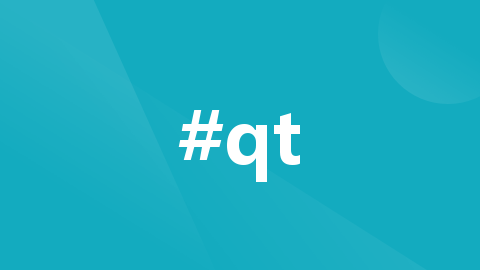
Qt QString 增、删、改、查、格式化等常用方法总结
目录一、QString 格式化 之arg():二、字符串拼接操作三、字符串部分删除四、字符串替换五、字符串查找六、其他,一些常用的方法一、QString 格式化 之arg():1、常规用法QString QString::arg(const QString &a, int fieldWidth = 0, QChar fillChar = QLatin1Char(' ')) constRet

目录
一、QString 格式化 之 arg():
1、常规用法
QString QString::arg(const QString &a, int fieldWidth = 0, QChar fillChar = QLatin1Char(' ')) const;
----------------
Returns a copy of this string with the lowest numbered place marker replaced by string a, i.e., %1, %2, ..., %99.fieldWidth specifies the minimum amount of space that a is padded to and filled with the character fillChar. A positive value produces right-aligned text; a negative value produces left-aligned text.
格式化时的占位符使用: %1, %2, ..., %99,
fieldWidth:指定字符串 a 的宽度,当 | fieldWidth | 的值大于 a 的长度时,以 fillChar 填充。当 fieldWidth 是正值时,右对齐,fillChar 填充在左端;当 fieldWidth 是负值时,左对齐,fillChar 填充在右端。fillChar 缺省时以空格填充。
看个小例子:
qDebug()<<QString("Hello %1!").arg("World"); //"Hello World!"
qDebug()<<QString("Hello %1!").arg("World", 8); //"Hello World!"
qDebug()<<QString("Hello %1!").arg("World", -8); //"Hello World !"
qDebug()<<QString("Hello %1!").arg("World", -8, QChar('*')); //"Hello World***!"
2,qlonglong、qulonglong、long、ulong、int、uint、short、ushort类型的格式化
QString QString::arg(int a, int fieldWidth = 0, int base = 10, QChar fillChar = QLatin1Char(' ')) const;
----------------
The a argument is expressed in base base, which is 10 by default and must be between 2 and 36. For bases other than 10, a is treated as an unsigned integer.
base:指定将 a 转化为字符串时使用的进制,base 必须在 2 和 36 之间,8表示八进制,10表示十进制,16表示十六进制。
看个小例子:
qDebug()<<QString("65535 = %1").arg(65535); //"65535 = 65535"
qDebug()<<QString("65535 = %1").arg(65535,0,2); //"65535 = 1111111111111111"
qDebug()<<QString("65535 = %1").arg(65535,0,16); //"65535 = ffff"
qDebug()<<QString("65535 = %1").arg(65535,10,16); //"65535 = ffff"
qDebug()<<QString("65535 = 0x%1").arg(65535,0,16); //"65535 = 0xffff"
3,double类型的格式化,浮点数
QString QString::arg(double a, int fieldWidth = 0, char format = 'g', int precision = -1, QChar fillChar = QLatin1Char(' ')) const;
----------------
Argument a is formatted according to the specified format and precision. See Argument Formats for details.Format Meaning:格式
e: format as [-]9.9e[+|-]999
E: format as [-]9.9E[+|-]999
f: format as [-]9.9
g: use e or f format, whichever is the most concise
G: use E or f format, whichever is the most concise
precision:精度
看个小例子:
qDebug()<<QString("%1").arg(12.345, 0, 'e'); //"1.234500e+01"
qDebug()<<QString("%1").arg(12.345, 0, 'e', 1); //"1.2e+01"
qDebug()<<QString("%1").arg(12.345, 0, 'E', 2); //"1.23E+01"
qDebug()<<QString("%1").arg(12.345, 0, 'f', 1); //"12.3"
qDebug()<<QString("%1").arg(12.345, 0, 'f', 2); //"12.35"
4,其他
QString QString::arg(const QString &a1, const QString &a2, const QString &a3, const QString &a4, const QString &a5, const QString &a6, const QString &a7, const QString &a8, const QString &a9) const;
----------------
This is the same as calling str.arg(a1).arg(a2).arg(a3).arg(a4).arg(a5).arg(a6).arg(a7).arg(a8).arg(a9), except that the strings a1, a2, a3, a4, a5, a6, a7, a8, and a9 are replaced in one pass.
