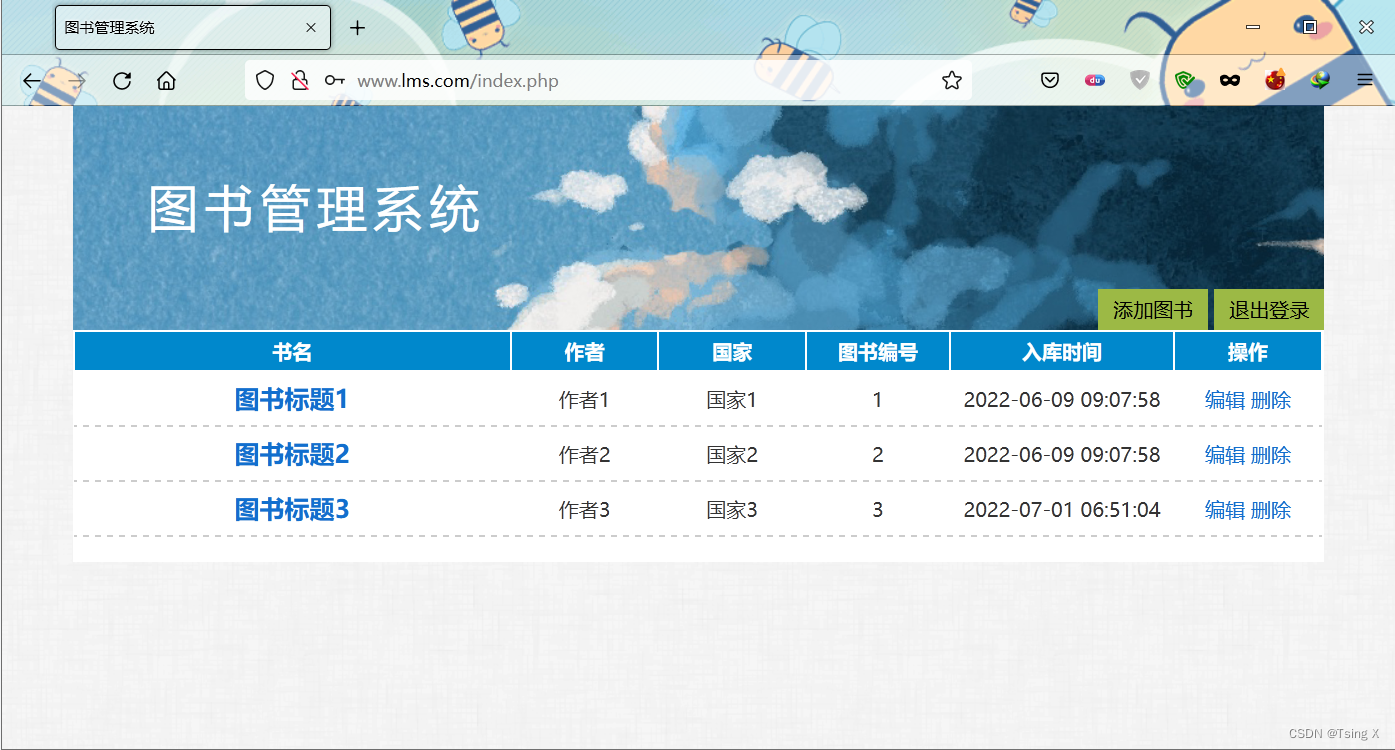
PHP+MySQL制作简单动态网站(附详细注释+源码)
项目名称:图书管理系统;项目实现的内容用户登录、用户注册、登录界面验证码功能、退出登录功能、内容查看、内容添加、内容修改。根目录布置。

一键AI生成摘要,助你高效阅读
问答
·
项目介绍
项目名称:图书管理系统
项目实现的内容:
1.用户登录、用户注册、登录界面验证码功能。
2.退出登录功能、内容查看、内容添加、内容修改。
前端页面设计得有点可能不太专业,将就着用。主要专注在功能的实现。
内容页
添加内容
删除内容
修改内容
具体实现步骤
根目录布置:
1.登录界面实现
具体步骤参照文章:
PHP+MySQL制作简单的用户注册登录界面(注释超详细~附源代码)_Tsing X的博客-CSDN博客
2.内容页实现
*注意,请完成登录界面后再进行后续操作
1.1创建index.html
用于显示内容页
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>图书管理系统</title>
<link rel="stylesheet" href="../css/style.css"/>
</head>
<body>
<div class="box">
<div class="top">
<div class="title">图书管理系统</div>
<div class="nav">
<a href="../add.php">添加图书</a>
<a href="../logout.php">退出登录</a>
</div>
</div>
<div class="main">
<table class="lib-list">
<tr>
<th>书名</th>
<th width="200">作者</th>
<th width="200">国家</th>
<th width="200">图书编号</th>
<th width="250">入库时间</th>
<th width="200">操作</th>
</tr>
<?php foreach($data as $v): ?>
<tr>
<td class="lib-title">
<a href="../show.php?id=<?php echo $v['id'];?>"><?php echo $v['title'];?></a>
</td>
<td class="center"><?php echo $v['author'];?></td>
<td class="center"><?php echo $v['country'];?></td>
<td class="center"><?php echo $v['id'];?></td>
<td class="center"><?php echo $v['addtime'];?></td>
<td class="center">
<a href="../edit.php?id=<?php echo $v['id'];?>">编辑</a>
<a href="../del.php?id=<?php echo $v['id'];?>" onclick="return confirm('确定删除?');" >删除</a>
</td>
</tr>
<?php endforeach;?>
</table>
</div>
</div>
</body>
</html>
1.2创建index.php关联index.html
实现对内容数据的获取,执行数据的查询操作
<?php
//主页
require './init_login.php';//验证是否有登录
require 'info_db_connect.php';//连接数据库
$sql='select id,title,author,country,addtime from info order by id asc';
//执行查询语句,查询结果集存储在对象$stmt中
$stmt = $pdo->query($sql);
//从stmt中取出查询结果,并保存在$data中
$data=$stmt->fetchAll(PDO::FETCH_ASSOC);
require './view/index.html';
?>
2.1创建info_db_connect.php
用于连接数据库
<?php
//用于管理界面的数据库的连接
//设置DSN数据源
$dsn = 'mysql:host=localhost;dbname=LMS;charset=utf8';//注意,此处的LMS为自定义的数据库名,你创建什么数据库名就将LMS改成它就行了
//连接数据库
try {
$pdo = new PDO($dsn,'root','Huawei@123');//通过pdo连接数据源,此处root为MySQl的登录用户名,其后是登录密码
}catch (PDOException $e){
echo 'error--'.$e->getMessage();
}
3.1创建add.html
添加内容的界面实现过程
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>图书管理系统</title>
<link rel="stylesheet" href="../css/style.css"/>
</head>
<body>
<div class="box">
<div class="top">
<div class="title">图书管理系统</div>
<div class="nav">
<a href="index.php">返回</a>
</div>
</div>
<div class="main">
<form action="../add.php" method="post">
<table class="lib-edit">
<tr>
<th>图书名称:</th>
<td><input type="text" name="title" placeholder="填写图书标题..." /></td>
</tr>
<tr>
<tr>
<th>作者姓名:</th>
