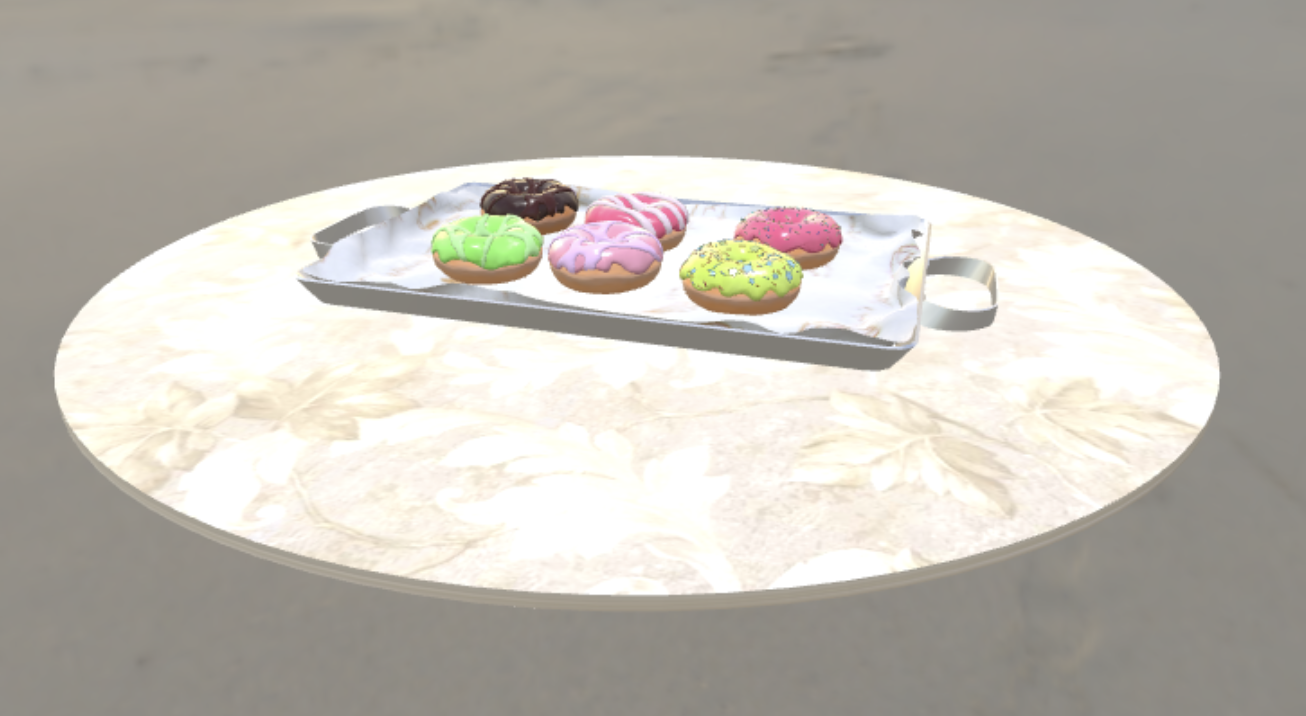
tree.js初体验 | 大帅老猿threejs特训
之前对Web3D并不了解,最近跟着大帅的训练营,通过三天的学习,了解了blender的操作和three.js。下面就用几十行js代码实现一个带动态效果的甜甜圈。

一键AI生成摘要,助你高效阅读
问答
·
之前对Web3D并不了解,最近跟着大帅的训练营,通过三天的学习,了解了blender的操作和three.js。下面就用几十行js代码实现一个带动态效果的甜甜圈。
准备
模型获取:www.sketchfab.com
模型处理:blender
tree.js基础api
基础场景
scene:场景(容器)。`new THREE.Scene()`
camera:相机,决定了在场景中能看到什么。`new THREE.PerspectiveCamera( 75, window.innerWidth / window.innerHeight, 0.1, 1000 )`
renderer:渲染器。`new THREE.WebGLRenderer()`
灯光
AmbientLight:环境光
DirectionalLight:方向光
PointLight:点光源(电灯泡)
SpotLight:聚光灯(手电筒、汽车灯)
Hemisphere:Light:半球光
材质
MeshBasicMaterial:基础材质(测试调试用得多)
MeshStandardMaterial:PBR材质(写实项目标配材质)
几何体
BoxGeometry:立方体
SphereGeometry:球体
CylinderGeometry:圆柱体
创建几何体
const geometry = new THREE.BoxGeometry( 1, 1, 1 );
const material = new THREE.MeshBasicMaterial( {color: 0x00ff00} );
const cube = new THREE.Mesh( geometry, material );
scene.add( cube );
GLTFLoader:加载gltf/glb模型
