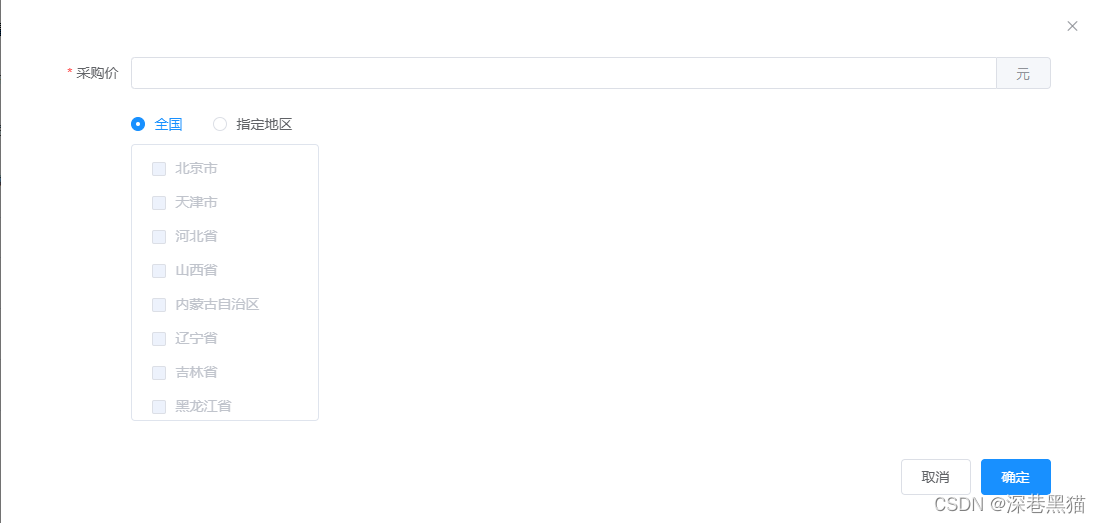
vue+element 全国+指定地区 地区级联选择器
1.实现效果(全国禁选,指定地区可选)2. 思路(级联选择器无法直接添加禁用,只能子地区数据里添加disabled=true)1.单选组<el-radio-group v-model="radio"><el-radio :label="1" @change="allCountryChangeHandle">全国</el-radio><el-radio :l
·
1.实现效果(全国禁选,指定地区可选)
2. 思路(级联选择器无法直接添加禁用,只能子地区数据里添加disabled=true)
1.单选组
<el-radio-group v-model="radio">
<el-radio :label="1" @change="allCountryChangeHandle">全国</el-radio>
<el-radio :label="2" @change="allCountryChangeHandle">指定地区</el-radio>
</el-radio-group>
2. 全国----只给第一层数据
指定地区---给三层数据
methods:{
init() {
// 获取地区
getRegionList(this.level).then(({ data }) => {
let dataBuild = data
if (this.showAllCountry) {
dataBuild = [{
d: '0',
h: '全国',
c: data
}
]
}
// this.regionList = dataBuild
// 获取三级地区数据
this.regionListBase = dataBuild
// console.log(data, 'data')
// 将一级地区数据赋值给level1
const level1 = data.map(item => {
return { d: item.d, h: item.h }
})
// 给每一条以及地区数据里添加disbled=true
level1.map((item) => {
this.$set(item, 'disabled', true)
})
// console.log(level1)
// 将处理好的level1 赋值
this.regionListLevet1 = level1
if (this.radio === 1) {
// 当时全国时只赋值一级地区数据切禁用
this.regionList = this.regionListLevet1
} else {
// 指定地区时赋值三级地区数据不禁用
this.regionList = this.regionListBase
}
})
}
}
3.单选组切换(使用element radio的事件change)
allCountryChangeHandle(val) {
if (val === 1) {
//已选择地区列表清空
this.chooseList = []
//cascader里的值清空(不清空会影响切换)
this.valueRegion = []
//在获取到数据后进行赋值
this.$nextTick(() => {
//将一级禁用地区列表赋值
this.regionList = this.regionListLevet1
})
} else if (val === 2) {
this.$nextTick(() => {
this.regionList = this.regionListBase
})
}
this.regionIsShow = !val
//单选时
if (this.multiple === false) {
this.valueRegion = undefined
//如果选中的是全国,已选中地区列表为 d: 0, path: '全国'
if (val === 1) {
this.chooseList = [{ d: 0, path: '全国' }]
this.$emit('isAllCountry')
console.log('this.allCountry is true')
} else {
this.chooseList = []
// this.$emit('noAllCountry');
console.log('this.allCountry is false')
}
// console.log('this.chooseList',this.chooseList);
this.$emit('update:chooseData', this.chooseList)
} else {
this.changeHandle()
}
}
3.完整组件代码
<template>
<div>
<!-- {{ valueRegion }} -->
<!-- {{ chooseList }}
{{ allCountry }} -->
<!-- <el-checkbox
v-show="true"
v-model="allCountry"
@change="allCountryChangeHandle"
>全国</el-checkbox> -->
<el-radio-group v-model="radio">
<el-radio :label="1" @change="allCountryChangeHandle">全国</el-radio>
<el-radio :label="2" @change="allCountryChangeHandle">指定地区</el-radio>
</el-radio-group>
<div style="margin-bottom: 5px" />
<!-- {{ valueRegion }} -->
<el-cascader-panel
v-show="hideRegion"
ref="consigneeRef"
v-model="valueRegion"
class="consref-panel"
:options="regionList"
:props="regionProps"
:placeholder="placeholder"
:size="size"
@change="changeHandle"
>
<!-- <template slot-scope="{ node, data }">
<span v-show="node.hasChecked||data.p" class="hidden-check">
<span class="hidden-check-inside" />
</span>
{{ data.h }}
</template> -->
</el-cascader-panel>
</div>
</template>
<script>
import { getRegionList } from '@/api/region'
// import { getList } from '@/api/region'
// import { getPathItem, getParentPathEp, getRegionPath } from '@/utils/index'
// import { Level } from 'chalk'
// import regionJSON from './region.json'
export default {
name: '',
props: {
value: {
type: [String, Array, Number],
default: ''
},
defLoadKey: {
type: Array,
default: () => []
},
multiple: {
type: Boolean,
default: true
},
checkStrictly: {
type: Boolean,
default: false
},
placeholder: {
type: String,
default: '请输入省市区县'
},
rootId: {
type: [String, Number, Array],
default: undefined
},
onlyShow: {
type: Array,
default: () => []
},
size: {
type: String,
default: 'small'
},
hideCountry: {
type: Boolean,
default: false
},
hideRegion: {
