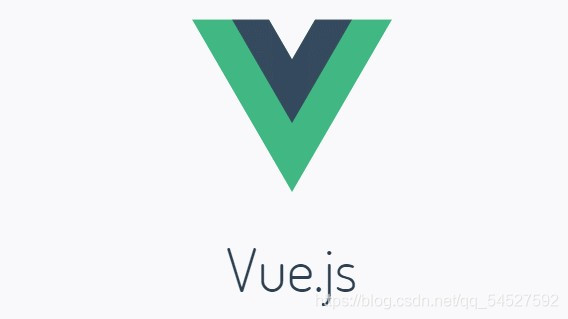
Vuex中四个map方法的使用
1.mapState方法:用于帮助我们映射state中的数据为计算属性2.mapGetters方法:用于帮助我们映射getters中的数据为计算属性<template><div><h1>当前值为: {{sum}}</h1><h2>数值放大{{bigSum}}</h2><List/></div></
·
1.mapState方法:
用于帮助我们映射state
中的数据为计算属性
2.mapGetters方法:
用于帮助我们映射getters
中的数据为计算属性
<template>
<div>
<h1>当前值为: {{sum}}</h1>
<h2>数值放大{{bigSum}}</h2>
<List/>
</div>
</template>
<script>
import List from './components/List.vue'
// 导入mapState, mapGetters方法
import { mapState, mapGetters} from 'vuex'
export default {
name: 'App',
components: {
List
},
computed: {
//借助mapState生成计算属性:sum(对象写法)
// ...mapState({sum:'sum'}),
//借助mapState生成计算属性:sum(数组写法)
...mapState(['sum']),
//借助mapGetters生成计算属性:bigSum(对象写法)
// ...mapGetters({bigSum: 'bigSum'}),
//借助mapGetters生成计算属性:bigSum(数组写法)
...mapGetters(['bigSum'])
}
}
</script>
3.mapActions方法:
用于帮助我们生成与actions
对话的方法,即:包含$store.dispatch(xxx)
的函数
4.mapMutations方法:
用于帮助我们生成与mutations
对话的方法,即:包含$store.commit(xxx)
的函数
<template>
<div>
<select v-model.number="n">
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<button @click="add(n)">点击+</button>
<button @click="awaitAdd(n)">延迟点击+</button>
</div>
</template>
<script>
// mapActions, mapMutations
import { mapActions, mapMutations } from "vuex";
export default {
data() {
return {
n: 1,
};
},
methods: {
//亲自写方法
// add () {
// this.$store.commit('add', this.n)
// },
// awaitAdd() {
// this.$store.dispatch('awaitAdd', this.n)
// }
//借助mapMutations生成对应的方法,方法中会调用commit去联系mutations(对象写法)
// ...mapMutations({add: 'add'}),
//借助mapMutations生成对应的方法,方法中会调用commit去联系mutations(数组写法)
...mapMutations(["add"]),
//借助mapActions生成对应的方法,方法中会调用dispatch去联系actions(对象写法)
// ...mapActions({ awaitAdd: "awaitAdd" }),
//借助mapActions生成对应的方法,方法中会调用dispatch去联系actions(数组写法)
...mapActions(["awaitAdd"]),
},
};
</script>
<style>
</style>
更多推荐
所有评论(0)