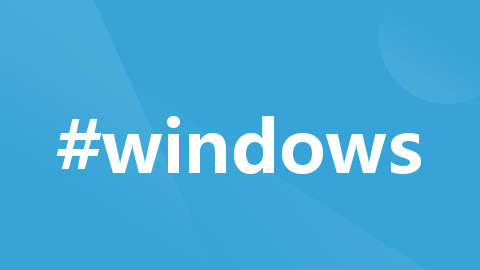
Java8 Stream代码段
import lombok.Data;import org.junit.Test;import java.math.BigDecimal;import java.util.*;import java.util.stream.Collectors;public class Jdk8StreamTest {/*** 分组* 按BuyerName的值,将...
·
import lombok.Data;
import org.junit.Test;
import java.math.BigDecimal;
import java.util.*;
import java.util.stream.Collectors;
public class Jdk8StreamTest {
/**
* 分组
* 按BuyerName的值,将原来的List分为多组
*/
@Test
public void testCollectorsGroupingBy() {
List<Invoice> invoiceList = genInvoiceList();
Map<String, List<Invoice>> map = invoiceList.stream().collect(Collectors.groupingBy(Invoice::getBuyerName));
}
/**
* 分组
*/
@Test
public void testCollectorsGroupingBy2() {
List<User> userList = genUserList();
//按名字分组
Map<String, List<User>> nameMap = userList.stream().collect(Collectors.groupingBy(User::getName));
System.out.println("nameMap = " + nameMap);
//按分数分组
Map<Integer, List<User>> scoreMap = userList.stream().collect(Collectors.groupingBy(User::getScore));
System.out.println("scoreMap = " + scoreMap);
//按名字分组,并求出组内元素个数
Map<String, Long> nameMap2 = userList.stream().collect(Collectors.groupingBy(User::getName, Collectors.counting()));
System.out.println("nameMap2 = " + nameMap2);
//按名字分组,组内的分数加和后取平值值
Map<String, Double> nameMap3 = userList.stream().collect(Collectors.groupingBy(User::getName, Collectors.averagingDouble(User::getScore)));
System.out.println("nameMap3 = " + nameMap3);
//分区:分区是分组的一种特殊情况,分组可以分成多组,但分区只分成2组
//分数及格的人
Map<Boolean, List<User>> map4 = userList.stream().collect(Collectors.partitioningBy(user -> user.getScore() >= 60));
System.out.println("map4 = " + map4);
//按名字分组,同一组内仅取分数最大的那个
Map<String, Optional<User>> map = userList.stream().collect(Collectors.groupingBy(User::getName, Collectors.maxBy(Comparator.comparing(User::getScore))));
map.forEach((k, v) -> {
System.out.println("### k=" + k);
System.out.println("### v=" + v.get());
});
}
/**
* 提取集合中VO的某一属性,如id,生产List<Long>
* 废弃废弃!!!!!!!!!!!!!!!!!!
* 如果id为null将导致idList里存在null元素。
*/
@Test
public void testMap() {
List<User> userList = genUserList();
List<Long> idList = userList.stream().map(User::getId).collect(Collectors.toList());
}
/**
* 提取集合中VO的某一属性,如id,生产List<Long>
* 正确写法
*/
@Test
public void testMap02() {
List<User> userList = genUserList();
List<Long> idList = userList
.stream()
.map(User::getId)
.filter(Objects::nonNull)
.collect(Collectors.toList());
List<String> nameList = userList
.stream()
.map(User::getName)
.filter(StringUtils::isNotBlank)
.collect(Collectors.toList());
}
/**
* 排序
* 按totalAmount的值,将原来的List重新排序,金额小-->大
*/
@Test
public void testSorted() {
List<Invoice> invoiceList = genInvoiceList();
List<Invoice> sortedInvoiceList = invoiceList.stream().sorted(Comparator.comparing(Invoice::getTotalAmount).reversed()).collect(Collectors.toList());
}
/**
* 加和
* 将List里每个对象的totalAmount加和
*/
@Test
public void testMapReduce() {
List<Invoice> invoiceList = genInvoiceList();
BigDecimal sum = invoiceList.stream().map(Invoice::getTotalAmount).reduce(BigDecimal.ZERO, BigDecimal::add);
}
/**
* 过滤
* 过滤出totalAmount大于100的对象
*/
@Test
public void testFilter() {
List<Invoice> invoiceList = genInvoiceList();
List<Invoice> moreThan100List = invoiceList.stream().filter(e -> e.getTotalAmount().compareTo(new BigDecimal("100")) == 1).collect(Collectors.toList());
}
/**
* 过滤
* 过滤出buyerName,剔重,并按字母顺序排序
*/
@Test
public void testFilter2() {
List<Invoice> invoiceList = genInvoiceList();