<td><input type="text" name="author" placeholder="填写作者名..." /></td>
</tr>
<tr>
<th>作者国籍:</th>
<td><input type="text" name="country" placeholder="填写作者国籍..." /></td>
</tr>
<tr>
<th>图书简介:</th>
<td><textarea name="content" placeholder="填写图书简介..."></textarea></td>
</tr>
<tr>
<th></th>
<td><input type="submit" value="提交入库登记" /></td>
</tr>
</table>
</form>
</div>
</div>
</body>
</html>
3.2创建add.php关联add.html
在验证用户为登录状态后,执行数据的插入工作
<?php
require './init_login.php';//验证是否有登录
if (!empty($_POST)){//用户提交了表单
//获取表单中输入的数据
$data = array();//用于存储表单中输入的数据的数组
$data['title']=trim(htmlspecialchars($_POST['title']));//存储书名
$data['author']=trim(htmlspecialchars($_POST['author']));//存储作者名
$data['country']=trim(htmlspecialchars($_POST['country']));//存储国籍
$data['content']=trim(htmlspecialchars($_POST['content']));//存储简介
//连接数据库
require 'info_db_connect.php';
$sql='insert into info(title,author,country,content) values(:title,:author,:country,:content)';
$stmt=$pdo->prepare($sql);//预编译sql语句
$stmt->execute($data);//执行插入数据的sql语句
header('Location:./index.php');//重定向到主页面
}
require './view/add.html';
4.1创建edit.html
编辑界面实现过程
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>图书管理系统</title>
<link rel="stylesheet" href="../css/style.css"/>
</head>
<body>
<div class="box">
<div class="top">
<div class="title">图书管理系统</div>
<div class="nav">
<a href='index.php'>返回</a>
</div>
</div>
<div class="main">
<form method="post">
<table class="lib-edit">
<tr>
<th>图书名称:</th>
<td><input type="text" name="title" value="<?php echo $data['title'];?>"/></td>
</tr>
<tr>
<th>作者姓名:</th>
<td><input type="text" name="author" value="<?php echo $data['author'];?>"/></td>
</tr>
<tr>
<th>作者国籍:</th>
<td><input type="text" name="country" value="<?php echo $data['country'];?>"/></td>
</tr>
<tr>
<th>图书简介:</th>
<td><textarea name="content"><?php echo $data['content'];?></textarea></td>
</tr>
<tr>
<th></th>
<td><input type="submit" value="提交修改" /></td>
</tr>
</table>
</form>
</div>
</div>
</body>
</html>
4.2创建edit.php,关联edit.html
在验证完用户为登录状态后,执行修改数据库的相关操作
<?php
//用于编辑内容
require './init_login.php';//验证是否有登录
require 'info_db_connect.php';//连接数据库
$id=isset($_GET['id'])?(int)$_GET['id']:0;//获取get传参id值
$data=array('id'=>$id);//将id值放到data数组中
$sql='select title,author,country,content,addtime from info where id=:id';//:id占位符
$stmt=$pdo->prepare($sql);//对于查询语句进行编译PDOStatement对象
if (!$stmt->execute($data)){//执行查询语句
exit('查询失败'.implode(' ', $stmt->errorInfo()));//输出查询失败原因
}
$data = $stmt->fetch(PDO::FETCH_ASSOC);//将查询结果存储在数组data中
if(empty($data)){
echo ('新闻id不存在');
}
//数据修改
if (!empty($_POST)){
// var_dump($data);
$id=isset($_GET['id'])?(int)$_GET['id']:0;//获取get传参id值
$data=array('id'=>$id);//将id值放到data数组中
