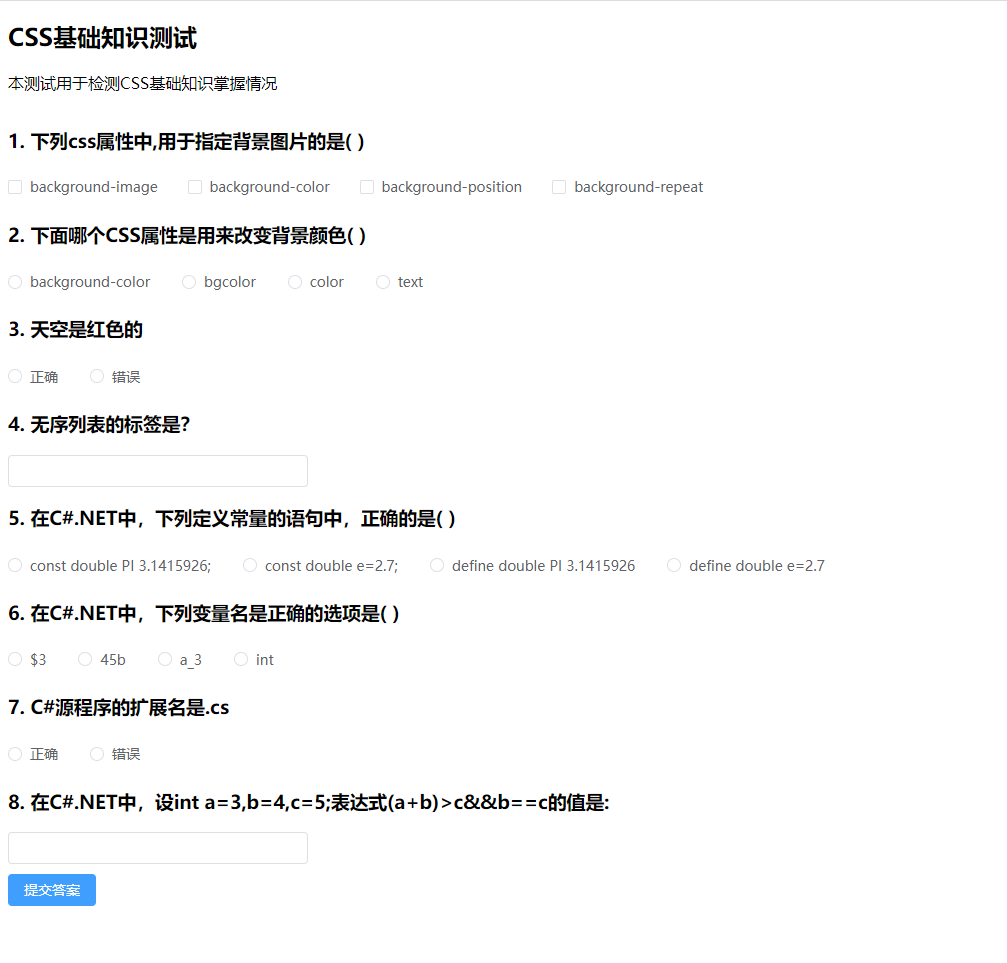
使用vue+element ui完成的考试测试功能Demo
使用vue+element ui完成的考试测试功能Demo根据api传回来的数据渲染试卷页面
·
根据api传回来的数据渲染试卷页面
<template>
<div>
<h2>{{ paper.tName }}</h2>
<p>{{ paper.tDesc }}</p>
<el-form ref="form" :model="answers" label-width="120px">
<!-- <el-row v-for="(question, index) in questions" :key="question.tid"> -->
<el-row v-for="(question, index) in questions" :key="question.tid" :id="'question-' + index">
<el-col :span="24" >
<h3 v-if="question.tType===1">【单选题】{{ index + 1 }}. {{ question.tExplain }}</h3>
<h3 v-else-if="question.tType===4">【多选题】{{ index + 1 }}. {{ question.tExplain }}</h3>
<h3 v-if="question.tType===3">【问答题】{{ index + 1 }}. {{ question.tExplain }}</h3>
<h3 v-if="question.tType===2">【判断题】{{ index + 1 }}. {{ question.tExplain }}</h3>
</el-col>
<el-col :span="24">
<!-- 单选 -->
<el-radio-group v-if="question.tType === 1" v-model="answers[question.tid]">
<el-radio v-for="option in question.topicSelect" :key="option.tselect" :label="option.tselect">
{{ option.topics }}
</el-radio>
</el-radio-group>
<!-- 多选 -->
<el-checkbox-group v-else-if="question.tType === 4" v-model="answers[question.tid]">
<el-checkbox v-for="option in question.topicSelect" :key="option.tselect" :label="option.tselect">
{{ option.topics }}
</el-checkbox>
</el-checkbox-group>
<!-- 问答 -->
<el-input style="width: 300px;" v-else-if="question.tType === 3" v-model="answers[question.tid]"></el-input>
<!-- 判断 -->
<el-radio-group v-else-if="question.tType === 2" v-model="answers[question.tid]">
<el-radio label="true">正确</el-radio>
<el-radio label="false">错误</el-radio>
</el-radio-group>
</el-col>