List<String> buyerNameList = invoiceList.stream().map(Invoice::getBuyerName).distinct().sorted().collect(Collectors.toList());
}
/**
* 发票信息
*/
@Data
private static final class Invoice {
/**
* 购方名称
*/
private String buyerName;
/**
* 价税合计
*/
private BigDecimal totalAmount;
}
@Data
private static final class User {
private Long id;
private String name;
private int score;
private int age;
}
private List<Invoice> genInvoiceList() {
List<Invoice> invoiceList = new ArrayList<>();
Invoice vo1 = new Invoice();
vo1.setBuyerName("A公司-统计");
vo1.setTotalAmount(new BigDecimal("100"));
Invoice vo2 = new Invoice();
vo2.setBuyerName("A公司-统计");
vo2.setTotalAmount(new BigDecimal("200"));
Invoice vo3 = new Invoice();
vo3.setBuyerName("B公司-统计");
vo3.setTotalAmount(new BigDecimal("300"));
Invoice vo4 = new Invoice();
vo4.setBuyerName("B公司-统计");
vo4.setTotalAmount(new BigDecimal("400"));
invoiceList.add(vo4);
invoiceList.add(vo2);
invoiceList.add(vo3);
invoiceList.add(vo1);
return invoiceList;
}
private List<User> genUserList() {
User user1 = new User();
user1.setId(1L);
user1.setName("zhangsan");
user1.setScore(60);
user1.setAge(20);
User user2 = new User();
user2.setId(2L);
user2.setName("lisi");
user2.setScore(80);
user2.setAge(23);
// 重名了:)
User user3 = new User();
user3.setId(3L);
user3.setName("zhangsan");
user3.setScore(80);
user3.setAge(24);
User user4 = new User();
user4.setId(4L);
user4.setName("wangwu");
user4.setScore(50);
user4.setAge(24);
// 重名了:)
User user5 = new User();
user5.setId(5L);
user5.setName("wangwu");
user5.setScore(100);
user5.setAge(22);
List<User> userList = Arrays.asList(user1, user2, user3, user4, user5);
return userList;
}
/**
* List转Map
*/
@Test
public Map<String, String> testList2Map() {
List<GroupJdCompanyVo> list = findAllCompanys();
return list.stream().collect(Collectors.toMap(GroupJdCompanyVo::getCompanyCode, GroupJdCompanyVo::getCompanyName));
}
}
/**
* List -> Map
* 需要注意的是:
* toMap 如果集合对象有重复的key,会报错Duplicate key ....
* apple1,apple12的id都为1。
* 可以用 (k1,k2)->k1 来设置,如果有重复的key,则保留key1,舍弃key2
*/
Map<Integer, Apple> appleMap = appleList.stream().collect(Collectors.toMap(Apple::getId, a -> a,(k1,k2)->k1));
以下是对List去重:
@Getter
@Setter
@ToString
public class ExportTemperatureDto {
private String name;
private Double morningTemperature;
private Double afternoonTemperature;
private String classId;
private String gradeId;
private Integer personId;
}
import static java.util.Comparator.comparing;
import static java.util.stream.Collectors.collectingAndThen;
import static java.util.stream.Collectors.toCollection;
public class StreamTest {
public static void main(String[] args) {
List<ExportTemperatureDto> temperatureList = Lists.newArrayList();
temperatureList.add(new ExportTemperatureDto(1, "haha"));
temperatureList.add(new ExportTemperatureDto(2, "haha"));
temperatureList.add(new ExportTemperatureDto(3, "haha"));
temperatureList.add(new ExportTemperatureDto(4, "haha"));
temperatureList.add(new ExportTemperatureDto(1, "hahaasdas"));
temperatureList.add(new ExportTemperatureDto(2, "hahaasdas"));
List<ExportTemperatureDto> result = temperatureList.stream()
.collect(
collectingAndThen(
toCollection(
() -> new TreeSet<>(comparing(ExportTemperatureDto::getPersonId))
),
ArrayList::new
)
);
result.forEach(System.out::println);
/*
输出结果为:
ExportTemperatureDto(personId=1, name=haha)
ExportTemperatureDto(personId=2, name=haha)
ExportTemperatureDto(personId=3, name=haha)
