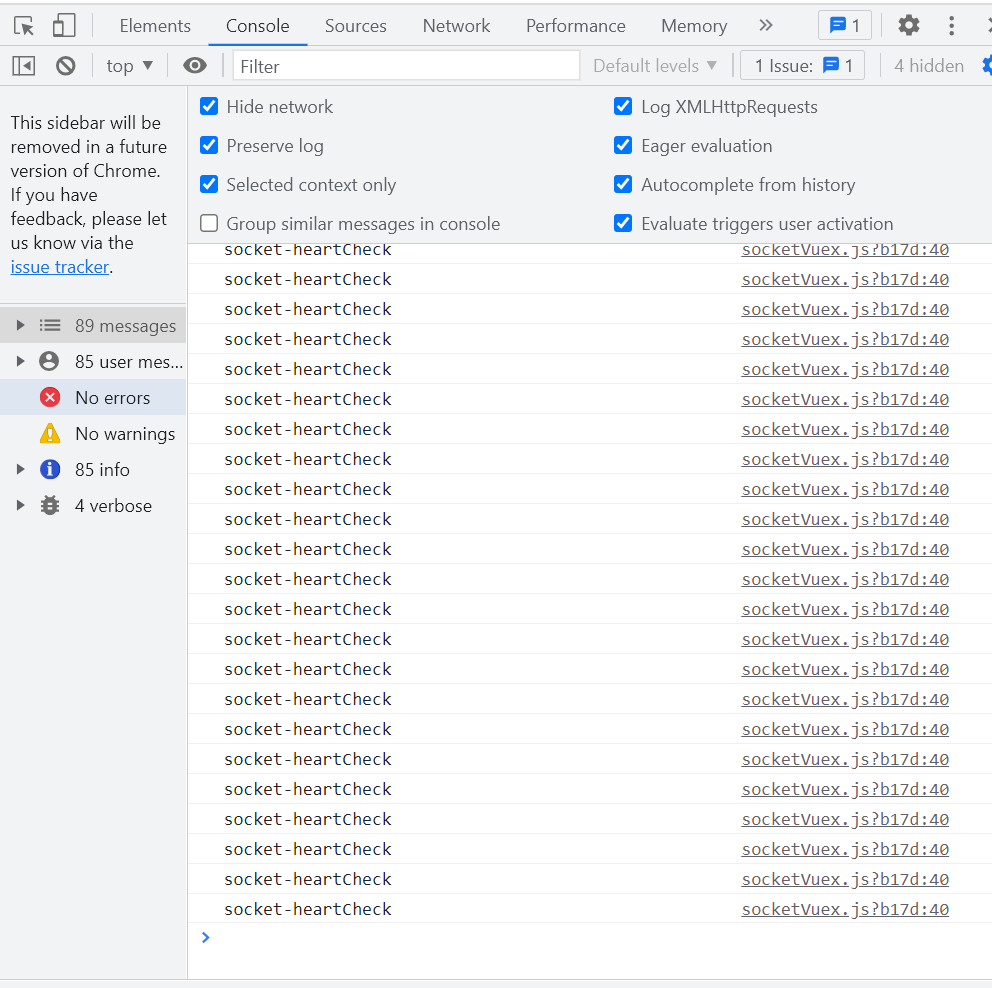
vue使用全局websocket
工具类 socketVuex.js配置文件参数--需要在配置文件中填写#WebSocket地址---https为 wssVUE_APP_WEBSOCKET_URL = 'ws://192.168.31.66:28060/systemInfoSocketServer'工具类jsimport Vue from 'vue'import Vuex from 'vuex'Vue.use(Vuex)expor
·
1.创建工具类
工具类 socketVuex.js
配置文件参数--需要在配置文件中填写
#WebSocket地址---https为 wss
VUE_APP_WEBSOCKET_URL = 'ws://192.168.31.66:28060/systemInfoSocketServer'
工具类js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
ws: null, //建立的连接
lockReconnect: false, //是否真正建立连接
timeout: 15000, //15秒一次心跳
timeoutObj: null, //心跳心跳倒计时
serverTimeoutObj: null, //心跳倒计时
timeoutnum: null, //断开 重连倒计时
msg: null //接收到的信息
},
getters: {
// 获取接收的信息
socketMsgs: state => {
return state.msg
}
},
mutations: {
//初始化ws 用户登录后调用
webSocketInit(state) {
let that = this
//this 创建一个state.ws对象【发送、接收、关闭socket都由这个对象操作】
state.ws = new WebSocket(process.env.VUE_APP_WEBSOCKET_URL);
state.ws.onopen = function(res){
console.log("Connection success...");
/**
* 启动心跳检测
*/
that.commit("start");
}
state.ws.onmessage = function(res){
if (res.data === "heartCheck") {
// 收到服务器信息,心跳重置
that.commit("reset");
console.log("socket-heartCheck");
}else{
state.msg = res;
}
}
state.ws.onclose = function(res){
console.log("Connection closed...");
//重连
that.commit('reconnect');
}
state.ws.onerror = function(res){
console.log("Connection error...");
//重连
that.commit('reconnect');
}
},
reconnect(state) {
//重新连接
let that = this;
if (state.lockReconnect) {
return;
}
state.lockReconnect = true;
//没连接上会一直重连,30秒重试请求重连,设置延迟避免请求过多
state.timeoutnum &&
clearTimeout(state.timeoutnum);
state.timeoutnum = setTimeout(() => {
//新连接
that.commit('webSocketInit')
state.lockReconnect = false;
}, 5000);
},
reset(state) {
//重置心跳
let that = this;
//清除时间
clearTimeout(state.timeoutObj);
clearTimeout(state.serverTimeoutObj);
//重启心跳
that.commit('start')
},
start(state) {
//开启心跳
var self = this;
state.timeoutObj &&
clearTimeout(state.timeoutObj);
state.serverTimeoutObj &&
clearTimeout(state.serverTimeoutObj);
state.timeoutObj = setTimeout(() => {
//这里发送一个心跳,后端收到后,返回一个心跳消息,
if (state.ws.readyState === 1) {
//如果连接正常
state.ws.send("heartCheck");
} else {
//否则重连
self.commit('reconnect');
}
state.serverTimeoutObj = setTimeout(function () {
//超时关闭
state.ws.close();
}, state.timeout);
}, state.timeout);
},
},
actions: {
webSocketInit({
commit
}, url) {
commit('webSocketInit', url)
},
webSocketSend({
commit
}, p) {
commit('webSocketSend', p)
}
}
})
2.引入
在main.js 引入挂载websocket工具
//引入
import socketPublic from '@/utils/socketVuex.js'
//挂载
Vue.prototype.$socketPublic = socketPublic
3.初始化
在 main.vue初始化websocket工具
created () {
this.initWebSocket();
},
methods: {
initWebSocket() {
this.$socketPublic.dispatch('webSocketInit');//初始化ws
},
}
4.使用
在 watch监听方法中接收websocket消息。那个vue页面需要业务消息就在哪里vue页面监听。
watch: {
'socketMsgs': {
//处理接收到的消息
handler: function() {
let that = this
that.toDoSocket(that.socketMsgs.data)
}
}
},
methods: {
toDoSocket: function (data) {
data = JSON.parse(data);
if (data.success) {
}
},
}
总:最后面的使用 根据自己那个vue页面需要获取websocket消息就在那个页面使用就好了。
更多推荐
所有评论(0)