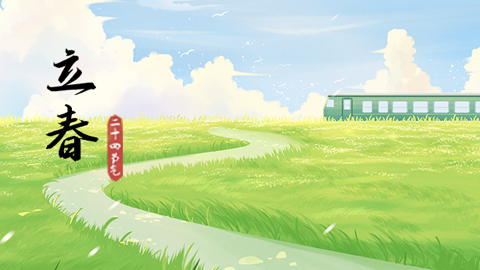
每天记录学习的新知识:CRC16
当接收到数据时,接收器可以使用相同的算法计算自己的校验和,并将其与接收到的数据一起进行比较。如果两个校验和匹配,数据被认为是无误的。CRC16(16位循环冗余校验)是一种用于检测数字数据中错误的编码方式。它通过对一个数据块(通常是消息或信息包)生成一个校验和,然后将其附加到数据的末尾来实现。该算法将数据视为一系列位,并对该序列执行位分割运算,使用多项式进行除法运算。这个除法的余数就是CRC16校验

一键AI生成摘要,助你高效阅读
问答
·
一、CRC 16
CRC16(16位循环冗余校验)是一种用于检测数字数据中错误的编码方式。它通过对一个数据块(通常是消息或信息包)生成一个校验和,然后将其附加到数据的末尾来实现。这个校验和是使用一种数学公式计算得出的16位值。
CRC16算法使用16次方的多项式来生成校验和。该算法将数据视为一系列位,并对该序列执行位分割运算,使用多项式进行除法运算。这个除法的余数就是CRC16校验和,它被附加到原始数据的末尾。
CRC16常用于通信协议中,例如Modbus,以确保传输的数据完整性。当接收到数据时,接收器可以使用相同的算法计算自己的校验和,并将其与接收到的数据一起进行比较。如果两个校验和匹配,数据被认为是无误的。如果它们不匹配,就假定在传输过程中发生了错误,数据可能需要重新传输。
CRC16算法有几种变体,每种变体使用不同的多项式。最常见的变体是CRC-16-CCITT和CRC-16-ANSI。
二、在线工具
http://www.ip33.com/crc.html
三、CRC-16/CCITT-FALSE
/**
*
* CRC数组处理工具类及数组合并
*/
public class CrcUtil {
/**
* 为Byte数组最后添加两位CRC校验
*
* @param buf(验证的byte数组)
* @return
*/
public static byte[] setParamCRC(byte[] buf) {
int checkCode = 0;
checkCode = crc_16_CCITT_False(buf, buf.length);
byte[] crcByte = new byte[2];
crcByte[0] = (byte) ((checkCode >> 8) & 0xff);
crcByte[1] = (byte) (checkCode & 0xff);
// 将新生成的byte数组添加到原数据结尾并返回
return concatAll(buf, crcByte);
}
/**
* CRC-16/CCITT-FALSE x16+x12+x5+1 算法
*
* info
* Name:CRC-16/CCITT-FAI
* Width:16
* Poly:0x1021
* Init:0xFFFF
* RefIn:False
* RefOut:False
* XorOut:0x0000
*
* @param bytes
* @param length
* @return
*/
public static int crc_16_CCITT_False(byte[] bytes, int length) {
int crc = 0xffff; // initial value
int polynomial = 0x1021; // poly value
for (int index = 0; index < bytes.length; index++) {
byte b = bytes[index];
for (int i = 0; i < 8; i++) {
boolean bit = ((b >> (7 - i) & 1) == 1);
boolean c15 = ((crc >> 15 & 1) == 1);
crc <<= 1;
if (c15 ^ bit)
crc ^= polynomial;
}
}
crc &= 0xffff;
//输出String字样的16进制
String strCrc = Integer.toHexString(crc).toUpperCase();
System.out.println(strCrc);
return crc;
}
/***
* CRC校验是否通过
*
* @param srcByte
* @param length(验证码字节长度)
* @return
*/
public static boolean isPassCRC(byte[] srcByte, int length) {
// 取出除crc校验位的其他数组,进行计算,得到CRC校验结果
int calcCRC = calcCRC(srcByte, 0, srcByte.length - length);
byte[] bytes = new byte[2];
bytes[0] = (byte) ((calcCRC >> 8) & 0xff);
bytes[1] = (byte) (calcCRC & 0xff);
// 取出CRC校验位,进行计算
int i = srcByte.length;
byte[] b = { srcByte[i - 2] ,srcByte[i - 1] };
// 比较
return bytes[0] == b[0] && bytes[1] == b[1];
}
/**
* 对buf中offset以前crcLen长度的字节作crc校验,返回校验结果
* @param buf
* @param crcLen
*/
private static int calcCRC(byte[] buf, int offset, int crcLen) {
int start = offset;
int end = offset + crcLen;
int crc = 0xffff; // initial value
int polynomial = 0x1021;
for (int index = start; index < end; index++) {
byte b = buf[index];
for (int i = 0; i < 8; i++) {
boolean bit = ((b >> (7 - i) & 1) == 1);
boolean c15 = ((crc >> 15 & 1) == 1);
crc <<= 1;
if (c15 ^ bit)
crc ^= polynomial;
}
}
crc &= 0xffff;
return crc;
}
/**
* 多个数组合并
*
* @param first
* @param rest
* @return
*/
public static byte[] concatAll(byte[] first, byte[]... rest) {
int totalLength = first.length;
for (byte[] array : rest) {
totalLength += array.length;
}
byte[] result = Arrays.copyOf(first, totalLength);
int offset = first.length;
for (byte[] array : rest) {
System.arraycopy(array, 0, result, offset, array.length);
offset += array.length;
}
return result;
}
}
更多推荐
所有评论(0)