new GLTFLoader().load('../resources/models/donuts.glb', (gltf) => {
scene.add(gltf.scene);
}
RGBELoader:加载环境光HDR图片
new RGBELoader()
.load('../resources/sky.hdr', function (texture) {
texture.mapping = THREE.EquirectangularReflectionMapping;
scene.environment = texture;
renderer.outputEncoding = THREE.sRGBEncoding;
renderer.render(scene, camera);
});
效果
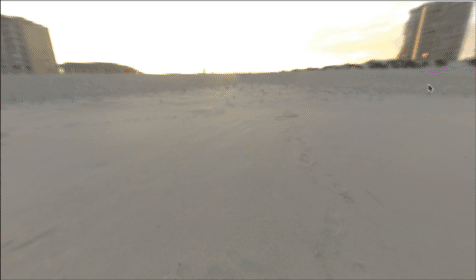
实现
1.初始化
let mixer;
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.01, 10);
const renderer = new THREE.WebGLRenderer({ antialias: true });
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// camera初始化位置
camera.position.set(1, 0.3, 0.5);
// 创建控件对象
const controls = new OrbitControls(camera, renderer.domElement);
// 场景添加灯光
const directionLight = new THREE.DirectionalLight(0xffffff, 0.7);
scene.add(directionLight);
2.加载甜甜圈模型
let donuts;
new GLTFLoader().load('../resources/models/donuts.glb', (gltf) => {
scene.add(gltf.scene); // 添加到场景中
donuts = gltf.scene;