</el-row>
</el-form>
<el-button style="margin-top: 10px;" type="primary" @click="submitAnswers">提交答案</el-button>
<DatiKa :questions="questions" :answers="answers"></DatiKa>
</div>
</template>
<script>
import DatiKa from './components/datika.vue'
export default {
data() {
return {
// 试卷基础信息
paper: {
tName: 'CSS基础知识测试',
tDesc: '本测试用于检测CSS基础知识掌握情况'
},
//题目,通过axios获取,可以直接添加数组
questions: [],
// 双向绑定答案
answers: {}
}
},
components:{
//注册答题卡子组件
DatiKa
},
created() {
//获取题目数据方法
this.fetchPaper()
},
methods: {
//axios获取后台api接口
async fetchPaper() {
const { data: res } = await this.$http.get('/api/Home/GTY')
if (res.status !== 200) return this.$message.error('数据请求失败')
this.questions = res.data
console.log(this.questions)
},
//提交答案方法
//将答案格式转化成一个数组,其中有两个属性anid(对应的是题目的tid),daan(选择或输入的答案)
submitAnswers() {
const answersArray = []
for (const [anid, daan] of Object.entries(this.answers)) {
answersArray.push({ anid, daan })
}
console.log(answersArray)
}
}
}
</script>
创建一个答题卡子组件并在父组件注册
<DatiKa :questions="questions" :answers="answers"></DatiKa>
components:{
DatiKa
}
根据父组件传递的值渲染答题卡子组件
<template>
<div class="answer-sheet">
<div class="answer-sheet-header">答题卡</div>
<div class="answer-sheet-content">
<ul>
<li
v-for="(question, index) in questions"
:key="question.tid"
:class="{ 'answered': answers[question.tid] !== undefined && answers[question.tid].length > 0 }"
@click="scrollToQuestion(index)">
{{ index + 1 }}
</li>
</ul>
</div>
</div>
</template>
<script>
export default {
props: {
questions: {
type: Array,
default: () => []
},
answers: {
type: Object,
default: () => ({})
}
},
methods: {
scrollToQuestion(index) {