$data = array();//用于存储表单中输入的数据的数组
$data=array('id'=>$id);//将id值放到data数组中
$data['title']=trim(htmlspecialchars($_POST['title']));//存储图书名称
$data['author']=trim(htmlspecialchars($_POST['author']));//存储作者名
$data['country']=trim(htmlspecialchars($_POST['country']));//存储国家名称
$data['content']=trim(htmlspecialchars($_POST['content']));//存储图书简介
// print_r($data);
//将数据写入到数据库中(update)
$sql='update `info` set title=:title,author=:author,country=:country,content=:content where id=:id';
$stmt=$pdo->prepare($sql);//预编译sql语句
$stmt->execute($data);//执行插入数据的sql语句
}
require './view/edit.html';
5.创建del.php
用于实现删除内容的操作,通过get值准确定位到要执行操作的数据
<?php
//用于删除内容
require './init_login.php';//验证是否有登录
require 'info_db_connect.php';//连接数据库
$id=isset($_GET['id'])?(int)$_GET['id']:0;//获取get传参id值
$data=array('id'=>$id);//将id值放到data数组中
//删除数据的sql语句
$sql='delete from info where id=:id';
//预处理
$stmt=$pdo->prepare($sql);
//执行sql语句
if (!$stmt->execute($data)){
exit('删除失败'.implode('-', $stmt->errorInfo()));
}
//重定向到主页面
header('Location:index.php');
6.1创建show.html
用于实现展示详细内容的功能
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>图书管理系统</title>
<link rel="stylesheet" href="../css/style.css"/>
</head>
<body>
<div class="box">
<div class="top">
<div class="title">图书管理系统</div>
<div class="nav">
<a href="index.php">返回</a>
</div>
</div>
<div class="main">
<div class="lib-title"><?php echo $data['title']?></div>
<div class="lib-time">【<?php echo $data['country'];?>】 <?php echo $data['author'];?></div>
<div class="lib-time">入库时间:<?php echo $data['addtime'];?> 编号:<?php echo $data['id'];?></div>
<div class="lib-content"><?php echo $data['content']?></div>
</div>
</div>
</body>
</html>
6.2创建show.php关联show.html
执行的操作有连接数据库,查询数据,对传参进行判断。
<?php
//内容详情页
require './init_login.php';//判断是否登录
require 'info_db_connect.php';//连接数据库
$id=isset($_GET['id'])?(int)$_GET['id']:0;//获取get传参id值
$data=array('id'=>$id);//将id值放到data数组中
$sql='select id,title,content,author,country,addtime from info where id=:id';//:id占位符
$stmt=$pdo->prepare($sql);//对于查询语句进行编译PDOStatement对象
if (!$stmt->execute($data)){//执行查询语句
exit('查询失败'.implode(' ', $stmt->errorInfo()));//输出查询失败原因
}
$data = $stmt->fetch(PDO::FETCH_ASSOC);//将查询结果存储在数组data中
if(empty($data)){
echo ('编号不存在');
}
require './view/show.html';
7.创建init_login.php
用于判断用户是否登录,若未登录则跳转登录界面,提升网站安全性
<?php
//验证是否有登录,无登录则跳转登录界面
//启动session
session_start();
if (!isset($_SESSION['username'])){
header('Location:login.php');
exit;
}
8.创建logout.php
依靠关闭session来实现退出登录的功能。
<?php
//用于退出登录
session_start();
//删除session
unset($_SESSION['username']);
//跳转登录界面
header('location:login.php');
*注意:篇幅有限,具体详见源代码,仅供学习参考,转载请注明出处。感谢支持。
源代码下载:
https://pan.quark.cn/s/7223b1e8b27a
提取码:SVw5
更多推荐
所有评论(0)