qDebug()<<QString("%1%2%3%4%5").arg("A","B","C","D","E"); //"ABCDE"
二、字符串拼接操作
str.append(s)方法,将 s 附加到字符串 str 的末尾;
str.prepend(s)方法,将 s 附加到字符串 str 的开头;
str.insert(p, s)方法,在给定的索引位置 p 插入 s;
+ 运算符,str = s1 + s2,将 s1 与 s2 连接在一起,结果赋值给 str;
QString str1 = "Hello ";
QString str2 = "World!";
...
str1.append(str2); //str1:"Hello World!" , str2:"World!"
str2.prepend(str1); //str1:"Hello " , str2:"Hello World!"
str1.insert(str1.size(), str2); //str1:"Hello World!" , str2:"World!"
str1 = str1 + str2; //str1:"Hello World!" , str2:"World!"
三、字符串部分删除
常用方法:str.chop(); str.resize(); str.truncate(); str.remove();
// str.chop(n) 从字符串 str 尾部删除 n 个字符
QString str = "0123456789";
str.chop(2); // str:"01234567"
str.chop(5); // str:"01234"
str.chop(12); // str:""
// str.resize(n) 改变字符串长度为:n
QString str = "0123456789";
str.resize(2); // str:"01"
str.resize(5); // str:"01234"
str.resize(12); // str:"0123456789\u0000\u0000",发生了内存扩充
// str.truncate(n) 从索引 n 处截断字符串
QString str = "0123456789";
str.truncate(2); // str:"01"
str.truncate(5); // str:"01234"
str.truncate(12); // str:"0123456789"
// str.remove(n, m) 从索引 n 处开始删除 m 个字符
QString str = "0123456789";
str.remove(2, 4); // str:"016789"
str.remove(8, 4); // str:"01234567"
str.remove(12, 4); // str:"0123456789"
//QString &QString::remove(QChar ch, Qt::CaseSensitivity cs = Qt::CaseSensitive)
//删除 str 中的所有ch,cs:是否区分大小写
QString str = "0a1A2aa3456789";
str.remove(QChar('a'), Qt::CaseInsensitive); //"0123456789"
四、字符串替换
replace()方法有很多的重载方法,参考官方文档。
QString str = "a0b0,a1b1,A0B0";
str.replace("a0", "--"); //"--b0,a1b1,A0B0"
str.replace("a0", "--", Qt::CaseInsensitive); //"--b0,a1b1,--B0"
str.replace(QRegExp("a[0-9]"), "--"); //"--b0,--b1,A0B0"
// QString &QString::fill(QChar ch, int size = -1)
// Sets every character in the string to character ch. If size is different from -1 (default), the string is resized to size beforehand.
QString str = "12345";
str.fill(QChar('*')); //"*****"
str.fill(QChar('*'), 2); //"**"
五、字符串查找
str.contains(s)方法,如果字符串 str 包含 s,则返回true;否则返回false。
str.count(s)方法,返回 s 在字符串 str 中出现的次数(可能重叠);
str.indexOf(s, from)方法,返回 s 在字符串 str 中第一次出现的索引位置,从索引位置 form 向前搜索。如果没有找到 s,返回 -1;
str.lastIndexOf(s,from)方法,返回 s 在字符串 str 中最后出现的索引位置,从索引位置 form 向后搜索。如果 from 是-1(默认),搜索从最后一个字符开始;如果 from 是-2,在倒数第二个字符,依此类推。如果没有找到 s,返回-1。
str.endsWith(s)方法,如果字符串以 s 结尾,返回 true;否则返回 false。
QString str = "banana";
qDebug()<<str.contains("an"); //true
qDebug()<<str.count("an"); //2
qDebug()<<str.indexOf("an"); //1
qDebug()<<str.indexOf("an", 2); //3
qDebug()<<str.lastIndexOf("an"); //3
qDebug()<<str.lastIndexOf("an", -4); //1
qDebug()<<str.endsWith("an"); //false
六、其他,一些常用的方法
str.left(n)方法,返回一个包含字符串 str 最左边的 n 个字符的子字符串。
str.right(n)方法,返回一个包含字符串 str 最右边的 n 个字符的子字符串。
str.mid(p, n)方法,从指定的位置 p 开始索引,返回一个包含 n 个字符的字符串。
QString str = "banana";
qDebug()<<str.left(2); //"ba"
qDebug()<<str.right(2); //"na"
qDebug()<<str.mid(2); //"nana"
qDebug()<<str.mid(2, 3); //"nan"
更多推荐
所有评论(0)