const questionEl = document.getElementById(`question-${index}`)
if (questionEl) {
questionEl.scrollIntoView({ behavior: 'smooth' })
}
}
}
}
</script>
<style scoped>
.answer-sheet {
position: fixed;
top: 20px;
right: 20px;
border: 1px solid #ddd;
background-color: #fff;
padding: 10px;
border-radius: 4px;
}
.answer-sheet-header {
font-weight: bold;
margin-bottom: 10px;
}
.answer-sheet-content {
max-height: 400px;
overflow: auto;
}
.answer-sheet-content ul {
list-style: none;
padding: 0;
margin: 0;
}
.answer-sheet-content ul li {
display: inline-block;
width: 30px;
height: 30px;
border-radius: 50%;
text-align: center;
line-height: 30px;
margin-right: 10px;
margin-bottom: 10px;
cursor: pointer;
}
api返回的题目数据示例
{
"status": 200,
"data": [
{
"tid": 1,
"tExplain": "下列css属性中,用于指定背景图片的是( )",
"tScore": 25,
"tType": 4,
"tSort": 1,
"tAnswer": "A,B,C,D",
"tpaperId": 1,
"topicSelect": [
{
"tselect": "A",
"topics": "background-image"
},
{
"tselect": "B",
"topics": "background-color"
},
{
"tselect": "C",
"topics": "background-position"
},
{
"tselect": "D",
"topics": "background-repeat"
}
]
},
{
"tid": 2,
"tExplain": "下面哪个CSS属性是用来改变背景颜色( )",
"tScore": 25,
"tType": 1,
"tSort": 1,
"tAnswer": "A",
"tpaperId": 1,
"topicSelect": [
{
"tselect": "A",
"topics": "background-color"
},
{
"tselect": "B",
"topics": "bgcolor"
},
{
"tselect": "C",
"topics": "color"
},
{
"tselect": "D",
"topics": "text"
}
]
},
{
"tid": 3,
"tExplain": "天空是红色的",
"tScore": 25,
"tType": 2,
"tSort": 2,
"tAnswer": "0",
"tpaperId": 1,
"topicSelect": [
{
"tselect": "A",
"topics": ""
},
{
"tselect": "B",
"topics": ""
},
{
"tselect": "C",
"topics": ""