ExportTemperatureDto(personId=4, name=haha)
因为TreeSet底层是使用TreeMap进行实现的,传入了根据getPersonId进行比较的比较器
在判断personId相同时,其比较结果为0,然后就会替换其value值,而key值是不会变化的,
又因为TreeSet是将传入的元素作为key的,所以使用TreeSet时,当比较器比较的结果相同时,以不会将原来的值替换成比较后的值
*/
}
}
Like查询
List<PersonBase> resultList = allPrimaryList.stream().filter(e -> e.getErpAccount().contains(queryTerm)).collect(Collectors.toList());
求和
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.FutureTask;
import java.util.stream.Collectors;
public class java_list_sum_test {
public static class Person {
int age;
Person (int age) {
this.age = age;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
public static void main(String[] args) throws InterruptedException, ExecutionException {
List<Long> testList = new ArrayList<>(Collections.nCopies(5, 0L));
testList.set(0,1L);
testList.set(1,2L);
testList.set(2,3L);
testList.set(3,4L);
testList.set(4,5L);
System.out.println("sum1 is " + testList.stream().reduce(0L, (a, b) -> a + b));
// reduce根据初始值(参数1)和累积函数(参数2)依次对数据流进行操作,第一个值与初始值送入累积函数,后面计算结果和下一个数据流依次送入累积函数。
System.out.println("sum2 is " + testList.stream().reduce(0L, Long::sum));
System.out.println("sum3 is " + testList.stream().collect(Collectors.summingLong(Long::longValue)));
// Collectors.summingLong()将流中所有元素视为Long类型,并计算所有元素的总和
System.out.println("sum4 is " + testList.stream().mapToLong(Long::longValue).sum());
System.out.println("***********************");
List<Person> testList1 = new ArrayList<>(Collections.nCopies(5, new Person(1)));
System.out.println("class sum1 is " + testList1.stream().map(e -> e.getAge()).reduce(0, (a,b) -> a + b));
System.out.println("class sum2 is " + testList1.stream().map(e -> e.getAge()).reduce(0, Integer::sum));
System.out.println("class sum3 is " + testList1.stream().collect(Collectors.summingInt(Person::getAge)));
System.out.println("class sum4 is " + testList1.stream().map(e -> e.getAge()).mapToInt(Integer::intValue).sum());
return;
}
}
List<Person> persons = Arrays.asList(
new Person("Alice", 25),
new Person("Bob", 30),
new Person("Charlie", 35)
);
int sum = persons.stream()
.mapToInt(Person::getAge)
.sum();
List转Map
//声明一个List集合
List<Person> list = new ArrayList();
list.add(new Person("11", "kiki"));
list.add(new Person("22", "dora"));
list.add(new Person("33", "fred"));
//将list转换map
Map<String, String> map = list
.stream()
.collect(Collectors.toMap(Person::getId, Person::getName));
//输出结果为:
{11=kiki, 22=dora, 33=fred}
对List假分页
List<RecLeaderVo> pageList = resultList.stream()
.skip((pageNoFromOne - 1) * pageSize)
.limit(pageSize)
.collect(Collectors.toList());
Map 过滤
Map<Integer, String> map = new HashMap<>();
map.put(1, "linode.com");
map.put(2, "heroku.com");
//Map -> Stream -> Filter -> String
String result = map.entrySet().stream()
.filter(x -> "something".equals(x.getValue()))
.map(x->x.getValue())
.collect(Collectors.joining());
//Map -> Stream -> Filter -> MAP
Map<Integer, String> collect = map.entrySet().stream()
.filter(x -> x.getKey() == 2)
.collect(Collectors.toMap(x -> x.getKey(), x -> x.getValue()));
// or like this
Map<Integer, String> collect = map.entrySet().stream()
.filter(x -> x.getKey() == 3)
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
google guava
ImmutableMap<Long, Person> map0 = personList.stream().collect(
ImmutableMap.toImmutableMap(Person::getId, java.util.function.Function.identity(), (k1, k2) -> k2));
System.out.println(JSONObject.toJSONString(map0));
更多推荐
所有评论(0)