0 ? startDate.getFullYear() : startDate.getFullYear() - Math.floor(months / 12)
console.log(year, 'year')
var yearType = ((year % 4 == 0) && (year % 100 != 0)) || (year % 400 == 0) ? 'leap' : 'normal';
var month;
if (startDate.getMonth() + 1 - months > 0) {
month = startDate.getMonth() - months
} else {
if (Math.floor(months / 12) == 0) {
month = startDate.getMonth() - months + 12
} else {
month = startDate.getMonth() - months + 12 * Math.floor(months / 12)
}
}
// var month = startDate.getMonth()+1 - months > 0 ? startDate.getMonth() - months : startDate.getMonth() - months + 12 * Math.floor(months / 12);
console.log(month, 'month')
var date = Math.min(startDate.getDate(), counts[yearType][month]);
return new Date(year, month, date);
}
具体实现:
- .getMonth() 得到的月数和实际月数不一样,打印出来是[0, 1, 2, 3, 4, 5, 6, 7, 8,9,10, 11] ,实际的越是要+1, 判断是否需要跨年,比如当前8月,查询近3个月的历史,8-3,不用跨年,直接8-3 ok;如果需要跨年,跨1年和跨N(N > 1)年又不一样;如果跨1年,Math.floor(months / 12) == 0 year = 当年-1,向上取值为1, 如果跨N年, year = 当年- months / 12向下取值。比如,当前是2023-6-5,查询近39个月的还款历史,正确日期为,2020-3-5 ,年数应该是 2023 - 3 (39 / 12 向下取值), 如下:
if (startDate.getMonth() + 1 - months > 0) {
year = startDate.getFullYear()
} else {
if (Math.floor(months / 12) == 0) {
year = startDate.getFullYear() - 1
} else {
year = startDate.getFullYear() - Math.floor(months / 12)
}
}
- 计算月数,同样道理,跨度为1年,月数要加上12,跨度为N(N > 1)年,要加 12 * months / 12向下取值。
if (startDate.getMonth() + 1 - months > 0) {
month = startDate.getMonth() - months
} else {
if (Math.floor(months / 12) == 0) {
month = startDate.getMonth() - months + 12
} else {
month = startDate.getMonth() - months + 12 * Math.floor(months / 12)
}
}
- 判断闰年、 平年
var yearType = ((year % 4 == 0) && (year % 100 != 0)) || (year % 400 == 0) ? 'leap' : 'normal';
- 哪一天,直接Math.min() 取最小
var date = Math.min(startDate.getDate(), counts[yearType][month]);
return new Date(year, month, date);
- 在 vue 文件中引用
html
<uni-calendar ref="calendar" class="uni-calendar--hook" :clear-date="true"
:insert="info.insert" :range="info.range"
@confirm="confirm" @close="close" />
js部分 注意要用HBuilderX引入uni-calender 插件, 怎么引入插件,自行百度,不多说
import { minusMonths } from "@/utils/utils.js"
let d = new Date()
let beforeDate = minusMonths(d, 3) // 默认查询近3个月历史
let beforeDateStr = beforeDate.toLocaleDateString() // 2023/6/9
let beforeDateStr1 = beforeDate.toLocaleDateString().replaceAll("/", "-") // 2023-6-9
methods中有confirm 函数
confirm(e) {
console.log(e, 'confirm');
// 选择日期 必须从前往后
if(e.range.before == "" || e.range.after == "") {
this.dateText = `${beforeDateStr}` + '~' + `${curDateStr}`
this.begDate = beforeDateStr;
this.endDate = curDateStr;
} else {
let before = new Date(e.range.before.replace(/-/g, '/'))
let after = new Date(e.range.after.replace(/-/g, '/'))
//判断选择的时间先后
if (after > before) {
this.begDate = e.range.before;
this.endDate = e.range.after;
} else {
this.begDate = e.range.after;
this.endDate = e.range.before;
}
this.dateText = this.begDate + '~' + this.endDate;
}
const params = {
openId: uni.getStorageSync('openId'),
pageNum: 1,
pageSize: 20,
begDate: this.begDate.replace(/-/g, ''),
endDate: this.endDate.replace(/-/g, ''),
duebillNum: this.planItem.duebillNum,
}
this.$api.repayHistory(params).then(res => {
if (res.data.code === 200) {
this.list = res.data.data || [];
this.status = res.data.data.length < this.pageSize ? 'nomore' : 'loadmore';
this.pageNum++;
}
})
this.$forceUpdate();
this.show = false;
},
效果图 1 默认查询近3个月
效果图2 点击日期,默认显示当前时间
效果图3 选个时间范围,注意!!!!我先选择的后面日期,又点的前面时间,所以要判断日期先后顺序,见上面代码中有判断!
效果图4 点确定,效果如图
完美结束!
码字不易,请注明链接出处!请多多点赞!https://www.jianshu.com/p/131cde96ed29
更多推荐
所有评论(0)