},
{
"tselect": "D",
"topics": ""
}
]
},
{
"tid": 4,
"tExplain": "无序列表的标签是?",
"tScore": 25,
"tType": 3,
"tSort": 3,
"tAnswer": "<ul><li>",
"tpaperId": 1,
"topicSelect": [
{
"tselect": "A",
"topics": ""
},
{
"tselect": "B",
"topics": ""
},
{
"tselect": "C",
"topics": ""
},
{
"tselect": "D",
"topics": ""
}
]
},
{
"tid": 5,
"tExplain": "在C#.NET中,下列定义常量的语句中,正确的是( )",
"tScore": 25,
"tType": 1,
"tSort": 1,
"tAnswer": "B",
"tpaperId": 2,
"topicSelect": [
{
"tselect": "A",
"topics": "const double PI 3.1415926;"
},
{
"tselect": "B",
"topics": "const double e=2.7;"
},
{
"tselect": "C",
"topics": "define double PI 3.1415926"
},
{
"tselect": "D",
"topics": "define double e=2.7"
}
]
},
{
"tid": 6,
"tExplain": "在C#.NET中,下列变量名是正确的选项是( )",
"tScore": 25,
"tType": 1,
"tSort": 1,
"tAnswer": "C",
"tpaperId": 2,
"topicSelect": [
{
"tselect": "A",
"topics": "$3"
},
{
"tselect": "B",
"topics": "45b"
},
{
"tselect": "C",
"topics": "a_3"
},
{
"tselect": "D",
"topics": "int"
}
]
},
{
"tid": 7,
"tExplain": "C#源程序的扩展名是.cs",
"tScore": 25,
"tType": 2,
"tSort": 2,
"tAnswer": "1",
"tpaperId": 2,
"topicSelect": [
{
"tselect": "A",
"topics": ""
},
{
"tselect": "B",
"topics": ""
},
{
"tselect": "C",
"topics": ""
},
{
"tselect": "D",
"topics": ""
}
]
},
{
"tid": 8,
"tExplain": "在C#.NET中,设int a=3,b=4,c=5;表达式(a+b)>c&&b==c的值是:",
"tScore": 25,
"tType": 3,
"tSort": 3,
"tAnswer": "false",
"tpaperId": 2,
"topicSelect": [
{
"tselect": "A",
"topics": ""
},
{
"tselect": "B",
"topics": ""
},
{
"tselect": "C",
"topics": ""
},
{
"tselect": "D",
"topics": ""
}
]
}
],
"msg": "查询成功"
}
最后的效果展示
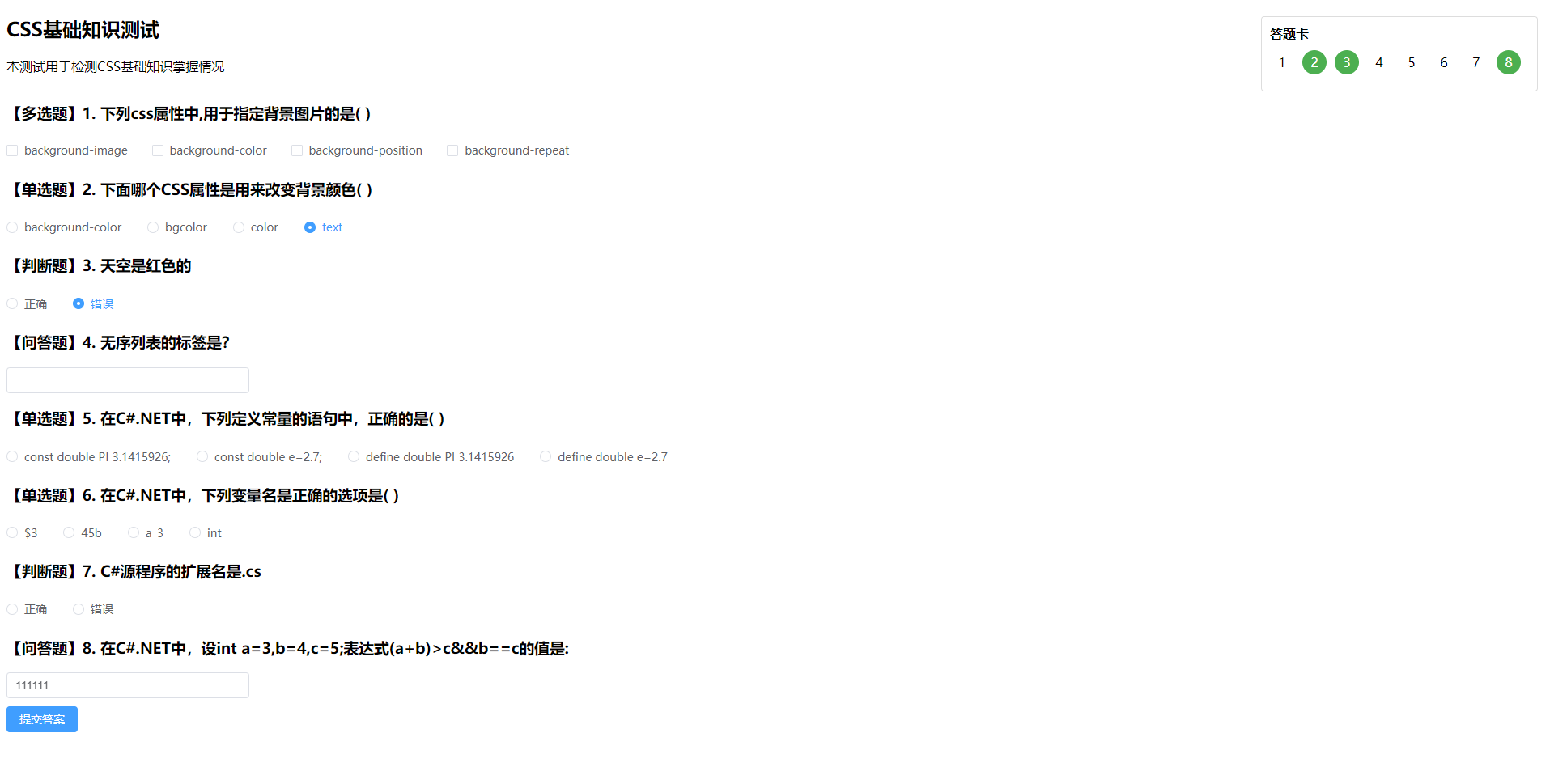
更多推荐
所有评论(0)