type: Boolean,
default: false
},
showAllCountry: {
type: Boolean,
default: false
},
level: {
type: Number,
default: 3
}
},
data() {
// const { data } = require('./regionLevel2.json')
// debugger
// const dataBuild = data
// console.log(dataBuild)
return {
radio: 2,
valueRegion1: undefined,
allCountry: false,
valueRegionPrev: undefined,
nodesPrev: undefined,
isShow: true,
regionIsShow: true,
regionList: [],
regionListBase: [],
regionListLevet1: [],
isHide: this.hideCountry,
isRegionShow: this.hideRegion,
chooseList: [],
areaList: [],
regionProps: {
multiple: this.multiple,
lazy: false, // 全局没要求加载
checkStrictly: this.checkStrictly,
expandTrigger: 'click',
emitPath: false,
value: 'd',
label: 'h',
children: 'c',
lazyLoad(node, resolve) {}
}
}
},
computed: {
valueRegion: {
get(val) {
return this.value
},
set(val) {
this.$emit('input', val)
}
}
},
created() {
// 因为地区JSON比较大,先屏蔽延迟加载,不然进来有卡顿
// setTimeout(() => {
// this.valueRegionPrev = this.valueRegion
// this.isShow = true
// this.$nextTick(() => {
// if (this.valueRegion) {
// this.initNode(this.valueRegion)
// }
// })
// }, 100)
this.init()
},
beforeUpdate() {
},
methods: {
init() {
// 获取地区
getRegionList(this.level).then(({ data }) => {
let dataBuild = data
if (this.showAllCountry) {
dataBuild = [{
d: '0',
h: '全国',
c: data
}
]
}
// this.regionList = dataBuild
this.regionListBase = dataBuild
// console.log(data, 'data')
const level1 = data.map(item => {
return { d: item.d, h: item.h }
})
level1.map((item) => {
this.$set(item, 'disabled', true)
})
// console.log(level1)
this.regionListLevet1 = level1
if (this.radio === 1) {
this.regionList = this.regionListLevet1
} else {
this.regionList = this.regionListBase
}
})
},
getSelectFullPathName() {
return this.$refs['consigneeRef'].presentText
},
changeHandle() {
if (this.multiple === false && this.allCountry) {
this.$emit('noAllCountry')
this.allCountry = false
}
const checkObj = []
const getCheckedNodes = this.$refs['consigneeRef'].getCheckedNodes()
// debugger
getCheckedNodes.forEach((item) => {
if (this.multiple === false) {
if (item && item.parent) {
item.parent.checked = false
}
}
if (item.parent === null) {
item.data.path = item.data.h
checkObj.push(item.data)
} else if (item.parent.checked === false) {
item.data.path = item.parent.data.h + '-' + item.data.h
checkObj.push(item.data)
}
// console.log(item.data)
})
if (this.allCountry) {
checkObj.unshift({ path: '全国' })
}
this.chooseList = checkObj
this.$emit('update:chooseData', this.chooseList)
},
allCountryChangeHandle(val) {
if (val === 1) {
this.chooseList = []
// this.$refs['consigneeRef'].clearCheckedNodes()
// this.$emit('update:chooseData', this.chooseList)
this.valueRegion = []
this.$nextTick(() => {
this.regionList = this.regionListLevet1
})
// this.regionList = this.regionListLevet1
// this.$set(this.$data, 'regionList', this.regionListLevet1)
// setTimeout(() => {
// }, 3000)
} else if (val === 2) {
this.$nextTick(() => {
// this.regionList = this.regionListLevet1
this.regionList = this.regionListBase
})
}
// return false
// debugger
this.regionIsShow = !val
// console.log('val', val)
if (this.multiple === false) {
this.valueRegion = undefined
// this.value = undefined
if (val === 1) {
this.chooseList = [{ d: 0, path: '全国' }]
this.$emit('isAllCountry')
console.log('this.allCountry is true')
} else {
this.chooseList = []
// this.$emit('noAllCountry');
console.log('this.allCountry is false')
}
// console.log('this.chooseList',this.chooseList);
this.$emit('update:chooseData', this.chooseList)
} else {
this.changeHandle()
}
}
}
}
</script>
<style lang="scss" scoped>
.consref-panel ::v-deep {
.el-cascader-menu__wrap {
height: 292px;
}
}
</style>
更多推荐
所有评论(0)