mixer = new THREE.AnimationMixer(gltf.scene); // 将模型作为参数创建一个混合器
const clips = gltf.animations; // 播放所有动画
clips.forEach(function (clip) {
const action = mixer.clipAction(clip);
action.loop = THREE.LoopOnce;
action.clampWhenFinished = true; // 将甜甜圈落下动画停在最后一帧
action.play();
});
})
3. 开启requestAnimationFrame更新动画
function animate() {
requestAnimationFrame(animate);
renderer.render(scene, camera);
controls.update();
if (donuts){
donuts.rotation.y += 0.005;
}
if (mixer) {
mixer.update(0.02);
}
}
animate();
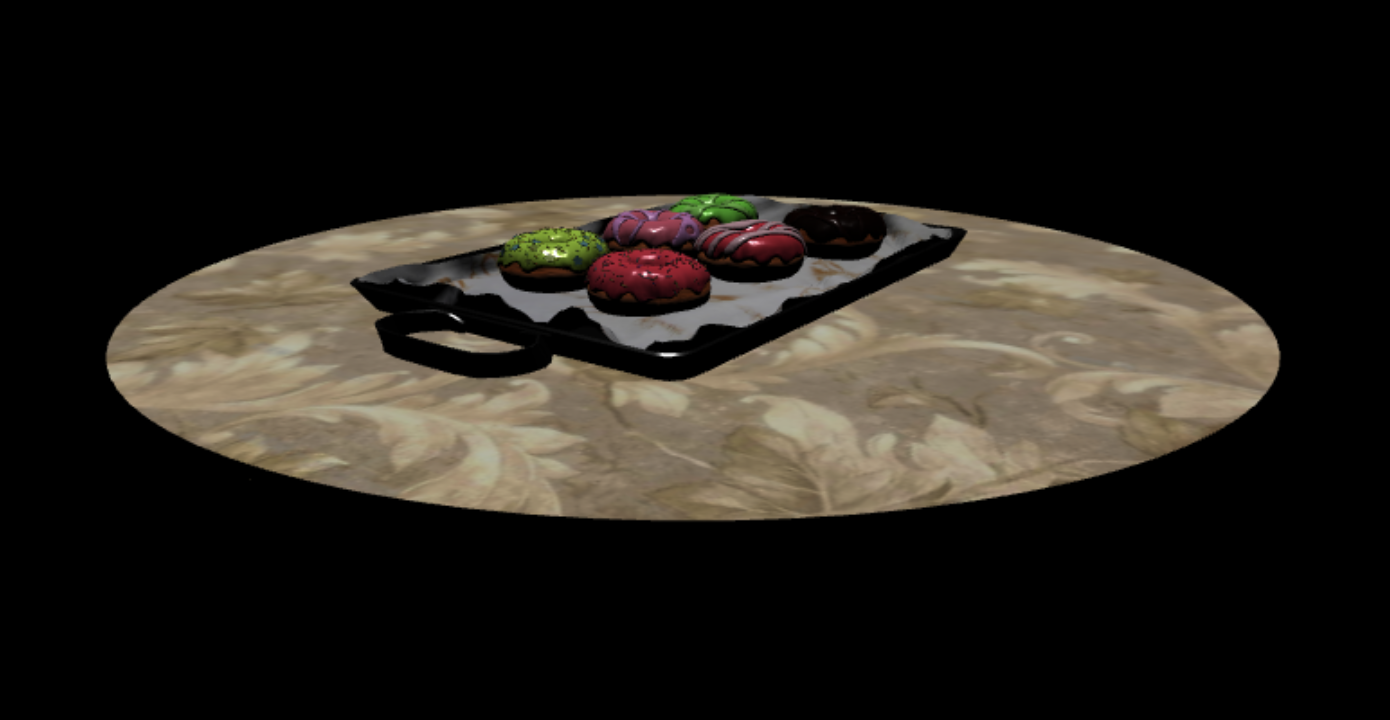
这时甜甜圈的动画已经有了,但是背景是黑色的,盘子的金属材质反射黑色的光,接下来加载一个天空的全景图片,通过它的光让反射光更自然
4.加载天空模型
new RGBELoader()
.load('../resources/sky.hdr', function (texture) {
scene.background = texture;
texture.mapping = THREE.EquirectangularReflectionMapping;
scene.environment = texture;
renderer.outputEncoding = THREE.sRGBEncoding;
renderer.render(scene, camera);
});
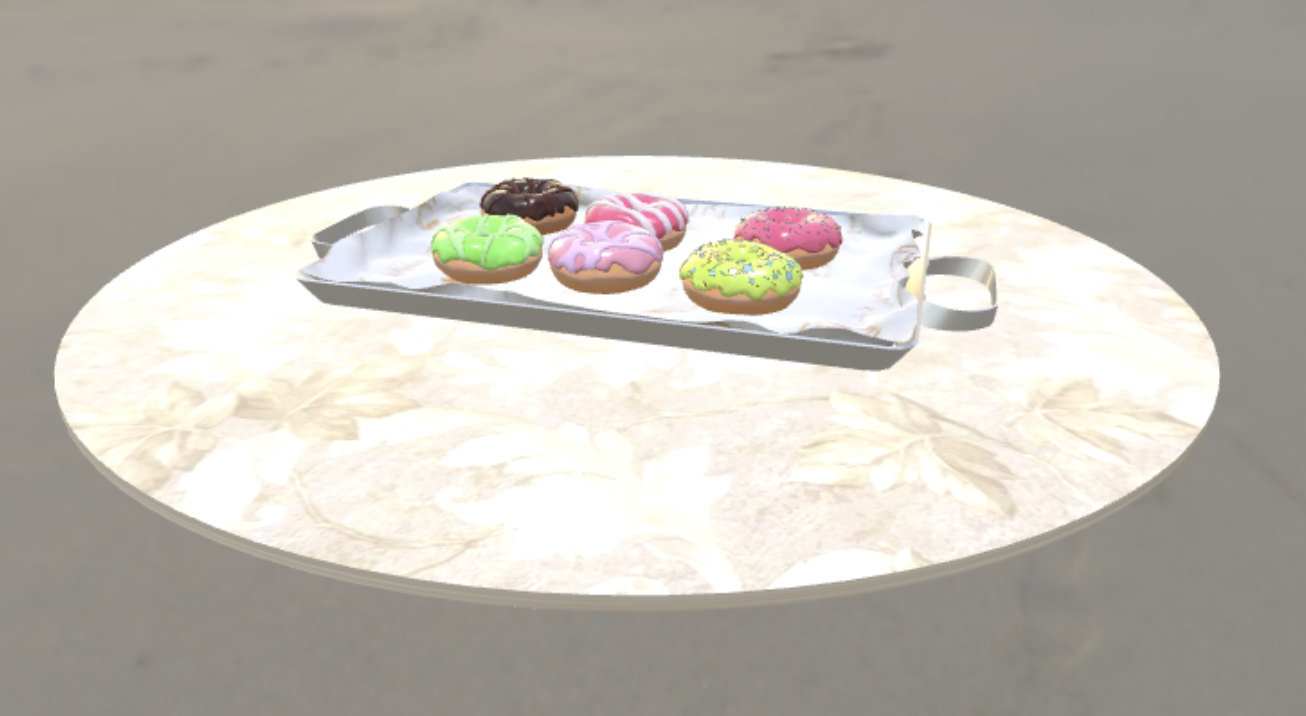
完成!
课程源码仓库地址,自己试一下吧!加入猿创营 (v:dashuailaoyuan),一起交流学习
更多推荐
所有评论(0)